Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial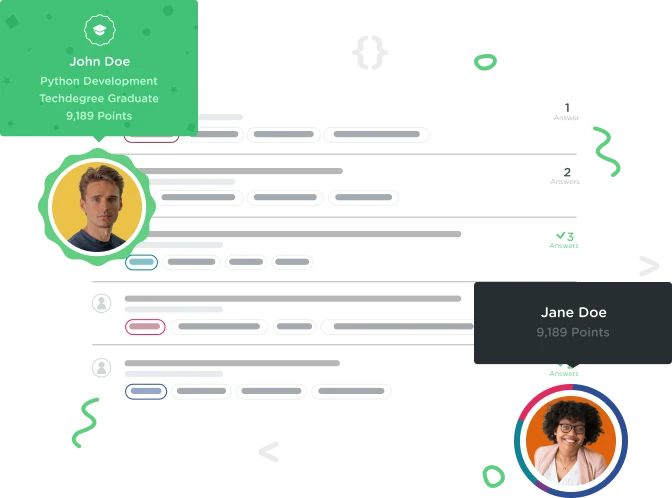

paulscanlon
Courses Plus Student 26,735 PointsMy answer
Hi guys
My way of doing this is slightly more compact than Dave's. Better or worse? What do you think?
Thanks in advance for the feedback
Happy coding
Paul
const products= [
{
name: "chair",
inventory: 2,
unit_price: 29.99 // 59.98
},
{
name: "table",
inventory: 4,
unit_price: 119.99 //479.96
},
{
name: "sofa",
inventory: 4,
unit_price: 699.99 //2799.96 TOTAL 3,339.90
},
];
function listProducts(arr) {
let prodArray = [];
let prodTotal = 0;
for(let i = 0; i < products.length; i++) {
prodArray.push(products[i].name);
prodTotal += products[i].unit_price * products[i].inventory;
}
console.log(prodArray);
console.log(`$${prodTotal.toFixed(2)}`);
}
listProducts();
2 Answers
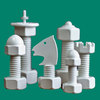
Steven Parker
241,809 PointsThe original code met the criteria of creating two functions, one which returned an array of names, and another which returned a value total. The console.log
statements were only used to confirm the function returns.
What you've done here is make the logging itself the primary intent of the program instead of creating functions to perform the two different tasks. For this objective, the function is actually superfluous and can be removed to make the code even more compact.
The important thing to recognize is that you accomplished different goals than what was done in the video. I'd say you did an equally good job of doing a different task.
You might be interested to know that there are array methods that can be used to both accomplish the original goals and make the code even more compact that what you have here. For example:
var listProducts = a => a.map(p=>p.name);
var totalValue = a => "$"+a.reduce((s,p)=>s+=p.unit_price*p.inventory,0).toFixed(2);
console.log(listProducts(products));
console.log(totalValue(products));
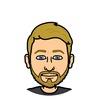
Lewis Marshall
22,673 PointsHeres my answer using some ES5 and ES6.
const products = [
{
name: "Destiny",
inventory: 4,
unit_price: 29.99
},
{
name: "GT Sport",
inventory: 5,
unit_price: 27.99
},
{
name: "Fortnite",
inventory: 3,
unit_price: 24.99
}
];
const listProducts = products => products.map(product => product.name);
console.log(listProducts(products));
const totalValue = products => products.map(product => product.inventory * product.unit_price)
.reduce((total, price) => total += price);
console.log(totalValue(products));
// [ 'Destiny', 'GT Sport', 'Fortnite' ]
// 334.88
paulscanlon
Courses Plus Student 26,735 Pointspaulscanlon
Courses Plus Student 26,735 PointsHey
Nice answer thanks a lot. Also thanks for sticking up for me in the other question, I totally got in there first lol
Thanks again
Paul
Yasir alabadi
5,744 PointsYasir alabadi
5,744 Pointshey! I was wondering as to why you have two sets of ' =>'
I tried the following.map method, didn't seem to work?
Steven Parker
241,809 PointsSteven Parker
241,809 PointsEach "=>" defines a function, and there are two functions being defined here: "listProducts" and "totalValue".
Your code should work, it's the equivalent of defining and then calling the first function.
And for future questions, always start a fresh question instead of posting to someone's "answer".