Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial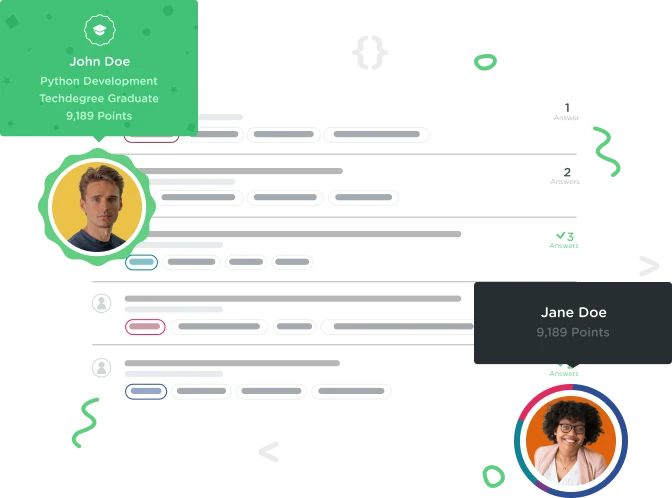
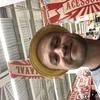
Gennadii Chamorsov
9,207 PointsLogical OR operator doesn't work with digits and words together in my code.
Please help me to find a mistake. I used the logical OR operator for this conditional statement:
let correctGuessFingers = false;
const fingers = prompt('How many fingers has the human?');
if ( fingers === 20 || fingers === 'Twenty' ) {
correctGuessFingers = true;
console.log("it's true");
}
Only the answer 'Twenty' works but '20' doesn't. Thanks in advance.
4 Answers
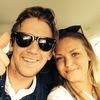
Jonathan Hermansen
25,935 PointsYou need to parse your fingers-variable before comparing it to a number. Now you compare a string to a number ("20" === 20)
, it will always return false.
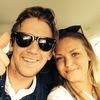
Jonathan Hermansen
25,935 PointsThat should work.

Hussein Ammar
11,106 Pointslet correctGuessFingers = false;
let fingers = prompt('How many fingers has the human?');
if ( fingers === "20" || fingers === 'Twenty' ) {
correctGuessFingers = true;
console.log("thats true");
} else {
console.log("Not true");
}
I tested your code and it should work!

Busra Tokuc
7,444 PointsThe value returned by the prompt method is a string. So you should convert the string value to a number value before comparing them. You can do this by adding a plus symbol(Unary operators) just before the fingers variable. You can check unary operators on MDN. (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators#Unary_operators)
if ( +fingers === 20 || fingers === 'Twenty' ) {
correctGuessFingers = true;
console.log("it's true");
}
Gennadii Chamorsov
9,207 PointsGennadii Chamorsov
9,207 PointsThanks for checking! I tried it this way:
And still, doesn't work.