Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial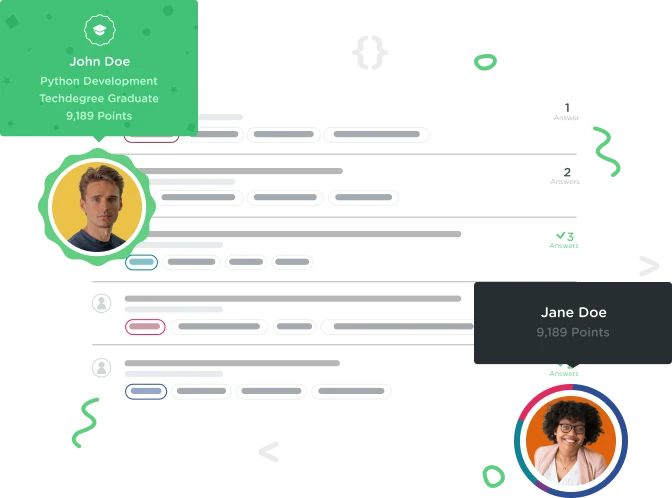
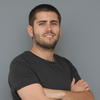
Can Uludag
Courses Plus Student 8,769 PointsKeeps saying "Oops! It looks like Task 1 is no longer passing."
Hi,
Whenever I try to check the code, it keeps saying "Oops! It looks like Task 1 is no longer passing". But preview seems fine.
What could be the problem?
Thanks
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
try{
this.discountCode = normalizeDiscountCode(discountCode);
} catch(IllegalArgumentException iae){
System.out.println(iae.getMessage());
}
}
private String normalizeDiscountCode(String discountCode){
for(char c : discountCode.toCharArray()){
if(!Character.isLetter(c) || String.valueOf(c) != "\\$"){
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}

Frederick Reiss
15,003 PointsI am not sure why, I updated one line of code in step two of the challenge and everything worked
( this.discountCode = normalizeDiscountCode(discountCode); )
public void applyDiscountCode(String discountCode) { this.discountCode = normalizeDiscountCode(discountCode); }
private String normalizeDiscountCode (String discountCode) {
for (char letter : discountCode.toCharArray()) { if (!Character.isLetter(letter) && letter != '$') { throw new IllegalArgumentException("Invalid discount code"); } } return discountCode.toUpperCase(); } }
3 Answers

Frederick Reiss
15,003 PointsCan,
1. You may be correct, but having worked as a functional software tester I understand how requirements are constantly being changed or updated. Sometimes the software tester is the last to find out what the real requirements might be.
2. Here is the code segment that worked for me, others are free to correct me because I am here to learn.
SUCCESS
public void applyDiscountCode(String discountCode) { this.discountCode = normalizeDiscountCode(discountCode); }
5. private String normalizeDiscountCode (String discountCode) { for (char letter : discountCode.toCharArray()) { if (!Character.isLetter(letter) && letter != '$') { throw new IllegalArgumentException("Invalid discount code"); } } return discountCode.toUpperCase(); } }

Frederick Reiss
15,003 PointsHello Jennifer,
I posted above trying to help 21 hours ago by Can Uludag...
Do you have a suggestion how to proceed, I am trying to understand what is happening.
In my searching I found another link:( https://teamtreehouse.com/community/bug-with-the-exercise-maybe-i-solved-it-but-i-dont-know-how-please-explain )
The suggestion was made that perhaps the requirements are not correct, is that True?
-Fred
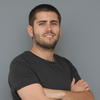
Can Uludag
Courses Plus Student 8,769 PointsSuprisingly, changing if(!Character.isLetter(c) || c != '$')
to if(!Character.isLetter(letter) && c != '$')
solved the issue. But I think it is against the rules of the challenge. Letter should not be numeric character. It can only be a letter or dollar sign. So || (or) is much more understandable than && (and) logic.
Frederick Reiss
15,003 PointsFrederick Reiss
15,003 PointsCan Uludag,
The Code below works for Challenge 1 of 2 for both the "Preview" & "Recheck work"
For Challenge 2 of 2 the Preview - results: Invalid discount code
clicking on the Recheck work button results with: Bummer! Hmmm...I ran order.applyDiscountCode("h1!") and I expected it to throw an IllegalArgumentException, but it didn't.
If the try/catch block is removed the Preview is blank.
public void applyDiscountCode(String discountCode) { } }
The "Recheck work" result is: Oops! It looks like Task 1 is no longer passing.
Go back to task one.. If the user puts the try/catch back in the result is: Bummer! Hmmm...I ran order.applyDiscountCode("h1!") and I expected it to throw an IllegalArgumentException, but it didn't.
...... // https://teamtreehouse.com/community/bug-with-the-exercise-maybe-i-solved-it-but-i-dont-know-how-please-explain private String normalizeDiscountCode (String discountCode) { for (char letter : discountCode.toCharArray()) { if (!Character.isLetter(letter) && letter != '$') { throw new IllegalArgumentException("Invalid discount code"); } } return discountCode.toUpperCase(); }
public void applyDiscountCode(String discountCode) { try { this.discountCode = normalizeDiscountCode(discountCode); } catch (IllegalArgumentException iae) { System.out.println(iae.getMessage());
} } }
Can someone explain what's going on here... Thanks, Fred