Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial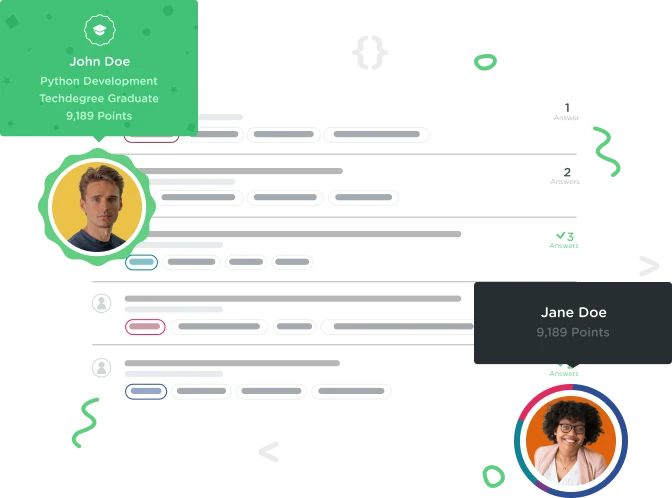

Darwin Smith
11,080 PointsIssue: changing className of sibling/childNodes
I'm confused as to exactly how to get to the solution and can't debug in this editor very well. Any help would be appreciated. Thanks!
const list = document.getElementsByTagName('ul')[0]; const li = list.childNodes;
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { for (let i = 0; i < li.length; i++) { li[i].firstChild.className = 'highlight'; } } });
const list = document.getElementsByTagName('ul')[0];
const li = list.childNodes;
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
for (let i = 0; i < li.length; i++) {
li[i].firstChild.className = 'highlight';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li>
<p>Element Selection</p>
<button>Highlight</button>
</li>
<li>
<p>Events</p>
<button>Highlight</button>
</li>
<li>
<p>Event Listening</p>
<button>Highlight</button>
</li>
<li>
<p>DOM Traversal</p>
<button>Highlight</button>
</li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>

Darwin Smith
11,080 PointsI think I'm supposed to use the provided 'list' const but unsure how to traverse and access only the p elements which precede the button elements
2 Answers

Jackson Monk
4,527 PointsI see the problem. You don't need a for loop or an li variable at all. You can complete this in one line, like this:
e.target.previousSibling.class Name = 'highlight';
This code says take the element the user clicked, go to its previous sibling, and change its class name to highlight

Darwin Smith
11,080 PointsThat makes sense, didn't realize it was so simple. Thank you very much.

Jackson Monk
4,527 PointsYou're welcome, happy I could help

Darwin Smith
11,080 PointsThese are the instructions:
A delegated click event listener has been attached to the selected ul element, which is stored in the variable list. The handler is targeting each button in the list. When any one of the buttons is clicked, a class of highlight should be added to the paragraph element immediately preceding that button inside the parent list item element. Add the code to create this behavior on line 5.
const list = document.getElementsByTagName('ul')[0];
const li = document.getElementsByTagName('li');
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
for (let i = 0; i < li.length; i++) {
li[i].firstChild.className = 'highlight';
}
}
});
Jackson Monk
4,527 PointsJackson Monk
4,527 PointsCould you elaborate a little more on your issue? I'm not sure exactly what you're having trouble with, but from my perspective it looks like your loop is never running because the e.target is referring to the entire ul, not the button elements inside it. Again, I'm not sure, so could you please tell me a bit more? I'd love to help.