Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial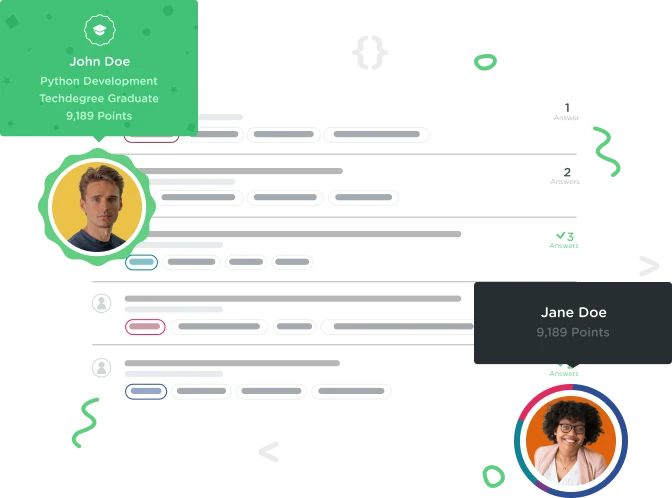

Christina Pruitt
3,000 PointsMaking conetations
I don't know what I'm doing wrong. I'm trying to do what they're asking in the prompt, but it keeps telling me " Bummer! Did you add the '#' character between id
and lastName
?"
`
var id = "23188xtr";
var lastName = "Smith";
var userName = "Kyle";
userName.toUpperCase();
userName += 'id' + '#' + 'lastName';
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
4 Answers
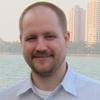
Ryan Field
Courses Plus Student 21,242 PointsI'm not exactly sure what the final output of the challenge is supposed to be, but it looks like you're trying to make it output the id
and lastName
variables connected by a #
in the middle. In that case, you need to unquote id
and lastName
as they are just strings with the quotes and will output KYLEid#lastName
regardless of what the variables are. You want something like this:
userName += id + '#' + lastName;
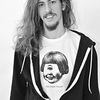
eck
43,038 PointsOne of the problems I can see is that you are not referencing the id and lastName, but instead you are only adding a string to userName.
This is what you want:
userName += id + '#' + lastName;

Christina Pruitt
3,000 PointsI'm still confused. Why?
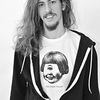
eck
43,038 PointsWell, in this example, if you write: lastName, it is expected that lastName is a variable, but if you write: "lastName" you have entered a string value. Below is a small example of what the difference would could look like:
// Here we declared a variable that contains a STRING value
var lastName = "Pruitt";
// When this code is run, the browser will create an
// alert with the word (a string value) "Pruitt" written in it.
alert(lastName);
// This code will also create an alert, but the text will say "lastName"
// instead of "Pruitt", since by putting lastName in quotes we tell
// the browser that is not a variable we are referencing, but instead
// we are just giving it a text value know as a string.
alert("lastName");
I hope that helps!

Ira Bradley
12,976 Points@Christina what is the string you are trying to create? I looked at the challenge and my code posted above works correctly. If you are trying to create the string "KYLE23188XTR#SMITH" than you need to use toUpperCase() on all strings like this:
var firstName = "Kyle"
var userName = firstName.toUpperCase() + id.toUpperCase() + '#' + lastName.toUpperCase();
From my understanding they want the first solution posted however.
Ira Bradley
12,976 PointsIra Bradley
12,976 PointsThe problem is that your lastName is not uppercased. Also don't use single or double quotes around variables. The computer understands when a variable is a string.