Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial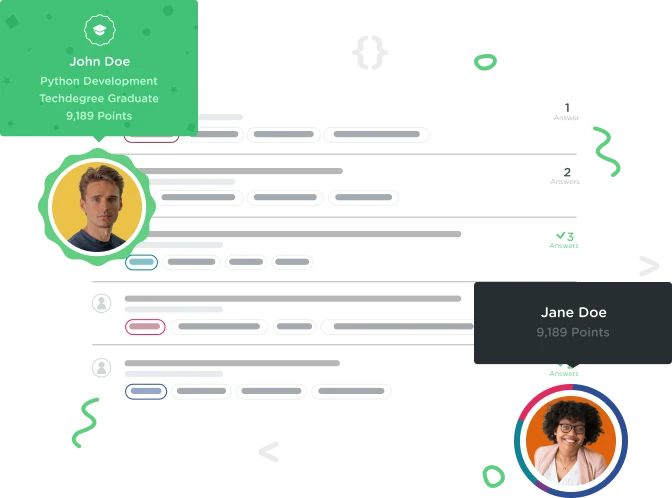
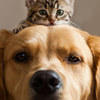
Devin Scheu
66,191 PointsJava Help
Question: The method getTitleFromObject will be called and passed a String and/or a com.example.BlogPost. Return the object type casted as a String if it is a String, and if it is the BlogPost type cast it, and return the results of the getTitle method.
Can someone walk me through step by step of this problem because im really confused, I know it must use the instance of method but everyway I have tried fails
Code:
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this return statement to be the correct string.
return "";
}
}
8 Answers

Ken Alger
Treehouse TeacherDevin;
This particular challenge is indeed a bit of a stretch of our mental Java prowess. Let's take a look..
Task 1
The method getTitleFromObject
will be called and passed a String
and/or a com.example.BlogPost
. Return the object type casted as a String
if it is a String
, and if it is the BlogPost
type cast it, and return the results of the getTitle
method.
I've included BlogPost.java
for your reference only.
How do we get through all that? We need to check if our object is either an instance of String
or a BlogPost
, right? We could start with:
if (obj instanceof String) {
// do something here
}
if (obj instanceof BlogPost) {
// do something similar but different here;
}
There is a good skeleton of what we need to do, we just need to add in a bit and do some casting for our obj
and assign it to a String
which is what the method returns.
String result = ""; // Create a String variable to store our results for us to return
if (obj instanceof String) {
result = (String) obj; // Cast our obj to a String, which we can return directly.
}
if (obj instanceof BlogPost) {
BlogPost bp = (BlogPost) obj; // Cast our obj to a BlogPost, we should be able to get our title from there.
result = bp.getTitle(); // Yippie! We can return that because result is a String and getTitle() returns a String
}
return result; // return the String of our Object's title
Does that help at all? Please post back if you are still stuck, confused, or have further questions. For myself casting is still confusing at times, so don't feel bad that you are stuck here.
Happy coding,
Ken

Cary Oldenkott
8,178 PointsKen, your syntax for "instanceof" has a capital O, which is causing errors. Just in case nobody caught that.

Ken Alger
Treehouse TeacherCary;
Doh! Thanks for pointing that out. I just updated my post.
Ken
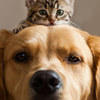
Devin Scheu
66,191 PointsThank you everyone for the help, I understand now.

Ben Wong
19,426 Pointsanymore hints please:)

Natenda Manyau
3,005 Pointshi. am really stuck. I've done all that is stated ABOVE BUT AM GETTING MASSIVE ERRORS
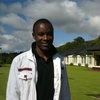
Admire Dhodho
5,411 Pointspost your code

jordanbruner
10,055 PointsI posted the following code based on the help described above but it's still returning an error: ' Bummer! I expected the String value I passed in to be returned and it wasn't. Received ''' When I go to Preview, nothing shows up in output. What could be going wrong here? Please help.
public String getTitleFromObject(Object obj) { String result = "";
if (obj instanceof String) {
result = (String) obj;
}
if (obj instanceof BlogPost) {
result = ((BlogPost) obj).getTitle();
}
return result;
}

Ken Alger
Treehouse TeacherJordan Bruner, your code seems to pass for me.
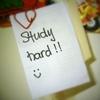
Quinton Rivera
5,177 PointsI cant get this code to work for me either, I keep getting complier errors ./TypeCastChecker.java:22: error: reached end of file while parsing return result;} ^ 1 error
public static String getTitleFromObject(Object obj) {
String result = "";
//check if it is instance of String if yes cast to string and assign to result
if(obj instanceof String){
result = (String)obj;}
//check if it is instance of obj if yes creat a blogpost object then cast obj to blogpost then assign to result
if(obj instanceof BlogPost){
result = ((BlogPost)obj).getTitle();}
return result;}

Ken Alger
Treehouse TeacherYour code works for me just fine. Make sure you have all of your closing curly brackets in place as that error message seems to indicate an issue along those lines.
Happy coding, Ken

Bryn Clegg
4,698 PointsI had problems with this and found that my code was correct, however, I had left one of these set to true which gave me errors. Setting it back to how it is below sorted the problem.
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsGreat answer Ken.
In the second
if
block, testing forinstanceof BlogPost
, we don't need a new variable. Craig briefly shows this in the video, but it does not get explained. To call a method on an object being cast, we need two sets of parens.result = ((BlogPost) obj).getTitle();
If we can be reasonably sure that the argument being passed in will either be of type BlogPost or String, we can compact the body of this method down to a single line.
Cheers
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherRobert;
You are indeed correct and for many folks the utilization of ternary statements is more readable. For some starting out, however, it can be confusing, which is one of the reasons I posted the above version.
It is great that now folks can see how to do the same thing with two different methods of coding.
Happy coding,
Ken
Broderic Crowe
1,549 PointsBroderic Crowe
1,549 PointsYou're a miracle worker Ken! I was relieved to finally find a comprehensive answer to this difficult challenge for my little java brain to understand. As opposed to the half-dozen other answers that were just vague and unhelpful. You broke it down and explained it excellently:) Thanks
Kevin Michie
6,554 PointsKevin Michie
6,554 PointsFantastic walk-through!
kristoffer tølbøll
1,337 Pointskristoffer tølbøll
1,337 PointsHow come you add to if-statements and not an if/else statement????