Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial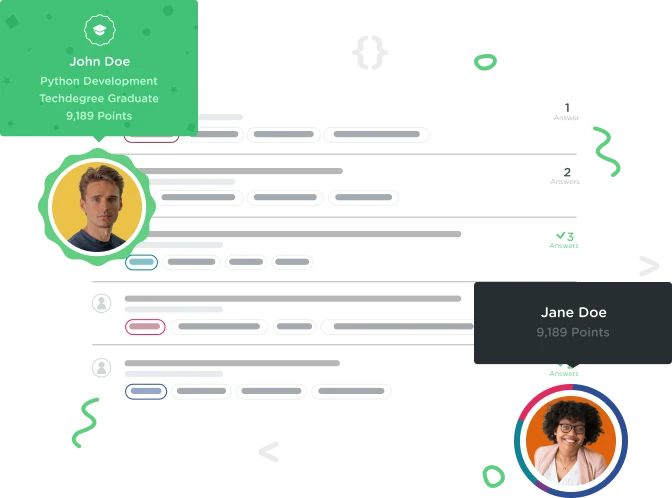

Carlo Antonio Bilbao
24,113 PointsJava, ArrayList Challenge
Not sure how to fix the compile error. Code looks good to me.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Date;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
private List<String> getExternalLinks("http") {
List<String> results = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
results.add(word);
}
}
return results;
}
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
4 Answers

Craig Dennis
Treehouse Teacherprivate List<String> getExternalLinks("http") {
There is something wrong with this line....looks like you are passing a value into the method declaration.
That help?

Marcus Karlsson
1,291 PointsIf you fix that error it complains about missing to implement the getExternalLinks method? Why?

Craig Dennis
Treehouse TeacherHow did you fix it?

Marcus Karlsson
1,291 PointsYou have to define what kind of datatype getExternalLinks takes in as a paramameter. While writing "http" it will of course know it's a string but writing it again in word.startsWith("http") it won't be able to know that u are refering to the "http" in the parameter field.
Since u will call the method getExternalLinks when u want to check a string in your text u have to write getExternalLinks(String text) since u wanna receive a string. Then later in your word.startWith u need to refer to the parameter u are taking in which is word.startWith(text).
Also as craig said u are passing a value of a string not the datatype String that u wanna pass in ur method

Michael Kroon
16,255 PointsHere's the answer that allowed me to pass.
public List<String> getExternalLinks () {
List<String> links = new ArrayList<String>();
for (String link : getWords()) {
if (link.startsWith("http")) {
links.add(link);
}
}
return links;
}
Part of the problem is that the method has been set to private. If it's private then it can only be accessed from within the class. The other problem is that there's a parameter being passed into it and not being used. We already know that the method should retrieve everything starting with "http" so we can use that within the method at 'link.startsWith("http")' and not have to pass a param at all.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherWhat's the compilation error?