Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial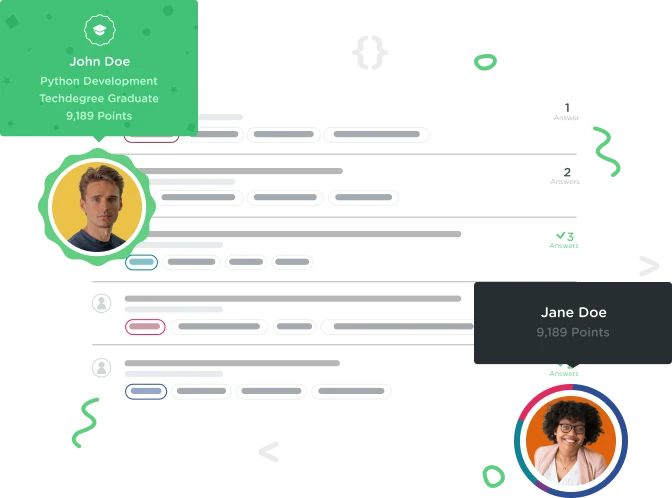
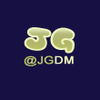
Jonathan Grieve
Treehouse Moderator 91,253 PointsMap Collections - Review
Every key has at the most ONE value. Maps match keys to values.
problems solved include counts of values and categorising items into groups.
With maps you define types for the keys and the values like so. ( Map <String, String> nameOfMap)
e.g.
Map<String, String> name = new HashMap<String, String>();
- You can add to maps with the put() method which takes a key and a value as its parameters.
name.put("YANA", "You Are Not Alone");
This returns null first time when using the put method on a map because originally Maps are created with no initial value. Each put method returns the previous value of a particular key.
- Keys can be set to null
other methods
- The Map interface includes methods for basic operations (such as put, get, remove, containsKey, containsValue, size, and empty), bulk operations (such as putAll and clear), and collection views (such as keySet, entrySet, and values).
+.remove("key") - Remove key value pairs in a Map by the key. returns value when removing +.contains() - check a map has a particular key.
- .get() - Check a key exists
Using KeySet() - return all the keys in a set
e.g.
Set<String> variable = variable.keySet();
------> EntrySet() used to loop through keys and values. Map.Entry is an interclass, only used within the map, only available to the map.
for ( Map.Entry entry: variable.entrySet() ) {
System.out.printf("%s stands for %s %n, entry.getKey(), entry.getValue() );
}
Other implementations
In the documentation K stands for the type and V, the type of value. There are interfaces for HashMap, Map with HashMap being the default. There's also LinkedHashMap inteface
- Interface Map<K, V>
- Class HashMap<K,V>
- Class LinkedHashMap<K,V> - maps with values in the order they were added
- Class TreeMap - predictable sorting order
e.g. For each treet, loop through each HashTag.
Map<String, Integer> hashTagCounts = new HashMap<String, Integer>();
for (Treet treet : treets) {
for(String hashTag : treet.getHashTags() ) {
Integer count = hashTagCounts.get(hashTag);
if(count==null) {
count=0;
}
count++;
hashTagCounts.put(hashTag, count);
}
}
e.g. A map that is keyed on "author"
Map<String, List<Treet>> treetsbyAuthor = new HashMap<String, List<Treet>>();
for(Treet treet : treets) {
List<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
if(authoredTreets == null) {
authoredTreets = new ArrayList<Treet>();
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
}
authoredTreets.add(treet);
}
System.out.printf("Treets by author: %s %n", treetsByAuthor ); System.out.printf("Treets by nickrp: %s %n", treetsByAuthor.get("nickrp") );
- The use of the Collections Maps, Lists and Sets are very common in Java.
- Always use the interface for the type declaration. You can change the implementation later if you need to.
- Queue is another interface.
Collections Recap
List - List<String> variable= new ArrayList<String>(); Sets - Set<String> variable = new Set<String>(); Maps - Map<String, String> variable = new HashMap<String, String>();
Nelson Fleig
25,549 PointsNelson Fleig
25,549 PointsThank you for the recap Jonathan!