Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial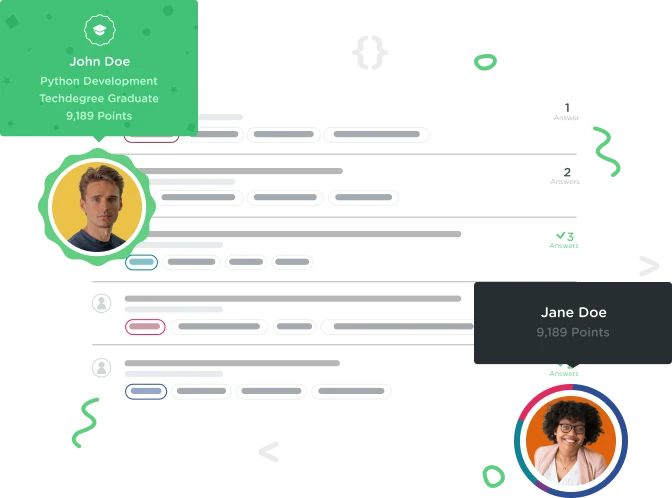

Raju Ramesh
307 PointsMethods
Can anyone please give me the code because it's a bit confusing to me.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I'm a machine!")
}
}
class Robot: Machine {
2 Answers

Dhanish Gajjar
20,185 PointsYou should try to go back and understand the concepts clearly. Below is the code. Hope it helps you understand.
class Robot:Machine {
override func move(_ direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}

Dhanish Gajjar
20,185 PointsRaju Ramesh The _ is used when you want to omit the external name for an argument. I strongly recommend visiting older videos where Pasan explains that arguments in swift can have 2 names, external and internal.
If the external name is not omitted, when you call the function move on an instance of a Robot
let robot = Robot()
robot.move(direction: "Up")
It would read like move ( direction : up )
whereas If it is omitted using an _
let robot = Robot()
robot.move("Up")
This would read like move up. It is not mandatory to always omit the external name. It is what shows up as a label when you call the function. By omitting it, direction becomes the internal name, which you can use within the function, but it won't show up elsewhere.
Hope it's not too confusing, and I have made it clear.
Raju Ramesh
307 PointsRaju Ramesh
307 PointsWhen to use underscore in function parameter brackets??