Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial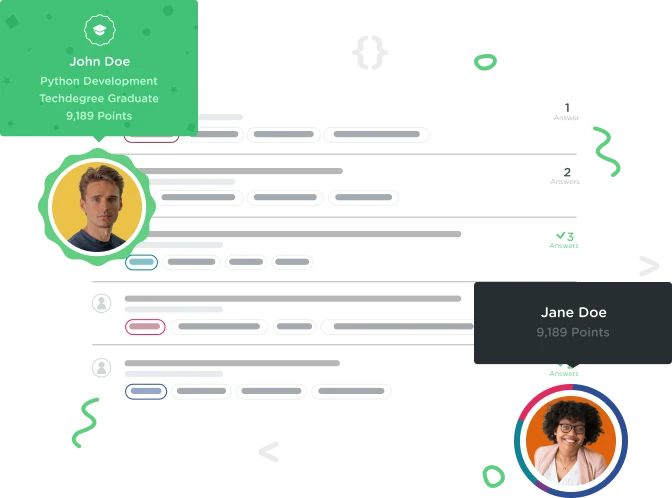
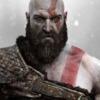
boi
14,242 PointsJavaScript is weird.
Alright. Since I'm coming from a Python background to JavaScript, I guess it's natural to feel a bit bamboozled.
So, basically, the working of the code below is as follows:
It takes a user input (a number) and returns a random number from 1 to the user number. I've included an if
clause to hunt for errors. Now here is the part where it got really weird for me.
When I ran the code, and passed a 0
to the prompt, the if
clause did not execute and it printed out The random number from 1 to 0, is 1
. Ok, so I said to myself "This is because the input is taken as a string which is '0'", and I totally understand that and changed the statement userInput === 0
to userInput == 0
BUT before that, I passed an empty value and if
clause did not catch the error. The else
clause ran the printed out the message The random number from 1 to NaN, is NaN
.
I thought the purpose of the isNaN()
method was to give me True in this case but it seems to act strange when passing an empty or null value. Upon research, what I found was that the isNaN()
method tries to convert the value passed to it into a number, and when an empty or null value is passed to it, it changes it to 0
, and hence the statement isNaN("")
= False. This is just absolutely not well designed (IMO, please don't attack me).
let userInput = prompt("Enter a number please: ");
if ( isNaN(userInput) || userInput === 0 ) {
alert("This input is not valid please try again");
}
else {
userInput = parseFloat(userInput);
const randomNumber = Math.floor(Math.random() * userInput) +1;
document.write(`The random number from 1 to ${userInput}, is ${randomNumber}.`);
console.log(isNaN("1"))
}
I mean, is JavaScript filled with these types of mechanics? It just scares me sometimes to think these mechanics are everywhere in JavaScript. Anyways, I love learning languages and love learning JavaScript, and will continue to learn JavaScript. I just had a small concern (or misunderstanding). I would highly appreciate any criticism, education, or tips from you. Thanks
1 Answer
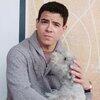
fredyzegdi
Full Stack JavaScript Techdegree Student 12,899 PointsHello: JS treats the exceptions in a very different way than Python does. If something doesn't work in JS, the console of the browser shows you Warnings but the rest of the code still running. In Python if something doesn't work and you if didn't catch the exception, the script crashes. Here, I wrote an alternative solution for your problem:
let userInput = parseInt(prompt("Please type a number [greater than 1]: "));
if(userInput > 1) {
const randomNumber = Math.floor(Math.random() * userInput) +1;
document.querySelector('body').innerHTML = `<h1>The random number from 1 to ${userInput} is ${randomNumber}</h1>`;
} else {
document.querySelector('body').innerHTML = "<h1>That is not a valid number!</h1>";
}
Have a nice day!
Damien Lavizzo
4,223 PointsDamien Lavizzo
4,223 PointsI had the same idea as you to check for validity, and this was my code. I've tested everything I can think of and it seems to function properly: