Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial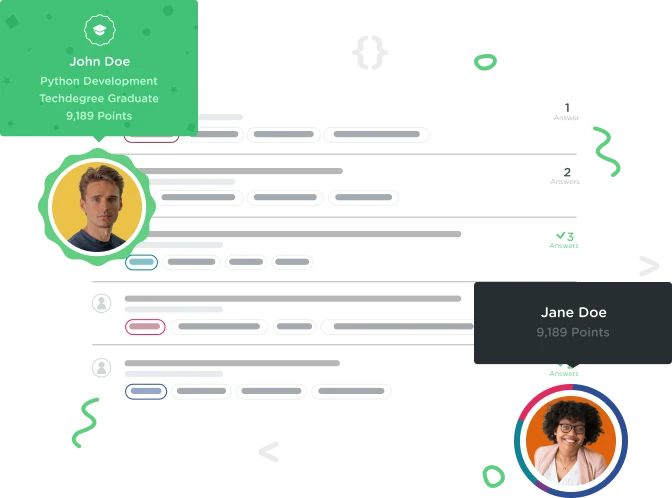

Mamta Chaudhary
527 PointsMy program is not letting the user enter their signature, the program ends before the signature is entered.
package Motel; import java.util.Scanner; import java.util.Date;
class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Scanner console = new Scanner(System.in);
Guest Identification = new Guest();
//Identification.Guest();
Date date = new Date();
System.out.println("Welcome to the Motel");
System.out.println("Please answer the following Questions to Check In: ");
System.out.println("Enter your Full Name: ");
String name = input.nextLine();
System.out.println("Enter your Address: ");
String address = input.nextLine();
System.out.println("Date of Birth: " + date.toString());
String dateOfBirth = input.nextLine();
System.out.println("Enter your Identification Card Number or SSC number: ");
int identificationNumber = input.nextInt();
System.out.println("Enter your Full Name for a E-Signature: ");
String signature = input.nextLine();
System.out.println(signature);
if (signature.equals(name)) {
System.out.println("Please Make sure this information is Correct: ");
System.out.printf(" Name: %s %n", Identification.getGuestName());
System.out.printf(" Address: %s %n", Identification.getGuestAddress());
System.out.printf(" D.O.B: %d %n", Identification.getGuestDateOfBirth());
System.out.printf(" ID#: %d %n", Identification.getGuestIdentificationNumber());
System.out.printf(" E Signature: ", Identification.getGuestSignature());
}
}
}
package Motel; import java.io.Serializable; public class Guest implements Serializable {
private String guestName;
private String guestAddress;
private String guestSignature;
private int guestDateOfBirth;
private int guestIdentificationNumber;
public Guest() {
this.guestName = guestName;
this.guestAddress = guestAddress;
this.guestDateOfBirth = guestDateOfBirth;
this.guestIdentificationNumber = guestIdentificationNumber;
this.guestSignature = guestSignature;
}
public Guest (String name, String address, String signature,
int dateOfBirth, int identificationNumber) {
this.guestName = name;
this.guestAddress = address;
this.guestDateOfBirth = dateOfBirth;
this.guestIdentificationNumber = identificationNumber;
this.guestSignature = signature;
}
public String getGuestName () {
return guestName;
}
public void setGuestName (String name){
this.guestName = name;
}
public String getGuestAddress () {
return guestAddress;
}
public void setGuestAddress (String address){
this.guestAddress = address;
}
public String getGuestSignature () {
return guestSignature;
}
public void setGuestSignature (String signature){
this.guestSignature = signature;
}
public int getGuestDateOfBirth () {
return guestDateOfBirth;
}
public void setGuestDateOfBirth ( int dateOfBirth){
this.guestDateOfBirth = dateOfBirth;
}
public int getGuestIdentificationNumber () {
return guestIdentificationNumber;
}
public void setGuestIdentificationNumber ( int iD){
this.guestIdentificationNumber = iD;
}
}
1 Answer
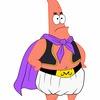
<noob />
17,062 Pointsfirst of all u have 2 constructors called Guest. u can overload constructs but their params have to be with different values and here u have the same constructor. i think you have problems with the date object as well but iβm not yet familiar with the date object so i canβt tell whatβs wrong