Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial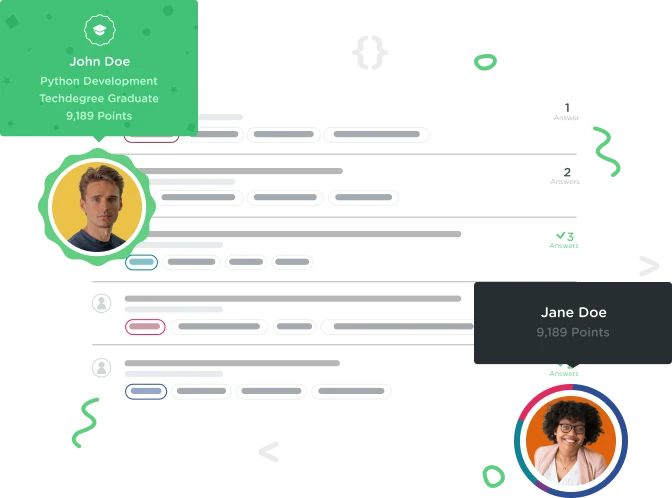
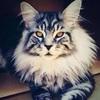
Alex Rodriguez
Front End Web Development Techdegree Student 20,810 PointsNeed help with challenge Task
I cant figure out how to do this task. I keep getting errors. Can some one help me please
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
} //end of ScrabblePlayer
public String getHand() {
return mHand;
}// end of gethand
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}//end of addtile
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}//end of hasTile
public String getTileCount() {
String progress = "";
for (char tile: mHand.toCharArray()) {
char display = '-';
}//end of for loop
if (mHand.indexOf(tile) >= 0) {
display = tile;
}//end of if
}//end of getTileCount
}//end of class
4 Answers
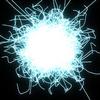
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Alex, it looks like you're trying to do what the video had you do, that's not necessary in this challenge, all that we want to do is check and see if the argument that we pass into the getTileCount() method matches a tile in the player's hand.
So we'd start by declaring the method.
public int getTileCount(char letter) {
}
We'd then want to create a count variable and start our loop that would go through each char in the string mHand, your for loop was correct, you would just want to make sure that you give another char argument as a parameter into your method so that you can compare them.
int count = 0;
for (char tile : mHand.toCharArray()) {
}
So now we got our loop, and our objects to compare together, last thing to do is expand our for loop in increment the counter if the letter that we are checking for is contained in the hand.
if (char == letter) {
count++;
}
Finally, we'd just need to return the count after we are out of the for loop, so altogether the code should look similar too.
public int getTileCount(char letter) {
int count = 0;
for (char tile :mHand.toCharArray()) {
if (tile == letter) {
count++;
}
}
return count;
}
Thanks, let me know if this doesn't help.
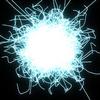
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Emmet,
The reason we want to call greeting is because that is the variable that we declared in the for loop, it's greeting that will be going through each string, so greeting constantly changes, to the next string in the array.
If we went through a for loop and just called our array each time we would have our array printed out four times, with each word that contains of an array.
think of a simple array that counts.
int[] intArray = {1, 2, 3, 4, 5}; for (int myInt: intArray) { System.out.println(myInt); }
would print 1 2 3 4 5
if we do this again and just call our array
int[] intArray = {1, 2, 3, 4, 5}; for (int myInt: intArray) { System.out.println(intArray); }
we'd get
1 2 3 4 5 1 2 3 4 5 1 2 3 4 5 1 2 3 4 5 1 2 3 4 5
because we're essentially telling our program since there are 5 ints in this I want to go through and for every int you come across, print out the whole array.
Thanks, I hope this helps.

Emmet Lowry
10,196 PointsHi rob could you explain the loop a bit more. I am having a tough time learning java any ideas what i should do and does it always have to be (char letter) Thanks.
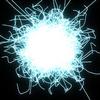
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Emmet,
Just staying with it would be the best bet. It's always trouble in the start but you get used to it and it does get easier.
The for loop is a probably the strongest loop that we have, keep in mind that we can only loop through an Array or a List of any kind, so it doesn't need to be a char at all. though in this instance what that loop is saying it is doing is we're first defining a char variable called letter, we are then looping through each character in mHand and we're checking to see if the value matches up with the letter than. Basicially think of it as us telling java.
"Java, loop through every character in mHand Array, and check that letter, if it matches the letter we are having the method check for, increment our count object by one."
Here's a simpler version we'll first start by creating an array on strings
String[] greetings = {"hi", "hello", "bye", "goodbye"}; for (String currentGreeting : greetings) { System.out.println(currentGreeting); }
What are doing with this?
We're telling java that we are declaring a String currentGreeting and we are wanting it to go through each String in our greetings array. We are then telling it to print each greeting on a new line.
So we should see
hi hello bye goodbye
printed to the screen with this, and we would.
Thanks let me know if this doesn't help.

Emmet Lowry
10,196 PointsOne question on how that loop above works why call the currentGreeting in the system.out.printIn, Could you not just call the greeting array in the first place? Thx for the other advice rob.