Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial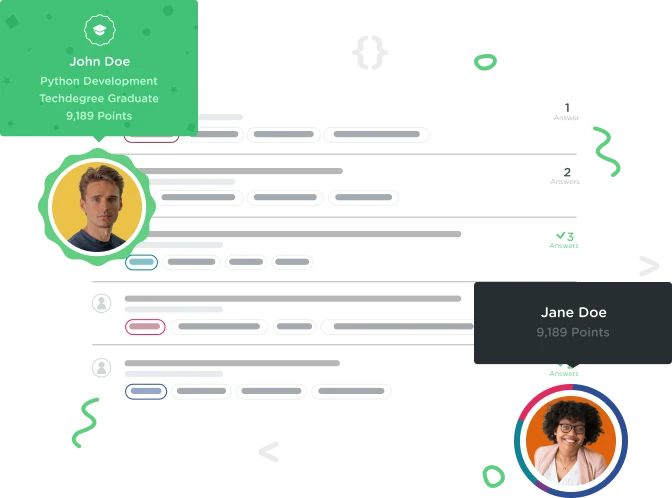

Diana Rooks
25,134 PointsMy froggy doesn't jump
When I press play to preview the game, the frog starts its idling dance. However, it does not jump when I press any of the following keys: up arrow, down arrow, right arrow, left arrow, w, a, s, or d.
Here is my code:
using UnityEngine;
using System.Collections;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
if (movement != Vector3.zero) {
playerAnimator.SetFloat ("Speed", 3f);
} else {
playerAnimator.SetFloat ("Speed", 0f);
}
}
}
2 Answers
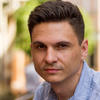
Nick Pettit
Treehouse TeacherAt this point in the project's development, the frog should only hop forward when you press any of the movement keys; and it will hop right off the screen.
I copy and pasted this code into my copy of the game and it behaved just as I described. My guess is that the error is somewhere else, either in a script, or in Unity's compilation.
Some things to try:
- Check the Console window, which is the tab right next to the Project window. Do you see any errors? If so, you'll need to fix them, otherwise Unity won't update your game with changes to any of your other scripts.
- Try restarting Unity. Sometimes this can fix strange issues with compilation on some hardware / OS configurations.
I hope that helps! If you're still having trouble, let me know and we'll figure it out.

Diana Rooks
25,134 PointsThanks, Nick. It's encouraging to know that I entered the code correctly, if only because I proofread it no less than 10 times and was starting to go a little cross-eyed. The problem, it turns out, was in the player object inspector. The PlayerMovement script, although created from this inspector, was not showing up as a component. After I reattached it, the frog could move forward and, mercifully, so could I.
P.S. Really cool course so far!
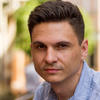
Nick Pettit
Treehouse TeacherThat makes sense! Glad you figured it out.
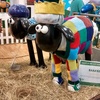
Bridie Begbie
785 PointsDiana, Id be really grateful if you could talk me through reattaching the playermovement script. i think I have the same problem as you!
Many thanks!
Jared Armes
Courses Plus Student 6,391 PointsJared Armes
Courses Plus Student 6,391 PointsWow, I had this exact same problem too. I look forward to an answer.