Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial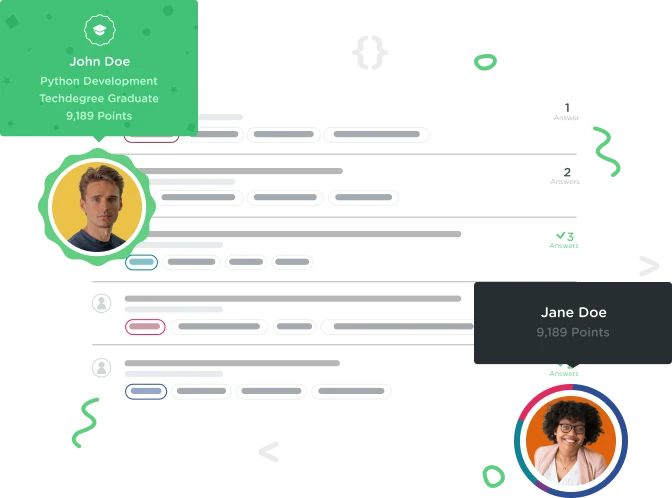

james white
78,399 PointsJava Data Structures - Using a map to store contact methods challenge
This is a three part challenge.
Link to challenge:
It starts out with:
Challenge Task 1 of 3
In the next couple of steps, let's fix up this work in progress Contact model. This is going to be used in a Web application where the user can add different ways to contact a person.
In this first step, modify the addContactMethod method to store the information in the mContactMethods Map.
The code you are given is for starters is:
public void addContactMethod(String method, String value) {
// TODO: Add to the contact method map
}
So naturally if you are told to 'Add' you use add:
public void addContactMethod(String method, String value) {
// TODO: Add to the contact method map
mContactMethods.add(method, value);
}
Wrong!
So you go looking at the video and the code in the zip downloads for the course.
Here's why you won't find the answer in either place.
Both the SongBook.java code in the video and the SongBook.java code in the zip download use Array lists (not HashMaps).
Totally bogus!
By the way - hashmaps are not some great new invention by the guys/gals at TeamTreehouse.
Way back in the early versions of Windows (before the Registry), there were something called ".ini files" (where "ini" was short for initialization I guess).
They used not only keys and values but had 'sections' as well.
I imagine using hashmaps/hashtables was around even before then even though Wikipedia doesn't give much history on them:
http://en.wikipedia.org/wiki/Hash_table
It does say:
In computing, a hash table (hash map) is a data structure used to implement an associative array, a structure that can map keys to values.
Yes, you can use the term "associative arrays" to confound and impress your non-programmer friends.
.
Thus hashmaps are pretty common knowledge in the programming world and I can go 'aroamin' on the internet to try and find some info on the Java implementation of the use of hashmaps.
Specifically I ended up relying on StackOverFlow to supply the information missing from the course content,
as per usual, the alternate source for code documentation whenever
there is a "disconnect" between what is presented in the Treehouse course videos
and what the particular challenge question is asking about.
.
Here's the StackOverFlow thread I (eventually) found:
http://stackoverflow.com/questions/11048783/java-hashmap-key-value-storage-and-retrieval
Notice the 'hm.put' statements for entering keys/values into the hashmap.
So replace '.add' with '.put' in the above code snippet and you should be good to go
(but of course you had to go outside TeamTreehouse to find that out)
Does the "bogus-ness" (bogosity?) end there?
Of course not..here's part 2 of the challenge:
Challenge Task 2 of 3
In this task, let's fix the getAvailableContactMethods method. We want to return a set of the currently defined contact methods.
HINT: How about a set of the keys from mContactMethods?
I know what you are thinking:
They actually gave us a HINT, so this one should be a slam-dunk, right?
Wrong!
Of course you immediately try:
public Set<String> getAvailableContactMethods() {
// FIXME: This should return the current contact method names.
Set names = mContactMethods();
return names;
}
Such code tries to follow the FIXME instruction to the letter
(even throwing in a 'set' like the HINT mentioned).
Of course you get a "Bummer!" message
How foolish of you to believe that a HINT would actually give you all the information you need!
So where do we go to get the missing piece of code information?
You guessed it - StackOverFlow:
http://stackoverflow.com/questions/8909810/how-to-print-all-key-and-values-from-hashmap-in-android
See the line of code that says:
Set keys = map.keySet();
There's your real hint '.keySet()' is the missing piece of info you need to figure out what code the challenge wants:
public Set<String> getAvailableContactMethods() {
// FIXME: This should return the current contact method names.
Set keys = mContactMethods.keySet();
return keys;
}
Note: I ended up using 'keys' (in the code snippet above) for the variable instead of 'names' just because :
1.) It seemed better suited to using hashmap's terminology
2.) The HINT (although not quite "hint-ful" enough) did actually mention 'keys'
Yes, (disclaimer for those code purists out there) it could have been done in one line instead of two.
Onward...
Challenge Task 3 of 3
For this task, let's fix the getContactInfo method.
That seems like a short and easy thing to fix...let's look at what they are giving us for starter code:
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
return null;
}
So reading the comment, the answer is clearly:
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
return methodName;
}
Having been "twice bitten" you are naturally a little "gun shy"
(even if it seems totally obvious).
With great trepidation (and a little hesitation) you press the "Check Work" button
Bummer!
AAAArrrrrggghh!
Even though you know its useless you re-watch the video and look at the code in the project's zip download one more time.
Inside SongBook.java you do find something that looks promising in these lines:
Map<String, List<Song>> byArtist = new HashMap<>();
for (Song song : mSongs) {
List<Song> artistSongs = byArtist.get(song.getArtist());
Hmmm..there's actually something that uses '.get' in connection with a new HashMap.
Could this be real?
Are you actually "getting" a break (bad pun intended)
Quick check on StackOverFlow to confirm:
http://stackoverflow.com/questions/1789679/get-string-value-from-hashmap-depending-on-key-name
Yes!
'mContactMethods', because it uses a HashMap, has a built-in 'mContactMethods.get()' method that you probably can pass in the 'methodName' parameter.
So this challenge forced me to research how to work with HashMaps in Java even though it was never actually fully explored in the Java Data Structures course.
Hope reading through all this helps someone understand a little more what the challenge is looking for..
All of which leaves me thinking...maybe, instead of spending all that time producing videos they should just say:
Here's your course topics --now go explore the whole internet, and when you are ready to take the challenges come back and will have some doozies for you to puzzle out.
Then all the points can be assigned to just solving the challenge puzzles instead of endless hours watching confusing videos that don't explain things fully.
Yow!
Reading that over I sound really whine-y and cynical.
Must be tired --time for bed.
Good night all.
ZZZZZZZZZZZZzzzzzzzzzzzzzzzzzz
10 Answers

Craig Dennis
Treehouse TeacherJust wondering....Did you watch the videos or just attempt the challenges?
Watch the Maps video and then read this post again afterwards.
I am truly sorry that you feel like you need to look outside of the information i delivered to you in the course. However, you can be certain that I would never assess your knowledge on something I didn't teach, what would be the point?

Edane Barton
11,457 PointsThe answers can all be found in the previous video 'Exploring the Java Collection Framework'-> Maps.
Rather than 'add' try 'put'ing the correct value :)
2 of 3 you wrote
public Set<String> getAvailableContactMethods(){
// FIXME: This should return the current contact method names.
Set names = mContactMethods();
return names;
}
what I find useful is that the method will tell you what it is expecting to return. In this case, it is expecting you to return a 'String', if they were expecting you to return a number, you would have seen
public Set (Integer).......
With that in mind, the String you would create is called
Set <String>
In the maps video look at 5:56, you will get your answer there.
3/3
You wrote that the answer was
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
return methodName;
}
An analogy that I would like to use here is that if your mom ask you for your brother's/sister's phone number and you answer back by shouting out their name.
In this case you should create another variable and use the 'get' method with the methodName.
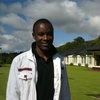
Admire Dhodho
5,411 PointsThe videos are not useful to him but as for me it is the right tool that made me find programming interesting. Thanks for the videos teamtreehouse
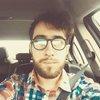
George Siaskov
1,292 PointsJust wondering....Did you watch the videos or just attempt the challenges
just attempt the challenges :)))
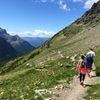
Stephen Wall
Courses Plus Student 27,294 PointsWow haha. Your videos are great Craig! As someone who has a lot of respect and pride for this site, as it is 100% responsible for the job I now hold, in addition to all other doors it has opened for me, here's a bit of advice to my fellow treehouse members: Don't be afraid to go outside of treehouse to look for answers! Google is your friend. Documentation is your friend. I am on stackoverflow ALLLL the time for work and there is nothing wrong with that. Rewatch the videos if you aren't understanding something, chances are you just missed something in the video as you were watching and coding along. Happens to me all the time. Finally don't be afraid to search the forums for answers and RESPECTFULLY ask for help. The staff at treehouse are incredibly friendly and only want you to succeed. Thanks again treehouse! Much Love!
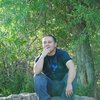
Brody Ricketts
15,612 PointsAfter several hours of going through the challenges and trying to understand, I decided to add some comments to help those still struggling with this challenge. I omitted all of my correct answers, and I will continue to help where I can.
package com.example.model;
import java.util.Map;
import java.util.Set;
import java.util.HashMap;
public class Contact {
private String mFirstName;
private String mLastName;
private Map<String, String> mContactMethods;
public Contact(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
/* This stores contact methods by name
* e.g.: "phone" => "(555) 555-1234"
*/
mContactMethods = new HashMap<String, String>();
}
// Task 1 "In this first step, modify the addContactMethod method to store the information in the mContactMethods Map."
public void addContactMethod(String method, String value) {
/*
* TODO: Add to the contact method map
*
* The task at hand is to put something into the HashMap defined on line 10, and line 18.
* We know that the method to add anything to a HashMap is .put();
* Ultimately it will look much like mMapName.put(K, V);
* Lastly, for this task, remember that the method is tagged with void.
* That means nothing will be returned, and no return statement is needed.
* Maps 2:40
*/
}
/* Task 2 "In this task, let's fix the getAvailableContactMethods method.
* We want to return a set of the currently defined contact method."
*/ "HINT: How about a set of the keys from mContactMethods?"
public Set<String> getAvailableContactMethods() {
/*
* FIXME: This should return the current contact method names.
*
* The task here is to show the current available contact method names.
* Remember when calling a key set of a HashMap we use the method .keySet();
* (Refer to the wording of the hint Craig gave us.)
* We know that the syntax for calling a key set will look like mMapName.keySet();
* This will only pull the keys, it will not pull their values as well.
* Finally, remember to check for the Map name on line 10.
* Maps 5:23
*/
return null;
}
// Task 3 "For this task, let's fix getContactInfo method."
public String getContactInfo(String methodName) {
/*
* FIXME: return the value for the passed in *methodName*
*
* The task here is the pull the value from a stored key(contact method).
* Remember when pulling from a HashMap we can use the method .get();
* We know that the name of the HashMap is mContactMethods(check line 10 and 18)
* We also know that the syntax looks much like mMapName.get();
* Maps 4:05
*
* A simple analogy that I hope helps you complete this task:
* You have a bowl from fruit on a table(mFruitBowl), you want to GET a piece of fruit(getFruit();).
* You decide to GET an apple. Apple has become your parameter,
* Remember you have to pass apple into mFruitBowl.get();, because that is where its stored, much like a HashMap.
*/
return null;
}

Craig Dennis
Treehouse TeacherNice write up! Thanks Brody!
Takudzwa Karisambudzi
7,157 PointsThat really helped thanx brody
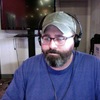
James Lambert
Courses Plus Student 14,477 PointsYep. Thanks.
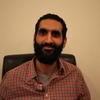
omid nassir
8,771 PointsThat was very helpful, thank you!

Khaleel Yusuf
15,208 PointsI don't understand. I need the code.

Andre Colares
5,437 PointsIn challenge 3 of 3, what is wrong with my code:
public String getContactInfo(String methodName) {
mContactMethods.get(methodName);
return methodName;
}

Andre Colares
5,437 PointsFinally I could understand. Here is the correct code :
public String getContactInfo(String methodName) {
String values = mContactMethods.get(methodName);
return values;
}
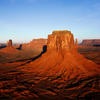
Tim Strand
22,458 Pointsdont need the last part just do: return mContactMethods.get(methodName);
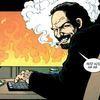
Greg Kitchin
31,522 PointsIt's a good idea to explore the Teachers Notes links as well.

Phillip Hurst
4,204 PointsGood to know I am not alone in that I very often find the Challenges very confusing. Few of them seem to fall in line with the directly previous video and I can succeed by using the things I just learned. More often I find myself googling the text of the challenge title and seeing how other people did it.

Andre Colares
5,437 PointsPhillip Hurst, I agree with you. In this course I felt this too. But what I can say to you is that is good that we learn to find answers in google/blogs/etc!

Craig Dennis
Treehouse TeacherI specifically spaced out the information you learned from earlier in the course to make sure you can access all the information you are learning when you need it.
There is a method to the madness ;)

Luis Santos
3,566 PointsMy sentiments exactly, I ask myself how am I suppose to learn this stuff when I keep googling for answers?

Raja Raja
142 PointsChallenge 2 answer:
public Set<String> getAvailableContactMethods() {
Set keys = mContactMethods.keySet();
return keys;
}

Nino Ross
8,264 PointsThanks so much for this post. I was getting frustrated that I had to keep referencing outside sources just to get hints to solving these problems. I genuinely don't want the answers flat out but a better explanation of the topics discussed and maybe better hints would be greatly appreciated. I sometimes find that the questions can often be far too vague / broad.
I was thrown for a loop when the challenge and the corresponding video required completely different methods. I wasn't even exactly sure where to start looking. I'm still new to all of this but I feel like I would get a better grasp of everything that's going on if I spent these hours in my textbook rather than watching videos that are great but don't explain 100% why we need to do what we're doing in the lectures.

Raja Raja
142 PointsChallenge 1 Answer:
public void addContactMethod(String method, String value) { // TODO: Add to the contact method map mContactMethods.put(method, value); }

none non
246 PointsFor 3rd challenge:
//passing the key to look for in mContactMethods,then save in temp, return temp; -----------------------------------------------------------------------------------;
public String getContactInfo(String contac) {
String temp=mContactMethods.get(contac);
return temp; }
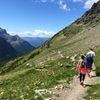
Stephen Wall
Courses Plus Student 27,294 Pointsreturn mContactMethods.keySet();
Andrew Winkler
37,739 PointsAndrew Winkler
37,739 PointsI love your posts and workflow. Please keep commenting. I am learning so much better and my self esteem is regaining it's composure as result of reading about your similar struggles with these lessons. Thank you!