Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial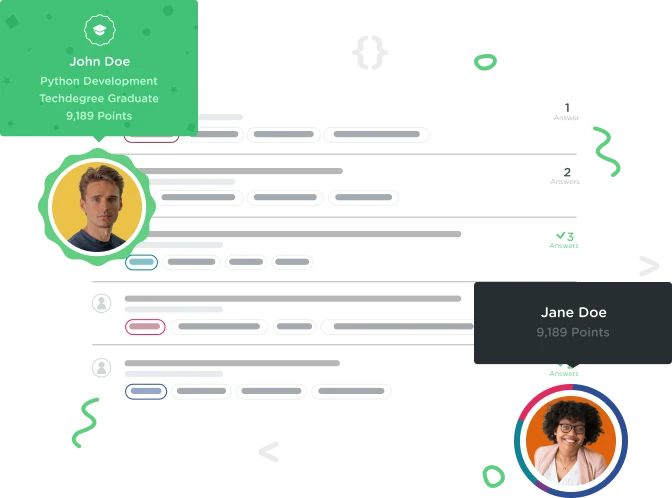

William Conley
Courses Plus Student 6,939 PointsMy code test out fine but I get an eror when I check it after it has been pasted.
package com.teamtreehouse;
import java.io.; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.;
public class Prompter { private BufferedReader mReader; private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<>();
String word = "";
for (String phrase : tmpl.getPlaceHolders()) {
if(!mCensoredWords.contains(word.toLowerCase()))
{
word = promptForWord(phrase);
words.add(word);
}
}
return words;
}
public String promptForWord(String phrase) throws IOException {
System.out.printf("Enter a %s %n%n", phrase);
String response = mReader.readLine();
while(mCensoredWords.contains(response.toLowerCase()) || response == "")
{
System.out.printf("%s is a censored word. Enter a valid %s %n%n", response, phrase);
response = mReader.readLine();
}
return response;
}
public String promptForStory() throws IOException {
System.out.println("Enter a story");
String story = mReader.readLine();
return story;
}
}
package com.teamtreehouse;
import java.io.IOException;
public class Main
{
public static void main(String[] args)
{
Prompter prompter = new Prompter();
try
{
String newStory = prompter.promptForStory();
Template template = new Template(newStory);
prompter.run(template );
}
catch (IOException e)
{
System.out.println("There was a problem running the template");
e.printStackTrace();
}
}
}
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.*;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<>();
String word = "";
for (String phrase : tmpl.getPlaceHolders()) {
if(!mCensoredWords.contains(word.toLowerCase()))
{
word = promptForWord(phrase);
words.add(word);
}
}
return words;
}
public String promptForWord(String phrase) throws IOException {
System.out.printf("Enter a %s %n%n", phrase);
String response = mReader.readLine();
while(mCensoredWords.contains(response.toLowerCase()) || response == "")
{
System.out.printf("%s is a censored word. Enter a valid %s %n%n", response, phrase);
response = mReader.readLine();
}
return response;
}
public String promptForStory() throws IOException {
System.out.println("Enter a story");
String story = mReader.readLine();
return story;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story

William Conley
Courses Plus Student 6,939 PointsThat a censored word was allowed through.

William Conley
Courses Plus Student 6,939 PointsThis problem is for the last challenge in the Java track.
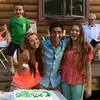
David Axelrod
36,073 PointsIn my opinion this is the hardest challenge on treehouse so props for getting this far! I stored the code locally on my laptop so ill paste in what i used below
1 Answer
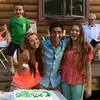
David Axelrod
36,073 PointsMain.java
package com.teamtreehouse;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
Prompter prompt = new Prompter();
Template tmpl = new Template(prompt.getUserStory());
prompt.run(tmpl);
}
}
Prompter.java
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.*;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
/*
ok we have their story and it was passed into to template
prompting,
DONE censoring and
outputting. for outputting use, tmpl (the template we are passing in)
*/
System.out.println("Please put in the words you want to use %n");
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
//we now have to render which takes in the results
String finalStory = tmpl.render(results);
// now remember rendering the template replaces the place holder words (__verb__)
// with the actual things (the words arrayList that is returned when we get the words
// instead of fake words, we have an array list of words from the user
// List<String> fakeResults = Arrays.asList("friend", "talented", "java programmer", "high five");
// String results = tmpl.render(fakeResults);
System.out.printf("Your TreeStory:%n%n%s", finalStory);
}
//prompt the user for the template here. no other choice really......as of right now
public String getUserStory() {
String userStory = null;
try {
System.out.println("Yo please put a story in here. Denominate words to be replaced with 2 underscores on either side");
System.out.println("Example __noun__ or __Adjective__%n");
userStory = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return userStory;
//replace this with user input
//String story = "Thanks __name__ for helping me out. You are really a __adjective__ __noun__ and I owe you a __noun__.";
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
//place holders is a list for stuff like __name__ and __example__
for (String phrase : tmpl.getPlaceHolders()) {
//for each of the placeholders (5 there are 5 iterations)
String word = promptForWord(phrase);
words.add(word);
}
//words is an array list of input , user or otherwise
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) {
String userResponse = null;
do {System.out.printf("Enter a word that is a(n) %s: ", phrase);
try {
userResponse = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}} while (mCensoredWords.contains(userResponse));
// ^^^^^^^^^^while user input is not contained in censored words
//great! we now have the censored word! lets return a string
return userResponse;
// return what was read on the line
//ok this looks good
}
}

William Conley
Courses Plus Student 6,939 PointsThanks for your help. I will compare your feedback to what I have to understand what I did wrong. One thing that stands out is that you used a do while loop and I used a while loop. I'm not real sure what the difference is between implementing a do/while versus just a while loop?
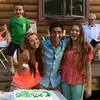
David Axelrod
36,073 PointsA while loop always checks the condition before you run the actual code. a do while runs the code at least once. once the first run is done in the do while, THEN it checks the conditional to see if it needs to run again. Hope this helps!
David Axelrod
36,073 PointsDavid Axelrod
36,073 PointsHey Will! What error did you get?