Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial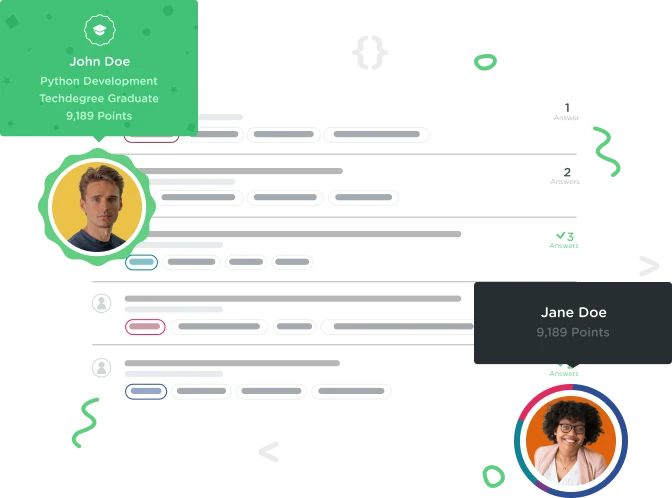

Miguel Nunez
1,081 PointsLocal variable
Why do you need to store that call in "letter"?
public boolean applyGuess(char letter) {
letter = normalizeGuess(letter);
normalizeGuess(letter); //wouldn't this be good enough?
boolean isHit = answer.indexOf(letter) != -1;
if (isHit) {
hits += letter;
} else {
misses += letter;
}
return isHit;
}
5 Answers

Tabatha Trahan
21,422 PointsYou need to store the value returned by normalizeGuess. If you just make a call to that method and don't store the value, you can't really do anything with it. So for this one, you are taking the argument passed in as letter, calling the normalizeGuess on that value, and then storing it in a variable named letter to use in the rest of the applyGuess method. So when ever you call a method that returns a value, you'll want to be able to store that value in a variable for future use.

Tabatha Trahan
21,422 PointsIt's all about variable scope. You are passing in a value stored in your letter variable. The method then takes it and does it's thing and returns a new value. The rest of your code has no idea what the method is doing- your original value stored in letter is not changed. Try it out by creating a method that accepts a parameter called num and returns num + 3. Outside of the method, create a variable called num and assign it a value. Call the method and pass it your variable num. If you don't save the returned value, it disappears- call the printf method to see what's in num outside of the method. So yes, you are passing letter to your normalizeGuess method, and that method is returning a value, but it is not changing the original value of letter that is outside of the method so you still need to store the value returned by the method if you want to use it for anything else within your program. Does that make sense?

Miguel Nunez
1,081 Points"you are passing letter to your normalizeGuess method, and that method is returning a value, but it is not changing the original value of letter that is outside of the method"
"the original value of letter that is outside of the method" -- Do you mean the value in "letter" returned by the normalizeGuess() ?

Tabatha Trahan
21,422 PointsI'm sorry I'm not very good at explaining this. So you have letter that holds a certain value outside of the normalizeGuess method. You are passing that value to the normalizeGuess method, which is being assigned to a local variable within the method. This value is manipulated and returned by the method. The original value of letter (assigned outside of the normalizeGuess method) has not changed, so you need to assign the results of the normalizeGuess method to it in order to use the results of the method. I'll try to find a good treehouse video that explains variable scope, as the teachers are way better at explaining things like this.

Miguel Nunez
1,081 PointsI think that what you're getting at here is that the variable or argument passed in to the applyGuess(letter) method has a different location in memory than the variable "letter" returned by the normalizeGuess(letter) method. So there is no "clash". That's why that assignment letter = normalizeGuess(letter) makes sense. Correct?

Tabatha Trahan
21,422 PointsYes, that's pretty much what I'm trying to say. You just need a variable to put the method result in so you can use it later in the program. There is no clash because letter in normalizeGuess(letter) is a parameter that uses the value passed to it and becomes a local variable known only to that method.
Miguel Nunez
1,081 PointsMiguel Nunez
1,081 PointsI get that. But what's the use of it in this specific context? We're using the parameter, aren't we?