Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial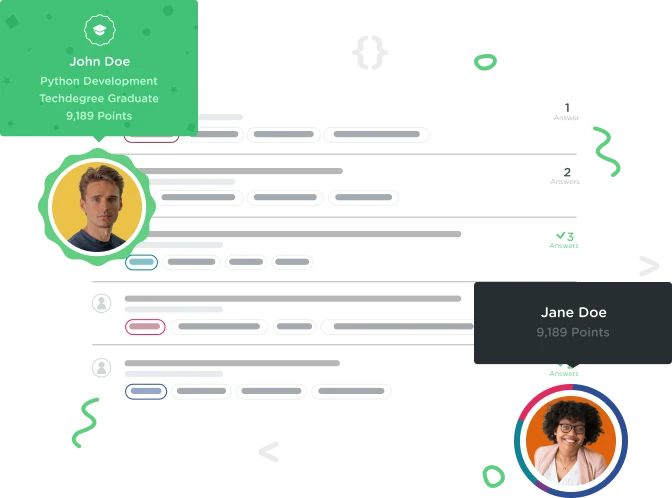

Carl South
Courses Plus Student 866 PointsJava Basics Challenge question for normalizeDiscountCode
I'm sure I am making this more difficult than it needs to be (story of my life) but I cannot figure out the question below.
In the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used, throw a IllegalArgumentException with the message Invalid discount code.
Below is my code where I tried to create a charcter array of the string discountCode then loop through checking to see if they were characters. Clearly its wrong. I don't even know how to check for '$' yet.
private String normalizeDiscountCode(String discountCode){
for (char c : discountCode.toCharArray()){
if (!discountCode.contains(c)){
throw new IllegalArgumentException("Invalid discount code");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
Thanks for your help!! Carl
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
for (char c : discountCode.toCharArray()){
if (!discountCode.contains(c)){
throw new IllegalArgumentException("Invalid discount code");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

andren
28,558 PointsYour answer is actually far closer to the solution than you seem to think. The condition of your if
statement is incorrect, but if you figured out the right condition then your code would work. Looping though the discountCode
as a char array is very much the intended way of solving this challenge.
I'll first give you some hints that might help you out, if you can't solve it after that then just post a comment and I'll provide the solution to you. Your code is close enough that I think you should give it another try though.
There is a method on the
Character
class calledisLetter
which comes quite in handy when solving this challenge. It is called like thisCharacter.isLetter(x)
wherex
is the character you want to verify is a letter. The method returnstrue
if thechar
is a letter, andfalse
if it is not.You need to check two things within your if statement, so you should have two conditions. If you are checking if the char is not a letter and not
$
then both of the conditions should betrue
for theif
statement to run. So the && operator should be used.Checking if the letter is not a
$
is far simpler than you probably think, try to think of the simplest imaginable way of checking if one thing is not equal to another, and then use that.

Carl South
Courses Plus Student 866 PointsYour hints definitely helped and I got it to work! Thanks very much Andren!
for (char c : discountCode.toCharArray()){
if (!Character.isLetter(c) && c != '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}

andren
28,558 PointsGood to hear. Your code was already pretty close to complete, and this is a challenge a lot of people struggle with. Usually even more so than you did, so I'm glad you managed to find the solution.

Khaleel Yusuf
15,208 PointsI need the solution

andren
28,558 PointsKhaleel Yusuf: If you combine Carl's original code and the code he posted after my hints then you get this:
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
for (char c : discountCode.toCharArray()){
if (!Character.isLetter(c) && c != '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
Which is the complete solution to the task.
Benjamin Orimoloye
23,328 PointsBenjamin Orimoloye
23,328 PointsHi Carl, I think you are overthinking the solution. Discount codes are usually a set of alphabets like "DISCNTTODAT". What you are trying to do is change this string to uppercase in case the user enters "discnttoday". All you need to do in your function is :
To check for match, you use the matches() function with a regular expressions pattern like this :
Hope this helps