Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial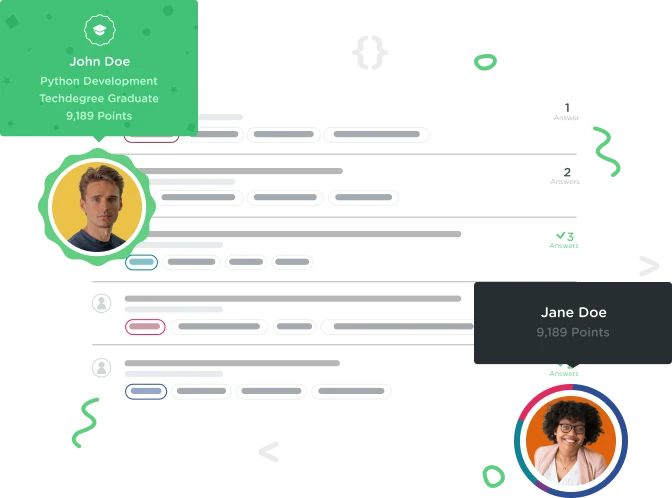

user one
31 Pointskeep getting java.lang.nullpointerexception?
here is the code. im getting the java.lang.nullpointerexcpetion on lines 21,25,134,175
import java.util.Scanner; public class TicTacToe { private char[][] board; private boolean xTurn; private Scanner keyboard;
public void TicTacToe()
{
board = new char[3][3];
for(int r = 0; r < 3; r++)
{
for(int c = 0; c < 3; c++)
board[r][c] = ' ';
}
xTurn = true;
keyboard = new Scanner(System.in);
}
private void displayRow(int row)
{
System.out.println(" " + board[row][0] + " | " + board[row][1] + " | " + board[row][2]);
}
private void displayBoard()
{
displayRow(0);
System.out.println("-----------");
displayRow(1);
System.out.println("-----------");
displayRow(2);
}
private void displayMenu()
{
if(xTurn)
System.out.println("X's Turn!");
else
System.out.println("O's Turn!");
System.out.println("What would you like to do?");
System.out.println("1: Make a move");
System.out.println("2: Start Over");
System.out.println("3: Quit");
System.out.print("Choice: ");
}
private boolean getMove()
{
boolean invalid = true;
int row = 0, column = 0;
while(invalid)
{
System.out.println("Which row, column would you like to move to? Enter two numbers between 0-2 separated by a space to indicate position.");
row = keyboard.nextInt();
column = keyboard.nextInt();
if(row >= 0 && row <= 2 && column >= 0 && column <= 2)
{
if(board[row][column] != ' ')
System.out.println("That position is already taken");
else
invalid = false;
}
else
System.out.println("Invalid position");
}
if(xTurn)
board[row][column] = 'X';
else
board[row][column] = 'O';
return winner(row,column);
}
private void restart() {
for(int r = 0; r < 3; r++)
{
for(int c = 0; c < 3; c++)
board[r][c] = ' ';
}
xTurn = true;
}
private boolean winner(int lastR, int lastC)
{
boolean winner = false;
char symbol = board[lastR][lastC];
int numFound = 0;
for(int c = 0; c < 3; c++) {
if(board[lastR][c] == symbol)
numFound++;
}
if(numFound == 3)
winner = true;
numFound = 0;
for(int r = 0; r < 3; r++) {
if(board[r][lastC] == symbol)
numFound++;
}
if(numFound == 3)
winner = true;
numFound = 0;
for(int i = 0; i < 3; i++) {
if(board[i][i] == symbol)
numFound++;
}
if(numFound == 3)
winner = true;
numFound = 0;
for(int i = 0; i < 3; i++) {
if(board[i][2-i] == symbol)
numFound++;
}
if(numFound == 3)
winner = true;
return winner;
}
private boolean boardFull() {
int numSpotsFilled = 0;
for(int r = 0; r < 3; r++) {
for(int c = 0; c < 3; c++) {
if(board[r][c] == 'X' || board[r][c] == 'O')
numSpotsFilled++;
}
}
return numSpotsFilled == 9;
}
public void play() {
while(true) {
displayBoard();
displayMenu();
int choice = keyboard.nextInt();
if(choice == 1) {
if(getMove()) {
displayBoard();
if(xTurn)
System.out.println("X Wins!");
else
System.out.println("O Wins!");
System.exit(0);
}
else if(boardFull()) {
displayBoard();
System.out.println("Draw!");
System.exit(0);
}
else {
xTurn = !xTurn;
}
}
else if(choice == 2)
restart();
else if(choice == 3)
System.exit(0);
else
System.out.println("Invalid Option");
}
}
public static void main(String[] args) {
TicTacToe game = new TicTacToe();
game.play();
}
}
1 Answer

Tyler B
5,787 PointsI assume your intent was to create a constructor on line ~8. Constructors don't have return types remove the void so it reads public TicTacToe()
that your private fields will have a non null value.