Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial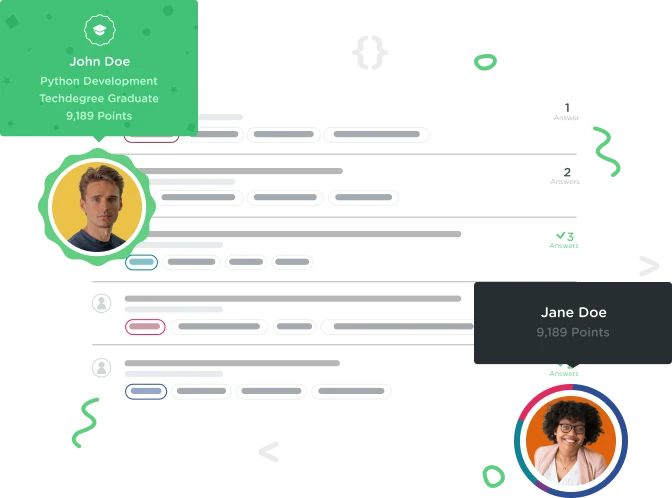

Daniel Coulter
4,691 PointsMy solution for the Refactor Challenge
let html = ''; const color = () => { return Math.floor(Math.random() * 256);} let randomRGB;
for ( let i = 1; i < 11; i++) {
randomRGB = rgb( ${color()}, ${color()}, ${color()} )
;
html += <div style="background-color: ${randomRGB}">${i}</div>
;
}
document.querySelector('main').innerHTML = html;
1 Answer
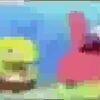
Charlie Palmer
15,445 Pointsconst eightBit = () => Math.floor(Math.random() * 256);
const genRGB = () => `rgb(${eightBit()}, ${eightBit()}, ${eightBit()})`;
const genHTML = (length) => {
let html = '';
for(let i = 0; i > length; i++) {
html += `<div style="background-color: ${genRGB()}">${i}</div>`
}
return html;
}
document.querySelector('main').innerHTML = genHTML(10);
haven't actually tested to see if it works but probably should. with arrow functions, you can skip the curly braces if the function can make a return in one line, you also won't have to use the return keyword as it should return the value returned from the method. I'm pretty sure instead of using the for-loop you could use a syntax like this
//Array(length).forEach((element, index) => {your function});
Array(10).forEach((x, I) => {
html += `<div style="background-color: ${genRGB()}">${i}</div>`;
});
however, I'm pretty sure that is bad practice unless you're trying to create and fill an array with data, the syntax might be a little bit wrong too but I don't think so.
so it could look like this:
const eightBit = () => Math.floor(Math.random() * 256);
const genRGB = () => `rgb(${eightBit()}, ${eightBit()}, ${eightBit()})`;
const genHTML = (length) => {
let html = '';
Array(length).forEach((x, i) => {
html += `<div style="background-color: ${genRGB()}">${i}</div>`;
});
return html;
}
document.querySelector('main').innerHTML = genHTML(10);
I'm sure Steven Parker will correct me if I'm wrong :)
Steven Parker
231,269 PointsSteven Parker
231,269 PointsOnly because you invoked me.
Array(n).fill(0).forEach()
would)And while it can work, creating an otherwise unused array simply to use forEach instead of for is pretty bizarre and less efficient (17% slower according to JSBench.me).
And did Daniel actually ask a question? I thought he might just be sharing.