Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial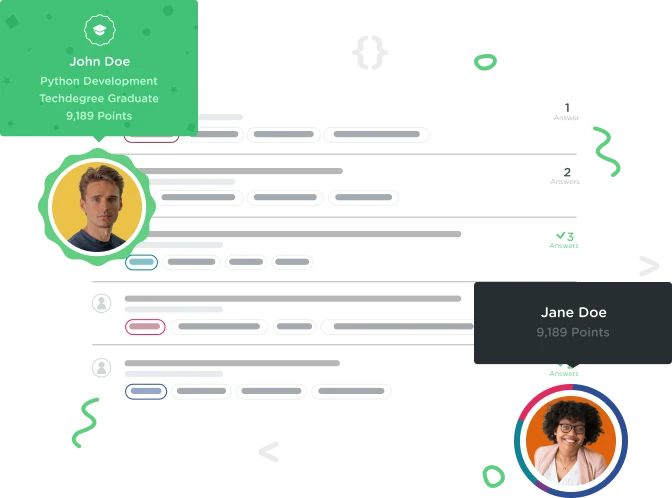

Wan Syarmin
340 PointsMy Output did not display
import java.io.Console;
public class Introductions {
public static void main(String[] args) {
Console console = System.console();
// Welcome to the Introductions program! Your code goes below here
console.printf("Hello, my name is Wan");
} }
I write the code as instructed from the video, but when i compile the text, the text did not display
treehouse:~/workspace$ javac Introductions.java
treehouse:~/workspace$ ls
Introductions.class Introductions.java
treehouse:~/workspace$ java Introductions
treehouse:~/workspace$
What i did wrong?, i am confuse.The "Hello, my name is Wan" didn't display
5 Answers

Derek Markman
16,291 PointsI'm glad you got it to work. :) Btw if my answers were helpful to you, if you wouldn't mind marking one as 'best answer' that would be highly appreciated. Also, you were close with your conditional statements, your only syntax error was after your boolean expressions, in your if statements. Remove the semi-colons after the if portion in your in your if - else statements.
import java.io.Console;
public class Introductions {
public static void main(String[] args) {
Console console = System.console();
String myName = console.readLine("what is your name ?");
if (myName.equals("Dog")) //you had a semi-colon here
{
console.printf("Go to hell\n");
System.exit(0);
}
String age = console.readLine("How old are you btw?");
int old = Integer.parseInt(age);
if (old<13) //you also had a semi-colon here
{
console.printf("Dude get lost,you are under age\n");
System.exit(0);
}
// Welcome to the Introductions program! Your code goes below here
console.printf("hi %s nice to meet you im window\n",myName);
console.printf("Finally %s you finally get the compiler working\n",myName);
console.printf("Good for you %s \n",myName);
}
}

Derek Markman
16,291 PointsYour code looks fine to me, I opened a workspace and it worked perfectly. Maybe try compiling and running it on one line:
javac Introductions.java && java Introductions

Wan Syarmin
340 Pointsty for your reply, i try your method but still not working..maybe its the workspace prob..im using it in chrome

Derek Markman
16,291 PointsMake sure you java file name is with a capital "I". And when you compile and run make sure you use a Capital "I"
I'm using chrome as well, try copy/paste my code and see if it works:
import java.io.Console;
public class Introductions {
public static void main(String[] args) {
Console console = System.console();
// Welcome to the Introductions program! Your code goes below here
console.printf("Hello, my name is Wan %n");
}
}
And compile and run on one line like before.

Wan Syarmin
340 Pointsi did what you ask and still the same result, i think it the compiler problem, cause my coding is correct, i appreciate your help Derek Markman

Derek Markman
16,291 PointsI don't think its the compilers fault seeing as how I just opened a new one up and compiled/ran my code fine, is your java file name with a capital "i"? Or a lowercase? Your java file name Introductions.java need a capital "i". Also make sure that your class name in your actual code is spelled with a capital "i".
If you used my code, I used a %n new line in my print statement, this is what your console should look like if compiled and ran properly:
treehouse:~/workspace$ javac Introductions.java
treehouse:~/workspace$ java Introductions
Hello, my name is Wan
treehouse:~/workspace$
Also, after you compiled you should've got a .class file auto generated, did you get an Introductions.class file? Take a snapshot of your workspace (It's the top-right corner inside your workspace, it's a square with a dot in the middle icon) After you snapshot your workspace send me the link to it.

Wan Syarmin
340 Pointsnow u mention it Derek, i check there is no Introduction.class file, when i click it, it says "No preview available"
btw this the snapshot link https://w.trhou.se/pafdct8rwq

Derek Markman
16,291 PointsOn your snapshot I can see that you did get an Introductions.class file. Which means that your code compiled successfully. What I want you to do is delete the class file, and the test java file and then just re compile your code:
javac Introductions.java
after you compile, take another snapshot and let me see it. And you shouldn't be able to preview your class file, there is no need to anyways so don't worry that's normal.

Wan Syarmin
340 Pointsty Derek its working now,i have another question i want to ask you,
https://w.trhou.se/0l1ef1mdow this is the link
its about the if statement, in the if statement those lower than 13 will get a text that i provide,and exit the program but if input more it will resume the system, my problem is when i input higher the 13 it will still get that text..why?
sorry for my bad english
Wan Syarmin
340 PointsWan Syarmin
340 PointsTy bro u are awesome :D
Derek Markman
16,291 PointsDerek Markman
16,291 PointsNo problem. :) Let me know if you have any other questions.
Wan Syarmin
340 PointsWan Syarmin
340 Pointssorry to bother you again bro but i need your help
https://w.trhou.se/zmi3jra31s
my do while keep repeating my text without giving me the chance to re input it back, why?
Derek Markman
16,291 PointsDerek Markman
16,291 PointsWatch out for infinite loops. You created two by assigning the readLine method to your name string variable and old string variable outside of your 'do code-block'. Let me know if you want me to clarify about how, assigning the readLine method to your string variables, outside of your 'do code-block', creates an infinite loop.
All you need to do is assign your string variables, name and old, inside of your 'do code-block' as the first line of code, so if your boolean-expressions from the if-statements as well as the 'while boolean-expression' come back as true it will always invoke the readLine method first.
I refactored your code just a little bit, but it seems to work as expected.