Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial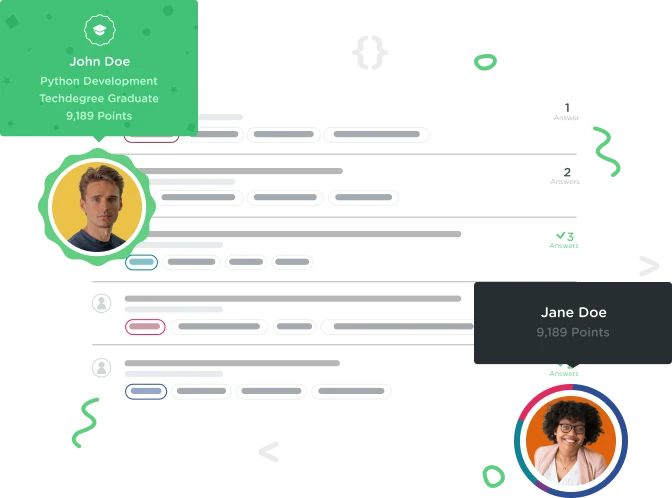

Kabir Gandhiok
12,553 PointsNeed help with a code challenge that asks to remove any vowels from a word that's used to call that function
The challenge is to create a function with a single word parameter, and to remove any vowels that are withing that word from inside the function and then return the word.
I've attached my code with this post.
It returns for instance "h, l, l" if I pass in "hello", I guess it the code isnt passing the challenge because of the comma in the string?
Thanks for your help!
def disemvowel(word):
my_list = list(word)
my_vowels = ["a", "e", "i", "o", "u"]
for letter in my_list:
if letter.lower() in my_vowels:
my_list.remove(letter)
string = ", ".join(my_list)
return string
new_word = "hellO"
disemvowel(new_word)
1 Answer
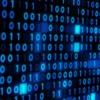
Alexander Davison
65,469 PointsYou don't need to call the function. (The code challenge does that for you)
You also shouldn't join the string with commas, and you don't need to create a list.
You can solve the challenge like this:
def disemvowel(string):
result = ""
for letter in string:
if letter.lower() not in 'aeiou':
result += letter
return result
How it works:
- We set the variable
result
to an empty string so later we can add letters to it. - We iterate though every single letter in the string and set the "temporary" variable's name to
letter
. - If the letter we are on lowercased isn't in 'aeiou' which are all the vowels, then we add the letter to the
result
variable. - Finally, after we loop through all the letters in the string, we return the
result
variable.
I hope this helps. ~Alex
If you have any questions, please ask below
Kabir Gandhiok
12,553 PointsKabir Gandhiok
12,553 PointsThanks Alex! That was awesome, I get it now :)