Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial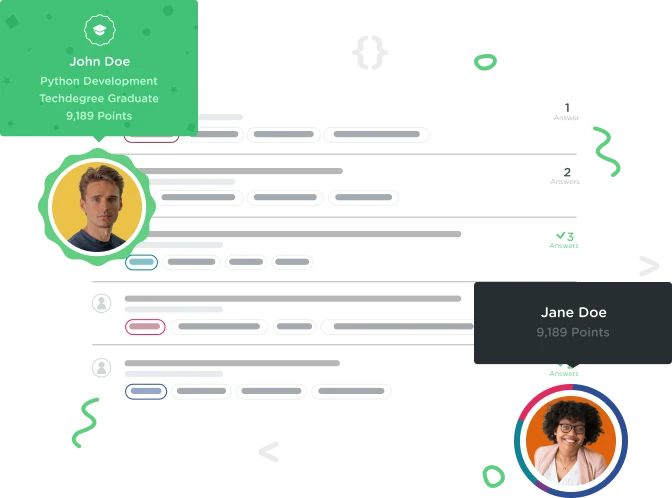
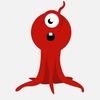
Justin Kraft
26,327 PointsLast Code Challenge of Java Data Structures - Worked in a different IDK but not here.
I cannot seem to pass this part of the challenge. I copied my code from each class to Eclipse and ran a dummy test to see if I could add a video and change the title and with the code below, it worked. Unfortunately, it does not work here and I cannot locate the issue. Thanks in advance.
import com.example.model.Course;
import com.example.model.Video;
import java.util.List;
import java.util.HashMap;
import java.util.Map;
public class QuickFix {
private static Map<String, Video> videoMap = new HashMap<String, Video>();
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video newVid = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
course.setVideos(1, newVid);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> videos = videosByTitle(course);
Video videoValue = videos.get(oldTitle);
videoValue.setTitle(newTitle);
}
public Map<String, Video> videosByTitle(Course course) {
List<Video> vids = course.getVideos();
for (Video vid : vids) {
videoMap.put(vid.getTitle(), vid);
}
return videoMap;
}
}
2 Answers
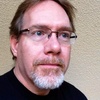
Chris Freeman
Treehouse Moderator 68,426 PointsI made the following changes to your code to get it to pass:
// QuickFix.java
import com.example.model.Course;
import com.example.model.Video;
import java.util.List;
import java.util.HashMap;
import java.util.Map;
public class QuickFix {
// remove class field. Make it local to videosByTitle
// private static Map<String, Video> videoMap = new HashMap<String, Video>();
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video newVid = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
// No '.setVideos()' method exists in challenge
// Get list of current videos from course
List<Video> currentVideos = course.getVideos();
// Add newVid to this list
currentVideos.add(1, newVid);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> videos = videosByTitle(course);
Video videoValue = videos.get(oldTitle);
videoValue.setTitle(newTitle);
}
public Map<String, Video> videosByTitle(Course course) {
// Create local video by title Map
Map<String, Video> videoMap = new HashMap<String, Video>();
List<Video> vids = course.getVideos();
for (Video vid : vids) {
videoMap.put(vid.getTitle(), vid);
}
return videoMap;
}
}

patateskafa
7,876 Points public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
videosByTitle(course).get(oldTitle).setTitle(newTitle);
}
this one line also works. you use the previously created videosByTitle method, pass in a course object which returns you a HashMap, you use the get() method and pass in the oldTitle which is the "key" of the map and it returns you the "value" pair of the key (which is a Video object) and then you call the setTitle() method defined in the Video class and pass in the newTitle.