Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial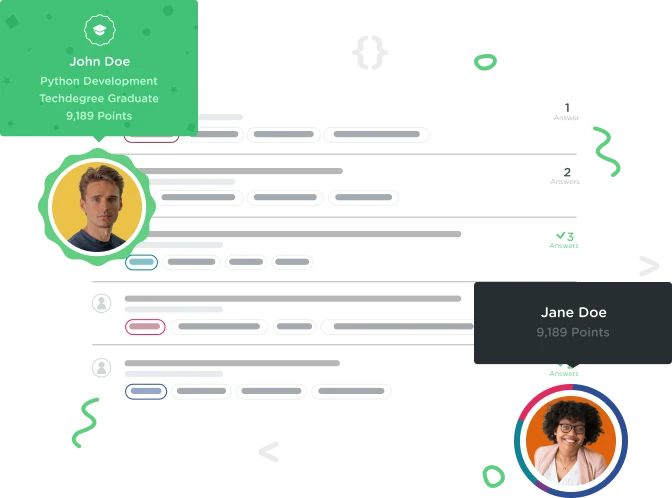
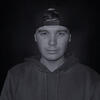
Jacob Larby
2,411 PointsMy Answer for this Unit
let html = '';
// Random number between 1 and 256 to get RGB value.
const RGB = () => Math.floor(Math.random() * 256);
// Loop that loops i amount of times. (Will place corresponding element i amount of times.)
for (let i = 1; i <= 10; i++) {
html += `<div style="background-color: rgb(${RGB()}, ${RGB()}, ${RGB()})">${i}</div>`;
}
// Selects the correct element and places the HTML inside the element.
document.querySelector('main').innerHTML = html;
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">1</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">2</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">3</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">4</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">5</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">6</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">7</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">8</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">9</div>`;
//
//red = Math.floor(Math.random() * 256);
//green = Math.floor(Math.random() * 256);
//blue = Math.floor(Math.random() * 256);
//randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
//html += `<div style="background-color: ${randomRGB}">10</div>`;
}
Let me know what you guys think. Cheers :)
1 Answer
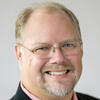
Jason Larson
8,362 PointsI'm not sure what unit you're working on, or if there is a question in your post, but that code looks like a good replacement for the commented code. The only thing I wanted to note is that your first comment is not fully correct.
const RGB = () => Math.floor(Math.random() * 256);
generates a number between 0 and 255 (which is appropriate for an RGB value), rather than generating a number between 1 and 256. I know it's a minor thing, but that small difference can cause some programs to crash, and produce bugs that are difficult to troubleshoot if you're not aware of it. If you actually wanted to generate the values 1 to 256, you would need to write:
const RGB = () => Math.floor((Math.random() * 256) + 1);
The parentheses around Math.random() * 256
are not necessary, but make the code easier to read, imho.