Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial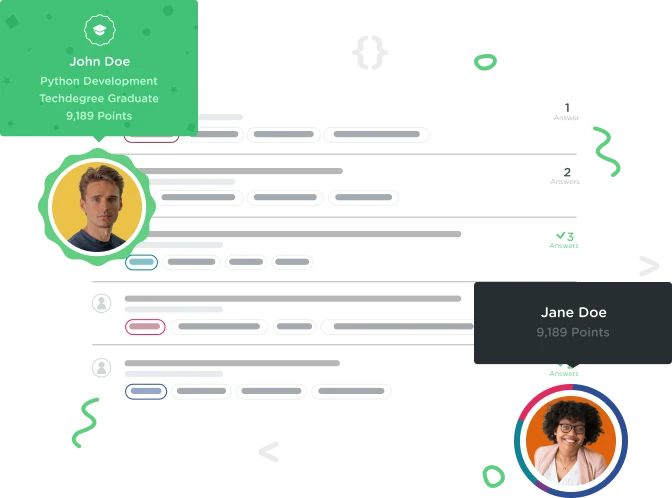

Philippe Pang
3,545 PointsIt says it can't find a symbol when I try to create a HashMap?
It says it can't find a symbol when I try to create a HashMap? It also says post.getCategory is invalid even though it clearly is a method of the BlogPost object declared in the outer for loop.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
public class Blog {
List<BlogPost> mPosts;
public Map<String, Integer> getCategoryCounts(){
Map<String, Integer> categoryCounts = new HashMap<String, Integer>();
for(BlogPost post: mPosts){
for(String category: post.getCategory()){
Integer counts = categoryCounts.get(category);
if(counts == null){
counts = 0;
}
counts++;
categoryCounts.put(category,counts);
}
}
}
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
Mod Edit: Fixed grammar.

Philippe Pang
3,545 PointsSo thats fixed. What about the part where i cant use post.getCategory()?
2 Answers
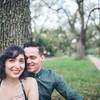
Christopher Earle
Courses Plus Student 22,340 PointsHi Phillippe,
Check what's going on in each of your for loops. In the outer loop, you loop through each BlogPost in mPosts, which is a list of blog posts. In the inner loop, you're code "loops" through each String in post.getCategory(), but that method only returns a single string.

Craig Dennis
Treehouse TeacherDid that work Phillipe?

Craig Dennis
Treehouse TeacherDid that work Phillipe?

Philippe Pang
3,545 PointsUmm i think im pretty confused on the nested for statement part for this piece
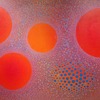
Jef Davis
29,162 PointsPhilippe,
I had the same exact code structure as you when I first ran into problems with passing this exercise. Christopher Earle's hint above put me on the right track:
You want to get rid of that second for loop you've created, because the getCategory() method only returns one variable. Instead of the for (String category : post.getCategory()) statement, you want to create a String variable named category and assign it the value of post.getCategory(). Doing this gives your method access to the information you need to then count the category using the same basic code you wrote above.
Christopher Earle
Courses Plus Student 22,340 PointsChristopher Earle
Courses Plus Student 22,340 PointsHi Phillippe,
Check the list of imported interfaces in Blog.java. Have you implemented the HashMap interface?