Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial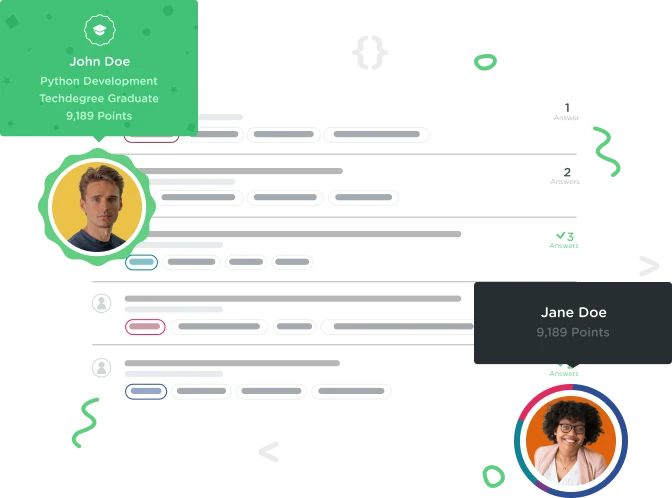

Jacek Skrzypek
5,040 PointsNeed help in removing and attaching buttons after every click
I tried to avoid attaching 'up' button to first li and 'down' to the last by putting an if statement into the attachListItemButtons(li) and then removing and attaching buttons after each click:
function attachListItemButtons(li) {
if (li != firstListItem) {
let up = document.createElement('button');
up.className = 'up';
up.textContent = 'Up';
li.appendChild(up);
};
if (li != lastListItem) {
let down = document.createElement('button');
down.className = 'down';
down.textContent = 'Down';
li.appendChild(down);
};
let remove = document.createElement('button');
remove.className = 'remove';
remove.textContent = 'Remove';
li.appendChild(remove);
}
for (let i = 0; i < lis.length; i ++) {
attachListItemButtons(lis[i]);
}
function removeListItemButtons(li) {
while (li.firstElementChild) {
let button = li.firstElementChild;
li.removeChild(button);
}
};
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
}
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(nextLi, li);
}
}
for (let i = 0; i < lis.length; i ++) {
removeListItemButtons(lis[i]);
}
for (let i = 0; i < lis.length; i ++) {
attachListItemButtons(lis[i]);
}
}
});
But the if statements don't seem to work 'inside' the click, as if inserting would not affect the first(and last)ListItem property?
I guess hiding the buttons would make more sense than removing ant attaching them, but I still would like to know, why my first solution didn't work.
2 Answers

KRIS NIKOLAISEN
54,971 PointsfirstListItem and lastListItem refer to the first and last list items respectively at the time of assignment: in this case when the page loads. By declaring them at the top of the script firstListItem will always be the 'grapes' list item and lastListItem will always be the 'plums' list item regardless of where they are moved up and down the list.
To get the current first and last list items, they should be read inside the attachListItemButtons() function.
function attachListItemButtons(li) {
if (li != listUl.firstElementChild) {
let up = document.createElement('button');
up.className = 'up';
up.textContent = 'Up';
li.appendChild(up);
};
if (li != listUl.lastElementChild) {
let down = document.createElement('button');
down.className = 'down';
down.textContent = 'Down';
li.appendChild(down);
};
let remove = document.createElement('button');
remove.className = 'remove';
remove.textContent = 'Remove';
li.appendChild(remove);
}

Jacek Skrzypek
5,040 PointsThanks Kris!