Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial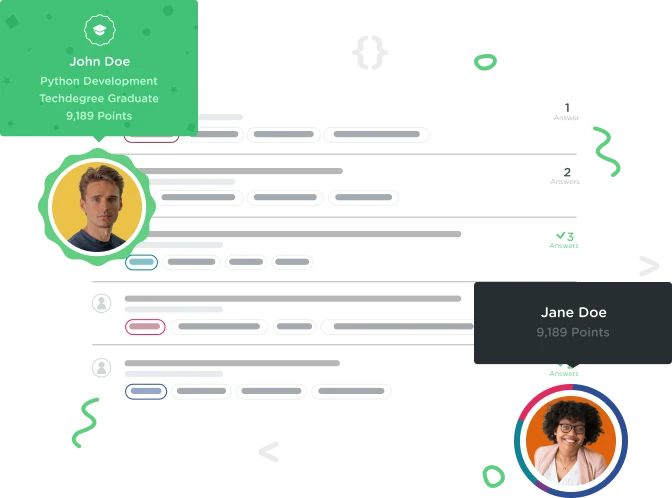

Aaron Banerjee
6,876 Pointsjava serialization code will not compile
When i run the example. java file which is the second file in the three files posted in this question, i get an '<identifier> expected error', can someone please help me understand why
package com.teamtreehouse;
import java.io.Serializable;
import java.util.Date;
public class Treet implements Comparable, Serializable {
private final String mAuthor;
private final String mDescription;
private final Date mDate;
public Treet(String author,String description,Date date){
mAuthor = author;
mDescription = description;
mDate = date;
}
@Override
public String toString(){
return String.format("Treet: \"%s\" by %s on %s", mDescription, mAuthor
,mCreationDate);
}
@Override
public int compareTo(Object obj){
Treet other =(Treet) obj;
if (equals(other)){
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if (dateCmp == 0){
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
public String getAuthor (){
return mAuthor;
}
public String getDescription(){
return mDescription;
}
public Date getDate(){
return mDate;
}
public String[] getWords(){
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}
import java.util.Arrays;
import java.util.Date;
import com.teamtreehouse.Treet;
import com.teamtreehouse.Treets;
public class Example {
public static void main(String[] args){
Treet treet = new Treet(
"CraigDennis","Whats good",new Date(14218499732000L)
);
System.out.printf("This is a new Treet: %s %n",treet);
Treet secondTreet = new Treet("Journey to code","@treehouse makes learning java so fun",new Date());
System.out.println("The words are:");
for (String word:treet.getWords()){
System.out.println(word);
}
}
Treet[] treets = {treet,secondTreet};
Arrays.sort(treets);
for (Treet exampleTreet: treets){
System.out.println(exampleTreet);
}
Treets.save(treets);
Treet[] reloadedTreets = Treets.load();
for (Treet reloaded : reloadedTreets){
System.out.println(reloaded);
}
}
package com.teamtreehouse;
import java.io.*;
public class Treets {
public static void save(Treet[] treets){
try (
FileOutputStream fos = new FileOutputStream("treets.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
) {
oos.writeObject(treets);
} catch(IOException ioe){
System.out.println("Problem saving treets");
ioe.printStackTrace();
}
}
public static Treet[] load(){
Treet[] treets = new Treet[0];
try (
FileInputStream fis = new FileInputStream("treets.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
){
treets = (Treet[]) ois.readObject();
} catch(IOException ioe){
System.out.println("Error reading file");
ioe.printStackTrace();
}catch(ClassNotFoundException cnfe){
System.out.println("Error loading treets");
cnfe.printStackTrace(();
}
return treets;
}
}
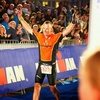
Steve Hunter
57,712 PointsWhich line is throwing the error?
1 Answer

Seth Kroger
56,413 PointsIt looks like you have about half of the code outside the curly brackets for main().
Caleb Kleveter
Treehouse Moderator 37,862 PointsCaleb Kleveter
Treehouse Moderator 37,862 PointsHey Aaron, I really wish I could help, but my Java is really rusty and I wouldn't trust my self to debug it 😄. I hope you find the answer. Happy coding!