Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial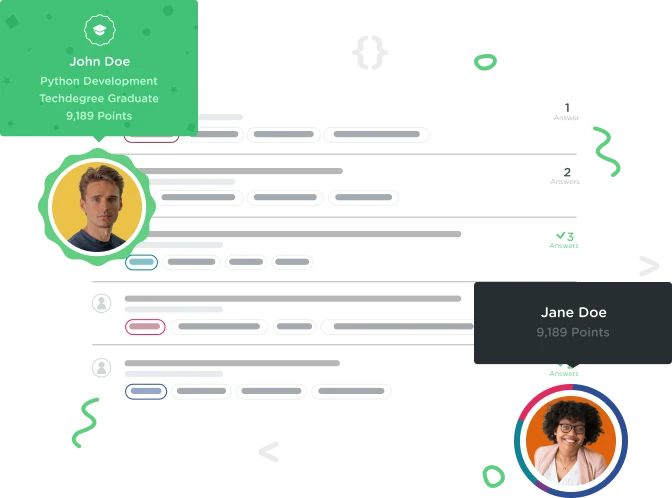
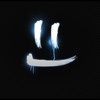
Grigorij Schleifer
10,365 PointsNeed help
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "This ist a String or a BlogPost";
BlogPost myBlogPost;
if(result instanceof Object){
result = (String) obj;
return result;
}else if(BlogPost instanceof Object){
myBlogPost = (BlogPost) obj;
return myBlogPost.getTitle();
}
return "";
}
}
The error says that i didnt initialised the BlogPost? What i am doing wrong?
2 Answers
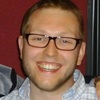
Victor Learned
6,976 PointsYour if statement is attempting to use the variable before it's been given a value or initialized. You only declared a BlogPost variable. You never actually initialized. Before a variable can be used it must be given an initial value. This is called initializing the variable. If we try to use a variable without first giving it a value:
Example:
int numberOfDays; //try and add 10 to the value of numberOfDays
numberOfDays = numberOfDays + 10;
the compiler will throw an error
You actually don't even need to create a variable to since what you really want is just the title. So you can just cast the obj to a BlogPost and immediately call the getTitle method which returns the string you want:
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if(result instanceof Object){
result = (String) obj;
}else if(BlogPost instanceof Object){
result = ((BlogPost) obj).getTitle();
}
return result;
}
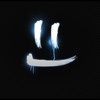
Grigorij Schleifer
10,365 PointsI added
BlogPost myBlogPost = new BlogPost();
And i get this error :
./TypeCastChecker.java:15: error: constructor BlogPost in class BlogPost cannot be applied to given types; BlogPost blogPost = new BlogPost(); ^ required: String,String,String,String,Date found: no arguments reason: actual and formal argument lists differ in length 1 error
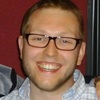
Victor Learned
6,976 PointsThat was my fault for not actually looking at the code before suggesting an answer. I've updated my answer and I have tested to ensure it's correct.