Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial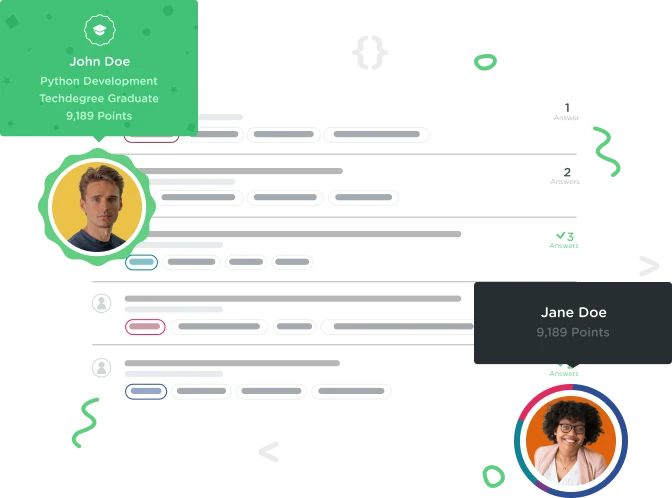
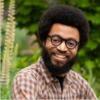
Nicolas Hampton
44,638 PointsMoving forward...
Now that I've completed the course track so far (though I will quickly come back once the next class was done, Craig, you're a great teacher!) I do have a few questions as I start a few projects of my own, like:
I use a chromebook when moving around. What's the best online IDE for this, and should I structure the files in it with future development in mind (we did a little of that, but move explination would help)?
Do you have any experience in Guava from Google to know if that would be a good and easy upgrade to the sdk?
What kind of projects will employers (or anybody) typically be looking for Java development for, in your experience?
I'm pretty new to programming, so direct me a few places, just thought you'd know my level, as where you left us off in the class.
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
// TODO(2): Add to the course videos as the second video.
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
return null;
}
}
1 Answer
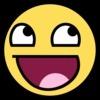
Michael Hess
24,512 PointsHi Nicolas,
I'm not sure if this was directed at Craig but here's my opinion:
For right now, you'll probably be alright using an IDE like Eclipse or Netbeans -- you may even want to try making a few projects with the console/terminal (the javac and java commands). Also, you may want to pick up a text book from the library, or buy one, that covers Java, too. One very well written book I like, that is available for free online, is Thinking In Java by Bruce Eckel. I believe it has practice exercises at the end of every chapter, too.
Here's a list of potential practice projects: http://blog.programmersmotivation.com/2014/07/09/list-projects/
This is a glimpse at what your future holds if you decide to follow the Java Developer road:
Most employers use J2EE or Java Enterprise Edition. J2EE applications usually have a HTML, CSS, JavaScript, Struts, and/or Tiles front-end, with a java middle layer, and a database (usual they follow the MVC or MVVM or MOVE development patterns but some don't). Many employers will want you to know ORM (Object Relation Mapping). Check out Spring, iBatis, and/or Hibernate. ORM frameworks solve the problem of object relational mapping impedance mismatching -- i.e. persisting Java objects or POJOs to a database. Plus, servlet containers like: Tomcat. Of course the ability to think critically and solve problems is highly prized, too. Some knowledge of SQL (making basic queries & creating tables is really all you need).
Many employers will want employees to know how to unit test -- usually with jUnit (If I recall Craig mentioned, in one of his videos, that he would be covering unit testing in a future course).
In addition, many employers will want employees to know basic Java: basic control structures, keywords, variables, arrays, interfaces, lists, arraylists, sets, maps, OOP/OOD (polymorphism, inheritance, encapsulation, abstraction), etc, and have good communication skills (both written and verbal). Craig has covered a great deal of these topics.
This is pretty much what you'll find in most job descriptions for noobie Java Developers.
**But starting out, many employers will only require that you have the fundamental Java skills and a positive attitude, so that they can train you further. Many employers will train junior developers --but some won't .*******
Check out Hibernate: http://hibernate.org/ Check out Spring: http://projects.spring.io/spring-framework/ Check out iBatis: http://ibatis.apache.org/ Check out Tomcat: https://tomcat.apache.org/download-80.cgi Check out Eclipse: https://www.eclipse.org/downloads/ Check out Netbeans: https://netbeans.org/downloads/ Check outThinking in Java 4th Edition: http://www.saeedsh.com/resources/Thinking%20in%20Java%204th%20Ed.pdf
Hope this helps!
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsThanks so much Michael, that gives me a lot of direction!