Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial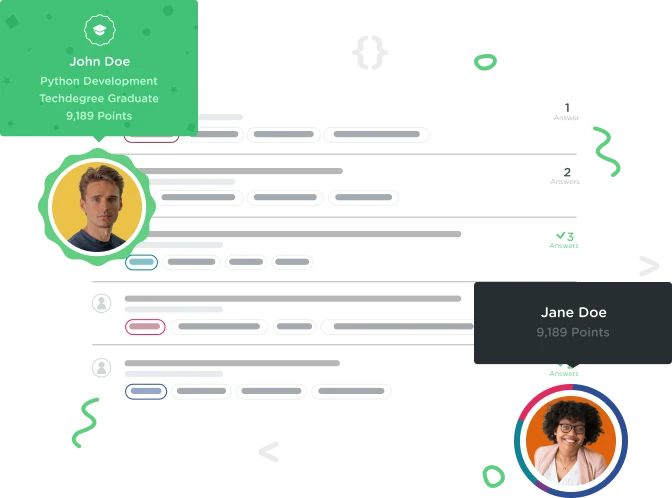
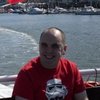
Sean Flanagan
33,236 PointsMaps again
Hi. This is my latest attempt at this question. I know I've started other threads about it so I beg your forbearance. The editor has spotted 9 errors.
I know people have tried hard to help so thanks. :-)
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
Map<String, Integer> blogPost = new HashMap<String, Integer>();
for (String hashMap : blog.getCategoryCounts()) {
Integer count = categoryCounts.get(hashMap);
if (count == null) {
count = 0;
}
count++;
hashMapCounts.put(hashMap, count);
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
6 Answers
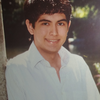
Jon Kussmann
Courses Plus Student 7,254 PointsSometimes when you are learning a lot of new information, you can "forget" about some of the previous things you learned. You have all of the logic there, just need to wrap it in a method.
First step is to create a method called getCategoryCounts that returns a Map of category to count (String, Integer):
public Map<String, Integer> getCategoryCounts() {
}
Since we know we are going to return a Map<String, Integer> from the method declaration, let's go ahead and make a new one:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> result = new HashMap<String, Integer>();
}
The new line above is the same as the first line you have added (I called it results, while you called it blogPost).
You should be able to add the rest of what you have. As a side note, I prefer the way you solved it in your other post. It's a little more "readable" to me. I'll be checking back if you need more clarification.

Craig Dennis
Treehouse TeacherMap<String, Integer> blogPost = new Map<String, Integer>();
Anything look wrong about this line?
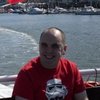
Sean Flanagan
33,236 PointsI've not declared it public.

Craig Dennis
Treehouse TeacherLooks like you accidentally deleted the method declaration ;)
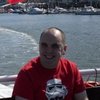
Sean Flanagan
33,236 PointsHi Craig. Does this mean I forgot to declare it public?
Thanks :-)
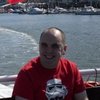
Sean Flanagan
33,236 PointsHi Jon. I've got it now.
I should have instantiated the implementation (HashMap) instead of the interface.
In retrospect it doesn't make sense to declare blogPost twice, whereas it does make sense to create an instance (getCategory) from String category.
The issue has been resolved and you and Craig both deserve a stiff drink after all the help you've given me here.
Thank you both very much and I shall remember this. Please let me know if there's anything I can do.
Sean :-)
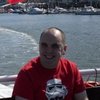
Sean Flanagan
33,236 PointsIs my not declaring it public what's wrong?
Thanks :-)

Craig Dennis
Treehouse TeacherHi Sean,
I am having a hard time understanding where you are at now. Originally there was no method declaration.
The line I was talking about, Jon furthered the hint, looks like you had the variable name wrong and had tried to instantiate the interface and not the implementation.
If that doesn't fix things, please re-post code and errors. You are super close!
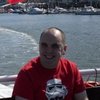
Sean Flanagan
33,236 PointsThank you both for your extreme patience throughout.
I've changed the code again. It's still not perfect:
package com.example;
import java.util.HashMap;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> blogPost = new Map<String, Integer>();
for (BlogPost post : mPosts) {
String blogPost = post.getCategory();
Integer count = blogPost.get(category);
if (count == null) {
count = 0;
}
count++;
blogPost.put(category, count);
}
return blogPost;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
And the errors:
./com/example/Blog.java:19: error: cannot find symbol public Map getCategoryCounts() { ^ symbol: class Map location: class Blog ./com/example/Blog.java:20: error: cannot find symbol Map blogPost = new Map(); ^ symbol: class Map location: class Blog ./com/example/Blog.java:20: error: cannot find symbol Map blogPost = new Map(); ^ symbol: class Map location: class Blog ./com/example/Blog.java:23: error: cannot find symbol Integer count = blogPost.get(category); ^ symbol: variable category location: class Blog ./com/example/Blog.java:28: error: cannot find symbol blogPost.put(category, count); ^ symbol: variable category location: class Blog Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 5 errors
If I don't reply immediately, it's just because I'm about to head to work and my mobile phone isn't internet-capable but I'll check in when I get home.
Thank you :-)
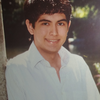
Jon Kussmann
Courses Plus Student 7,254 PointsHi Sean,
This is what you currently have:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> blogPost = new Map<String, Integer>();
for (BlogPost post : mPosts) {
String blogPost = post.getCategory();
Integer count = blogPost.get(category);
if (count == null) {
count = 0;
}
count++;
blogPost.put(category, count);
}
return blogPost;
}
The first error is that you are attempting to instantiate an interface (Map). As Craig stated above, you should be using an implementation - in this case HashMap:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> blogPost = new HashMap<String, Integer>(); //Look over here!
for (BlogPost post : mPosts) {
String blogPost = post.getCategory();
Integer count = blogPost.get(category);
if (count == null) {
count = 0;
}
count++;
blogPost.put(category, count);
}
return blogPost;
}
The second error is you are attempting to re-declare blogPost:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> blogPost = new HashMap<String, Integer>();
for (BlogPost post : mPosts) {
String blogPost = post.getCategory(); // I think you mean String category = post.getCategory();
Integer count = blogPost.get(category);
if (count == null) {
count = 0;
}
count++;
blogPost.put(category, count);
}
return blogPost;
}
Sean Flanagan
33,236 PointsSean Flanagan
33,236 PointsHi Jon.
I've taken another crack at my Blog class. There are still some errors but not as many. Progress, I guess lol. Here it is:
And the errors:
Thanks Jon. :-)
Jon Kussmann
Courses Plus Student 7,254 PointsJon Kussmann
Courses Plus Student 7,254 PointsHi Sean,
Craig has posted a big hint below.
When you declare your Map, you called it "blogPost"... but you are using "categoryCounts" for your different operations. I think it was a copy + paste error from combining the code from your two different threads.
The second has to do with the other side... when you instantiate your Map. You can't instantiate Map directly. Try using HashMap instead.