Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial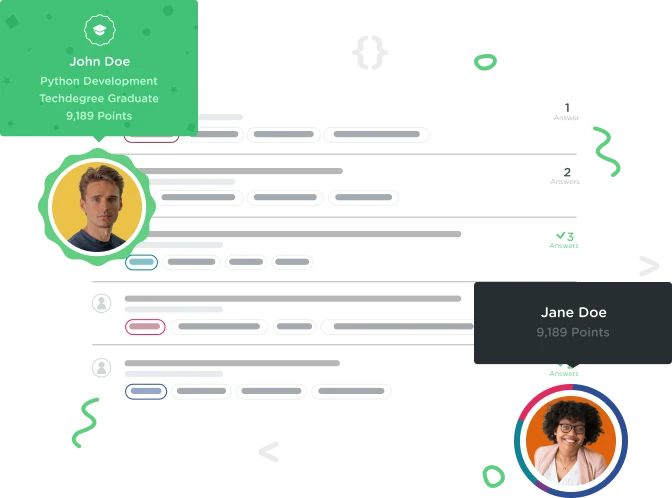
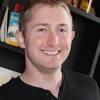
Phil Spelman
6,664 PointsJUnit 5
Hi Craig! I was hoping you might add some notes or another video showing the differences between JUnit4 and JUnit5. A couple things I've run into is that they no longer support the @Test() parameter for expected exceptions, and that there is no longer support for @Rule annotations like with JUnit4.
If you end up putting together material on that I will for sure check it out!
3 Answers

Craig Dennis
Treehouse TeacherWe were just talking about this. I've added it to the Java Content Trello Roadmap. Thanks Phil!

Arnór Kristmundsson
2,013 PointsHey,
here is my solution for the overstockingIsNotAllowed()
in jUnit 5:
@Test
void overstockingIsNotAllowed() throws Exception{
assertThrows(IllegalArgumentException.class, () -> {
bin.restock("Fritos", 2600, 100, 50);
});
}
but I was wondering, is it possible to check the error message in jUnit 5?
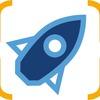
codeboost
6,886 PointsI guess this is a bit of a late reply, but yes, and there are multiple ways. The easiest I found is this one:
@Test
void overstockingIsNotAllowed() throws Exception{
Exception exception = assertThrows(IllegalArgumentException.class,
() -> bin.restock("Fritos", 2600, 100, 50),
"There are only 10 spots left");
);
}
For other people trying this out with junit5, @Rule doesn't exist anymore. Correct me if there's a better way, but it seems this is the junit 5 way:
import org.junit.jupiter.api.extension.ExtensionContext;
import org.junit.jupiter.api.extension.TestExecutionExceptionHandler;
class IllegalArgumentExceptionExpectedExtension implements TestExecutionExceptionHandler {
@Override
public void handleTestExecutionException(ExtensionContext extensionContext, Throwable throwable) throws Throwable {
if (throwable instanceof IllegalArgumentException) {
return;
}
throw throwable;
}
}
And use it like this:
import org.junit.jupiter.api.extension.ExtendWith;
class BinTest {
private Bin bin;
@BeforeEach
void setUp() {
bin = new Bin(10);
}
@ExtendWith(IllegalArgumentExceptionExpectedExtension.class)
@Test
void overstockingNotAllowed() {
bin.restock("Fritos", 2600, 100, 50);
}
}
I'm not sure how to test the exception message with an extension.

Muhammad Nagy
944 PointsIn JUnit 5, the implementation should be like that
@Test
void overstockingNotAllowed() {
IllegalArgumentException thrown =
assertThrows(
IllegalArgumentException.class,
() -> {
bin.restock("Fritos", 2600, 100, 50);
});
assertTrue(thrown.getMessage().equals("There are only 10 spots left"));
}