Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial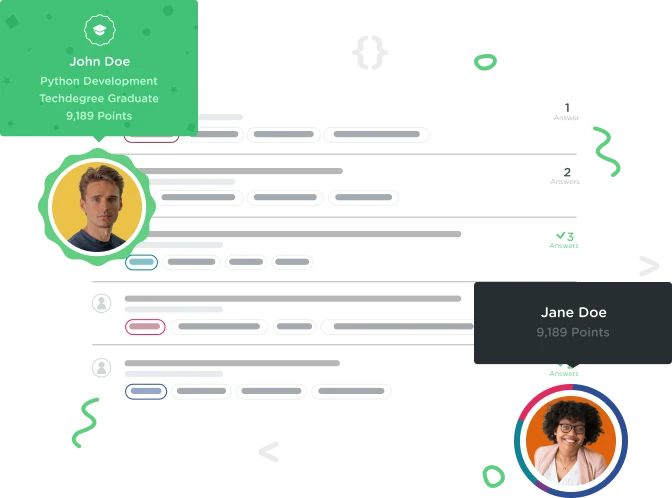

Kieran Forbes
3,706 PointsMy code stops to work when I add the <0 value error
Any help would be appreciated on how I will be able to add a Value Error when the price is less than 0. Thanks!
total = []
prices = []
print("Hi there, and welcome to our store, please enter 5 items you bought today along with their prices")
while len(prices) < 5:
if prices <=0:
raise ValueError("This is a negative value")
try:
purchase = input("What did you buy today?")
price = float(input("How much did it cost?"))
total.append(purchase)
prices.append(price)
except ValueError:
print("Please enter a valid number")
def bubbleSort(nlist):
sorted = False
while not sorted:
swaps = 0
for i in range(len(nlist) -1):
if nlist[i] > nlist[i+1]:
temp1 = nlist[i]
nlist[i] = nlist[i + 1]
nlist[i + 1] = temp1
swaps = swaps + 1
if swaps == 0:
sorted = True
return nlist
bubbleSort(prices)
first_total = (total[0], prices[0])
final_total = list(zip(total, prices))
print(final_total)
discount = first_total
print("Since you bought", len(total), "products with us today, we will now give you the cheapest product for free, meaning you will get", discount, "for free")
totaltotal = prices[1:]
totaltotalt = sum(totaltotal)
print("So the total price that you will pay today is ",totaltotalt)
2 Answers

KRIS NIKOLAISEN
54,970 PointsYou could move it to the try and check the price (not prices)
while len(prices) < 5:
try:
purchase = input("What did you buy today?")
price = float(input("How much did it cost?"))
if price <=0:
raise ValueError("This is a negative value")
total.append(purchase)
prices.append(price)
except ValueError:
print("Please enter a valid number")
Also if you sort prices
without making the same changes to total
the price no longer corresponds to the correct purchase by index

YZ L
6,184 Pointsmake a 'for' loop:
for _ in prices:
if _ <= 0:
raise ValueError("This is a negative value")

Kieran Forbes
3,706 PointsThank you for your reply, can I ask how you would implement that in my code as I am still getting an error?
Kieran Forbes
3,706 PointsKieran Forbes
3,706 PointsGreat solution, thanks