Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial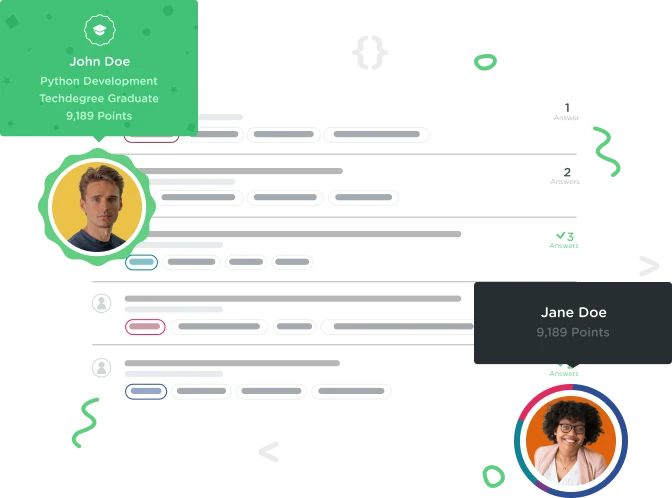

Radovan Mencik
9,552 Pointsmost_courses
Hello, could anybody help me with this challege? It seems to work well in IDLE and the Workspaces. So where is the problem?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
teachers = {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
'Kenneth Love': ['Python Basics', 'Python Collections', 'Python mythology'],
'Radovan':['Lumberjack song']}
def num_teachers(d):
tlist = []
for i in d.keys():
tlist.append(i)
a = len(tlist)
return a
def num_courses(d):
course = 0
for value in d.values():
for things in value:
course += 1
return course
def courses(d):
lessons = []
for i in d.values():
lessons += i
return lessons
def most_courses(d):
for i, h in d.items():
a = len(h)
d[i]= str(a)
high = []
for x in d.values():
high.append(x)
num = str(max(high))
for k, v in d.items():
if num in str(v):
name = str(k)
return name
1 Answer
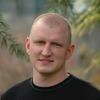
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsSo at the top of the challenge, Kenneth has given you some sample data of what MIGHT be passed in, so you have an idea of what data types you might work with. But for this challenge to pass, it may be testing your most_courses function against more than 1 sample data. The one he gives you a sample is "1 scenario" think about other scenarios he could pass for the arguments.
Maybe in scenario 1, he passes you, 2 teachers and 1 teacher has 3 courses and the other has 2. Easy your code works for that. But maybe Scenario 2, he passes you an Empty Dictionary that has 0 teachers, does your code still work or does it crash with an Error? Maybe scenario 3 he passes you 3 teachers who all have the same amount of courses... well how do you decide which teacher has more courses if they are all the same?
Just know that the sample data isnt the only thing being tested, your code has to work for more "Test cases" than just the 1 example.
Also side note: I noticed you are looping 3 times in your 1 function. Normally you dont have to jump into a separate loop each time you need to do something. Usually you only have to loop twice if you are traversing Lists of lists or something. But if you are traversing 1 list of items you should be able to do everything in that one loop. Just something to note, because maybe your function is passed a HUGE list of teachers and courses with possibly hundred thousand or more items. Well your function will exponentially raise in time it takes to run because it has two traverse those items 3 separate times instead of just once. If that makes sense?