Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial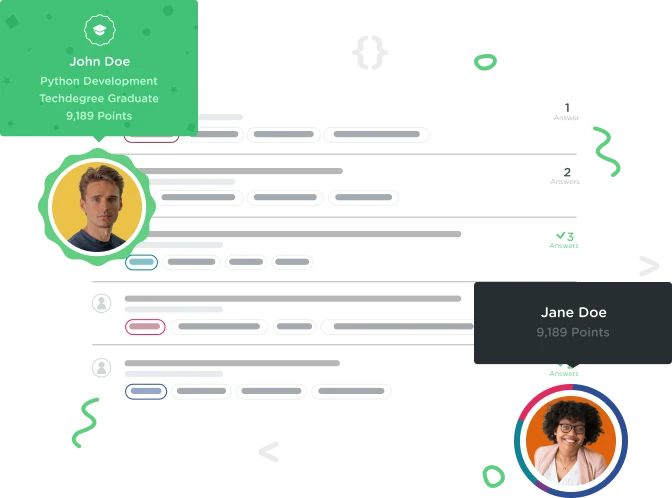

Antony Gabagul
9,351 PointsKeep getting : TypeError: 'undefined' is not an object (evaluating 'listItems[i].style')
I keep getting TypeError: 'undefined' is not an object (evaluating 'listItems[i].style') and have no idea how to properly select by id
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
let listItems = document.querySelectorAll('#rainbow');
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
3 Answers

Stephan Olsen
6,650 PointsWhat's happening is that you're selecting the whole node. This means that you're basically selecting this:
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
querySelectorAll returns a NodeList, which is why when you're iterating over it, will be able to set it to the first color in your array, but after that it will return undefined, as there are no other items in the NodeList. What you need to do is select all the elements in the list, and not just the ul element. Below should fix it for you.
document.querySelectorAll('#rainbow > li');
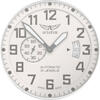
Martin Balon
43,651 PointsHi Anton, you are really close - in query selector add 'li' after #rainbow. Code should look like this:
let listItems = document.querySelectorAll('#rainbow li');
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
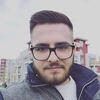
Florin Truscan
6,782 Points"should contain all list items in the <ul>" it all makes sense now.