Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial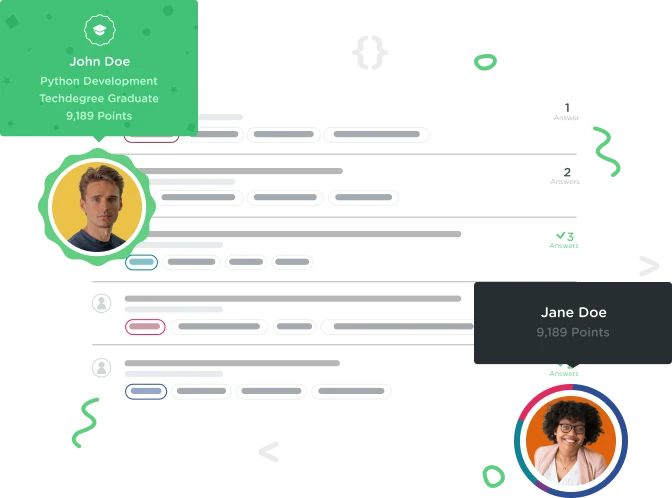

grctniu75rx
6,328 PointsJava methods
The challenge is to "Fix the getLineNumberFor method to return a 1 if the first character of lastName is between A and M or else return 2 if it is between N and Z." I kind of know how to do this in what seems to be an ugly bit of code. But it wants me to do it in this specific way and I just don't know where to start. Any advice is much appreciated
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
7 Answers
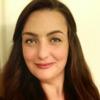
Jennifer Nordell
Treehouse TeacherHi there! Let's try some hints and see if you can get this working. I think you can!
- This can be done with one if / else statement
- If the character at (
charAt
) the 0 index of the string being passed in (lastName
) is less than or equal to 'M' set thelineNumber
equal to 1 - Otherwise (
else
) set thelineNumber
equal to 2 - You can find some examples of the use of
charAt
here
I hope this helps, but let me know if you're still stuck!

chase singhofen
3,811 Pointsi new i needed a if else statement but i put in an if else if statement, and i couldnt figure out how to declare the char. i didnt even think to use that if(lastName.charAt(0) <= 'M')
i also put return 1 & else return 2.
i was actually trying to think of an expression to use for " through" i was trying to declare the whole alphabet. i didnt know the pc would scan the whole alphabet w/o us putting it in the code.
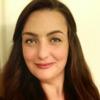
Jennifer Nordell
Treehouse TeacherIt might not be immediately obvious, but all these characters that you see on the screen are actually representations of numbers. It's why we have to specify the "encoding" of a text file. So when you say < 'M'
, you are actually saying if the numerical version of this character is less than the numerical version of the other letter, then do this.
You can view the Oracle documentation here. You might also view the documentation on Unicode.

Mehmet Arabaci
4,432 PointsHi,
So I went to do this challenge and I tried the whole <= M thing but it wouldn't work for me so I ended up with the longest and most tedious code I could write to solve it:
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char firstLetterOfLastName = Character.toLowerCase(lastName.charAt(0));
if (firstLetterOfLastName == 'a') {
lineNumber = 1;
}
if (firstLetterOfLastName == 'b') {
lineNumber =1;
}
if (firstLetterOfLastName == 'c') {
lineNumber =1;
}
if (firstLetterOfLastName == 'd') {
lineNumber =1;
}
if (firstLetterOfLastName == 'e') {
lineNumber =1;
}
if (firstLetterOfLastName == 'f') {
lineNumber =1;
}
if (firstLetterOfLastName == 'g') {
lineNumber =1;
}
if (firstLetterOfLastName == 'h') {
lineNumber =1;
}
if (firstLetterOfLastName == 'i') {
lineNumber =1;
}
if (firstLetterOfLastName == 'j') {
lineNumber =1;
}
if (firstLetterOfLastName == 'k') {
lineNumber =1;
}
if (firstLetterOfLastName == 'l') {
lineNumber =1;
}
if (firstLetterOfLastName == 'm') {
lineNumber =1;
}
if (firstLetterOfLastName == 'n') {
lineNumber =2;
}
if (firstLetterOfLastName == 'o') {
lineNumber =2;
}
if (firstLetterOfLastName == 'p') {
lineNumber =2;
}
if (firstLetterOfLastName == 'q') {
lineNumber =2;
}
if (firstLetterOfLastName == 'r') {
lineNumber =2;
}
if (firstLetterOfLastName == 's') {
lineNumber =2;
}
if (firstLetterOfLastName == 't') {
lineNumber =2;
}
if (firstLetterOfLastName == 'u') {
lineNumber =2;
}
if (firstLetterOfLastName == 'v') {
lineNumber =2;
}
if (firstLetterOfLastName == 'w') {
lineNumber =2;
}
if (firstLetterOfLastName == 'x') {
lineNumber =2;
}
if (firstLetterOfLastName == 'y') {
lineNumber =2;
}
if (firstLetterOfLastName == 'z') {
lineNumber =2;
}
return lineNumber;
}
I'd be curious as to why why I couldn't get code such as below to work
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char firstLetterOfLastName = Character.toLowerCase(lastName.charAt(0));
if (firstLetterOfLastName >= 'a' && <='m'){
lineNumber = 1;
} else{
lineNumber = 2;
}
return lineNumber;
}
Looking at the posts above I can see that <m would have worked but how comes not a range like >= and(&&) <=
Thanks
Mehmet
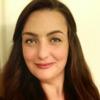
Jennifer Nordell
Treehouse TeacherHi there, Mehmet! Your last piece of code would have worked except that it contained a syntax error. You wrote this:
if (firstLetterOfLastName >= 'a' && <='m')
Java does not assume to know what is supposed to be less than or equal to m
. You must specifically say on both sides of the && what value to look at. So if you had rewritten it as:
if (firstLetterOfLastName >= 'a' && firstLetterOfLastName <='m')
... then that would have passed!

chase singhofen
3,811 Pointsi wish the workspace would show me syntax errors in real time. i keep forgetting these braces and i spend about 30 minutes adding and deleting until is says i passed
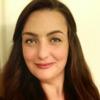
Jennifer Nordell
Treehouse TeacherThat would be great! However, I'm unaware of any Java IDE that does that at all. Generally, the code must be interpreted and compiled before a syntax error/compiler error will be reported. This is true of several languages.

chase singhofen
3,811 Pointsi guess what i meant was :
when i use eclipse or netbeans i get little "squigglies" if it senses something is off. i can hover over it and it will show hints of what might be missing and most of the time i am forgetting braces. other times i will get options to add or change methods or import libraries.
in the workspace it just takes forever for me to figure out what i miss especially if i keep editing my code. the check syntax doesn't help me & the ' ^ ' doesn't explain much when i check.
sometimes the syntax errors will point at a brace when i check so i add or delete one, then come to find out i forgot to declare my int variable
it's just frustrating having to come to the forum for every question when all you need is something minor such as a brace
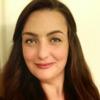
Jennifer Nordell
Treehouse TeacherAh, I get what you mean! Yes, that would be lovely. You might submit that as a suggestion to Treehouse staff. In my experience, they are always glad to get feedback from students. You can reach them at help@teamtreehouse.com

Abed Sujan
16,301 Points[Moderator redacted.]
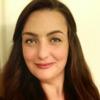
Jennifer Nordell
Treehouse TeacherHi there, Abed Sujan! Your help is appreciated in the Community, but please note that Treehouse highly discourages the practice of posting explicit copy/pastable answers to pass a challenge without any sort of explanation whatsoever. Feel free to repost your answer with an explanation.

Mehmet Arabaci
4,432 Pointsno way! That makes perfect sense now.
Thanks Jennifer
grctniu75rx
6,328 Pointsgrctniu75rx
6,328 PointsThank you, I would rather not ask for what the actual code should be. But, I've been at it for quite some time, and while I know logically what it should be, the syntax itself is something I can't seem to get right. We haven't done something with this particular code yet in the track so I can't find out how by watching the videos. Do you mind showing me the code, so I can figure out how you got from A to B?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHi stormy! Let's take a look then at how I wrote it:
I hope my comments will make this code clear. But we're using
lastName.charAt(0)
to retrieve the first letter of the string passed into the function. In this case, it's the person's last name. Because there are only two options here, either line one or line two, we only need to check if they go into one of the lines otherwise they go into the other line.. So I checked to see if the first letter was less than or equal to M. If that is the case, I set the lineNumber to 1. Otherwise, I set it to 2. At the end we return the lineNumber the person is assigned to. Hope this helps!grctniu75rx
6,328 Pointsgrctniu75rx
6,328 PointsAh thank you! I didn't understand how the proper parenthetical syntax in the line " if(lastName.charAt(0) <= 'M')"
Carlos Salcedo
1,788 PointsCarlos Salcedo
1,788 Pointsexactly what i needed thanks
Tai Do
2,312 PointsTai Do
2,312 PointsThank you!!
Ciro Abril
935 PointsCiro Abril
935 Pointsawesome!! thanks
lap Nguyen
715 Pointslap Nguyen
715 Pointsyup this helped. thx Jennifer for the explanation and stormyseliquini for bringing it up.