Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial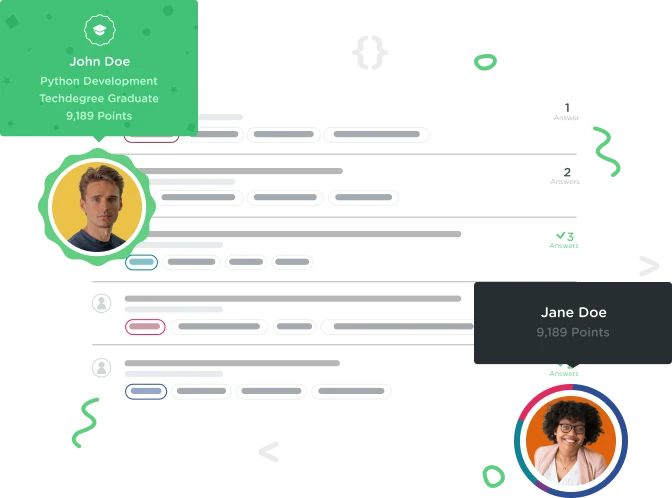

Jacob Davis
Courses Plus Student 2,965 PointsJava Finishing Tree Story
I have the tree story working in my IDE. I think the problem has something to do with catching null values when the user is prompted to fill in the phrases, but when you just hit enter an empty string is passed through, so I do not get any errors when I tried that. Basically I need guidance on how to pass the psuedo tests. I apprecieate any help.
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
// write your code here
System.out.printf("Please enter a story and put double underscores before and after the words " +
"you want replaced.%n");
BufferedReader storyInput = new BufferedReader(new InputStreamReader(System.in));
String story = null;
try {
story = storyInput.readLine();
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
}
Template tmpl = new Template(story);
Prompter prompt = new Prompter();
prompt.run(tmpl);
}
}
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) {
String word = null;
do {
System.out.printf("Please enter a(n) %s%n", phrase);
mReader = new BufferedReader(new InputStreamReader(System.in));
try {
word = mReader.readLine();
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
}
if (mCensoredWords.contains(word)) {
System.out.printf("The word %s is censored, please enter a valid %s%n", word, phrase);
}
} while (mCensoredWords.contains(word));
return word;
}
}
3 Answers

Jacob Davis
Courses Plus Student 2,965 PointsHere is what I have
public String promptForWord(String phrase) throws IOException, NullPointerException {
String word = "";
do {
System.out.printf("Please enter a(n) %s%n", phrase);
mReader = new BufferedReader(new InputStreamReader(System.in));
try { word = mReader.readLine();
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
}
catch (NullPointerException n) {
System.out.println("Null is not accepted");
n.printStackTrace();
}
if (mCensoredWords.contains(word)) {
System.out.printf("The word %s is censored, please enter a valid %s%n", word, phrase);
}
else if (word.isEmpty()) {
System.out.printf("You did not enter a(n) %s, please try again.%n", phrase);
}
} while (mCensoredWords.contains(word) || word.isEmpty());
return word;
}

Todd Anderson
4,260 PointsI am sorry we haven't figured this out. Is the error in your IDE or in the treehouse workspace?
When I pasted your code into the workspace the error I got was that the story hadn't been presented (to do #3), but no errors other than that. I did notice that you are trying to append words to lists that are set to null which I'm not sure you meant to do. Just like you did the empty string, you can just create a new list (List<String> words = new ArrayList();) to append the words to. That might help. If this doesn't solve your problem I will delete my answers so that more experienced coders will look at your post.

Jacob Davis
Courses Plus Student 2,965 PointsThe error is in the treehouse workspace. Everything works fine in the ide. No worries I appreciate all the help you've given it has helped me think about things in different ways. Don't worry about deleting your answers I have contacted support and they will help after the discussion has been up for 48 hours.

Jacob Davis
Courses Plus Student 2,965 PointsThis is my updated code.
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = new ArrayList<String>();
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = new ArrayList<String>();
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) {
String word = "";
do {
System.out.printf("Please enter a(n) %s%n", phrase);
mReader = new BufferedReader(new InputStreamReader(System.in));
try { word = mReader.readLine();
if (word == null) {
throw new IOException();
}
} catch (IOException e) {
System.out.println("Entering null values is not allowed.");
e.printStackTrace();
}
if (mCensoredWords.contains(word)) {
System.out.printf("The word %s is censored, please enter a valid %s%n", word, phrase);
}
} while (mCensoredWords.contains(word));
return word;
} }

Jacob Davis
Courses Plus Student 2,965 PointsI have changed all the lists that were set to null to new array lists. Also, in the promptForWord method in the try block I throw a new exception to catch if a null is entered which is then caught. The error I get in the tree house workspace is I didn't present the tree story. And this is what the preview page looks like.
java.io.IOException
at com.teamtreehouse.Prompter.promptForWord(Prompter.java:77)
at com.teamtreehouse.Prompter.promptForWords(Prompter.java:57)
at com.teamtreehouse.Prompter.run(Prompter.java:38)
at com.teamtreehouse.Main.main(Main.java:25)
at JavaTester.run(JavaTester.java:77)
at JavaTester.main(JavaTester.java:39)
java.io.IOException
at com.teamtreehouse.Prompter.promptForWord(Prompter.java:77)
at com.teamtreehouse.Prompter.promptForWords(Prompter.java:57)
at com.teamtreehouse.Prompter.run(Prompter.java:38)
at com.teamtreehouse.Main.main(Main.java:25)
at JavaTester.run(JavaTester.java:77)
at JavaTester.main(JavaTester.java:39)
Please enter a story and put double underscores before and after the words you want replaced.
Please enter a(n) first prompt
Entering null values is not allowed.
Please enter a(n) second prompt
Entering null values is not allowed.
Testing null and then null
Jacob Davis
Courses Plus Student 2,965 PointsJacob Davis
Courses Plus Student 2,965 PointsI know this doesn't work because isEmpty() cannot be used on a variable that is a null. If I check for null and send it back to the loop a never ending loop occurs and if I let the null pass then it says I did not present the tree story. So I'm not sure what else to try.