Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial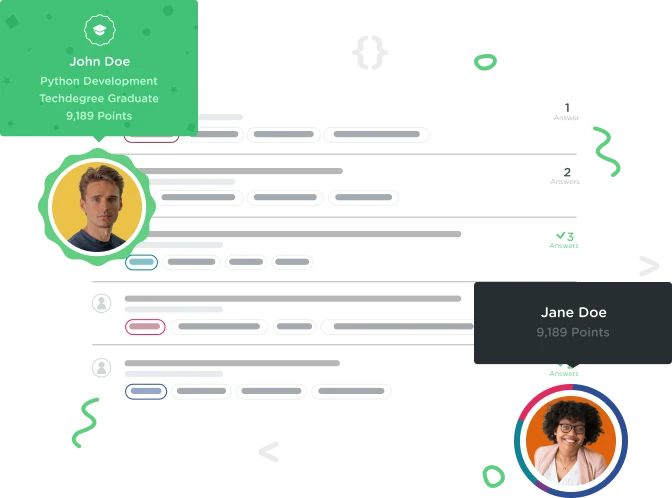

Brendan Milton
9,050 PointsJava Data Structures
Really Struggling with the sets question to loop through and create a set list.
Im trying to figure out the layout of the "getAllAuthors" method, but its leaving me so confused.... I think im on the right track
public List<BlogPost> getAllAuthors() { Set<BlogPost> allAuthors = new Set<BlogPost>(); for(BlogPost mAuthor : getPosts){ allAuthors.addAll(mAuthor.getAllAuthors()); } System.out.printf("Authors: %s %n", allAuthors); } }
any ideas?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public List<BlogPost> getAllAuthors() {
Set<BlogPost> allAuthors = new Set<BlogPost>();
for(BlogPost mAuthor : getPosts){
allAuthors.addAll(mAuthor.getAllAuthors());
}
System.out.printf("Authors: %s %n", allAuthors);
}
}
5 Answers
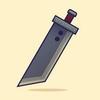
Allan Clark
10,810 PointsThe getAllAuthors method is looking to return a Set of just the authors in a BlogPost. So we will need to change the return statement so that it returns a Set of Strings.
Unfortunately the addAll() method will not work for this example. What that method does is take in a collection (i.e. Set or List or whatever) and adds EVERYTHING to the collection it was called on.
setA.addAll(setB);
This takes each element of setB and adds it to setA. Unfortunately we don't want ALL of each BlogPost, we only want to add each author and add it to the new Set. You would use that for each loop to go through each post, grab the author (with post.getAuthor() ) and add each one to the new set.
// 'm' before a variable indicates a member (aka. class) variable by convention
// so we will want to make up a new name, more times than not this will be just
// a shortened and/or lower case version of the class name for readability
for(BlogPost post: mPosts) {
allAuthors.add(post.getAuthor());
}
P.S. Don't forget to import TreeSet, it isn't included in the Set import. We use TreeSet here to satisfy the alphabetical requirement.

James Simshaw
28,738 PointsHello,
Currently you have:
public List<BlogPost> getAllAuthors() {
Set<BlogPost> allAuthors = new Set<BlogPost>();
for(BlogPost mAuthor : getPosts){
allAuthors.addAll(mAuthor.getAllAuthors());
}
System.out.printf("Authors: %s %n", allAuthors);
}
You appear to be creating a Set of Blogposts, however, in the BlogPost class, authors are stored as a String, so you would want to have a Set of Strings. You also cannot create a new Set because that is an interface. You will have to create a new class that implements the Set interface, like TreeSet or HashSet. Since you are creating a Set(and rightfully so) here, you would want to have the return type for the function be a Set instead of a List(again, a set containing Strings, since that is the type of the author variable).
For your for loop, getPosts is a function and so you need to call it as getPosts(). I also wouldn't recommend naming the BlogPost variable mAuthor. I'd call it something like post. Also, in your allAuthors.addAll line, you want to call the BlogPost.getAuthor() method and so instead of using addAll(), just use the add() function to add a single element to your set.
Finally, instead of printing out the set, you just want to return it.
I hope this helps. Let me know if you are having any futher issues.

Brendan Milton
9,050 PointsYou helped me figure it out!
your method just has to return
"allAuthors"

ISAIAH S
1,409 PointsWhat do you mean?

Brendan Milton
9,050 PointsThanks so much for your help guys, I feel like im really close to understanding it.
this is my code now, down to one error
public List<String> getAllAuthors() { Set<String> allAuthors = new Set<String>(); for(BlogPost post : getPosts()){ allAuthors.add(post.getAuthor()); } return getAllAuthors(); }
it says "Bummer! Per best practices, you should be returning the Set interface. You returned a java.util.List<java.lang.String>
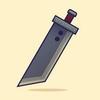
Allan Clark
10,810 PointsThat error is about the return type in your method signature. public List[String] should be public Set[String] (the greater than and less than should replace those brackets because they won't display here). Related to that is your return statement. It is trying to return a method, you want to return the allAuthors Set that was just made.
Last thing I see is
Set allAuthors = new Set();
Set is an interface so it can't be on the right side of the =. Instead we want to use a class that implements Set, specifically for this assignment TreeSet for alphabetical order. Don't forget to import TreeSet.

ISAIAH S
1,409 PointsHi Allan Clark, this is my code:
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set allAuthors = new TreeSet<String>();
for(BlogPost post : getPosts()){
allAuthors.add(post.getAuthor());
} return getAllAuthors();
}
}
It says:
'Bummer! Uh oh. java.lang.reflect.InvocationTargetException'.

Brendan Milton
9,050 PointsThanks for the responses! I'll keep trying