Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial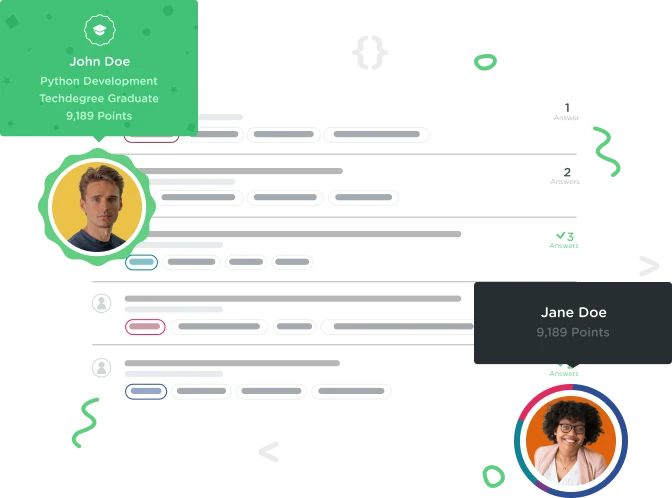
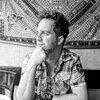
jlampstack
23,932 PointsNeed help in better understanding declaring new variables....
At 1:45 Guil declares a new variable with the following code....
'use strict';
let toybox = { item1: 'car', item2: 'ball', item3: 'frisbee' };
let {item3: train} = toybox;
console.log(train);
Just to clarify, now train = frisbee
?
What is the purpose of this? Why would one want to do this? A train is not a frisbee, so why would someone want two different variable names to hold the same value?
Can anyone provide an example? Thanks!
4 Answers

Seth Kroger
56,415 PointsDestructuring is about pulling pulling an object or array apart into its components. In the example you are creating a variable named train
and assigning it the value of the item3
property of toys. Admittedly the value of 'frisbee' doesn't make much sense as the value of train
but we can rename the variable to something else and it would still give the same result:
let { item3: someToy } = toybox;
// someToy = toybox.item3, or someToy = 'frisbee'
//Or we can just use the property name
let { item3 } = toybox;
// item3 = toybox.item3 = 'frisbee'
// We can do more than one property at once.
let { item1, item2 } = toybox;
// item1 = toybox.item1 and item2 = toybox.item2
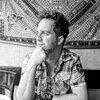
jlampstack
23,932 PointsTO RECAP: I was getting a bit crossed up with the whole frisbee / disc. I was unaware at first that disc is another name for frisbee. I would have better understood with a different example. I've altered the scenario in case anyone else is has the same problem understanding as me. Hope this helps....
'use strict';
let toybox = { item1: 'car', item2: 'ball', item3: 'frisbee' }; // Creates new toybox object holding 3 properties / key-value pairs
let {item3: frisbee} = toybox; // Extracts item3 from toy box and creates a new variable named frisbee
// The new variable doesn't need to be named frisbee
// We can name it anything we like, but frisbee just makes sense
frisbee = "flying disc"; // Assigns new value to frisbee changing value from 'frisbee' to 'flying disc'
console.log(frisbee); // consoles value of new frisbee variable
console.log(frisbee.toLocaleUpperCase()); // just for fun to show we can use it same way as any regular variable
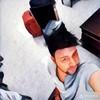
Ari Misha
19,323 PointsHiya there! Destructuring syntax in ES6 is one of the most important features ever in JavaScript Ecosystem. Complex frameworks like Angular or NativeScript or React etc., are built on it. In the above example, you're destructuring the toybox variable which contains an object literal. See the thing is it's not 'bout the meaning of words in English since its a computer programming language, it's defo 'bout understanding the syntax. I'll give ya a couple of examples that you'll encounter a lot in JS:
- Example 1:
// app/time.js
export class Time { ... }
export class Minutes extends Time { ... }
export class Seconds extends minutes { ... }
// app/app.js
import { Time } from './time.js';
...
...
- Example 2:
let cart = { name: "John Doe", item: "Jeans", quantity: "2", price: "30.99$" }
let { brand } = cart;
console.log (brand) // logs out "undefined"
~ Ari
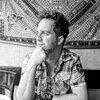
jlampstack
23,932 PointsThanks Ari Misha - I understand the syntax, but I don't fully understand the examples. Perhaps its a bit more advanced than my level. I will probably need to build my skills up to that. I'm not familiar with any of the frameworks you mentioned.
jlampstack
23,932 Pointsjlampstack
23,932 PointsThank you Seth Kroger . With your help I think i had an AHA! Moment, but not quite 100% confident. Just to recap....
let { property:variable } = object