Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial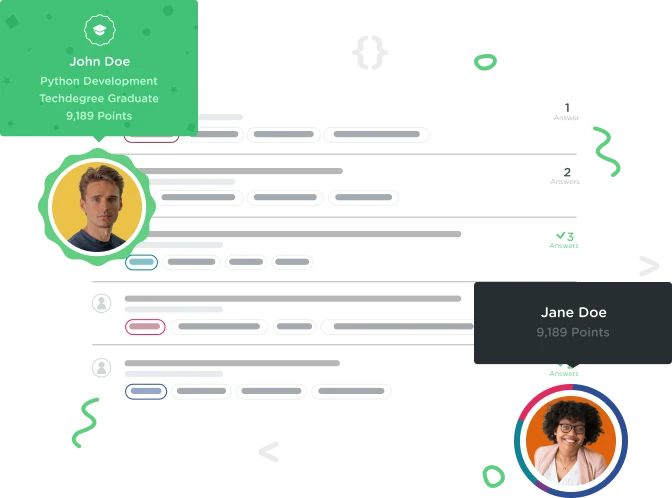

Unsubscribed User
Full Stack JavaScript Techdegree Student 1,198 PointsIs my formula correct to 2 random numbers between 2 values? when I output the result it seems everything is working fine
/**
* Returns a random number between two numbers.
*
* @param {number} lower - The lowest number value.
* @param {number} upper - The highest number value.
* @return {number} The random number value.
*/
const randomNumber = (upper, lower) => Math.floor(Math.random() * (upper -lower)) + lower;
// Call the function and pass it different values
console.log(randomNumber(50,34));
2 Answers
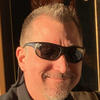
Peter Vann
36,429 PointsHi Ismael!
This will give you a random number between (and including) two numbers:
const randNumBtwn = (min, max) => Math.floor(Math.random() * (max - min + 1)) + min;
Your version does not ever include the upper/max value.
I hope that helps.
Stay safe and happy coding!
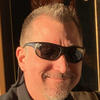
Peter Vann
36,429 PointsHi Ismael!
I thought about it some more and here is a way to generate a random number BETWEEN two numbers (excluding both those numbers). It is possible there is a cleaner way to do it, but this at least works:
const getRandomNum = (min, max) => {
let randNum = Math.floor(Math.random() * (max - min)) + min; // max is excluded already (got rid of + 1)
if (randNum == min) { // Exclude min
randNum = getRandomNum(min, max) // Yes, it's using recursion!?!
}
return randNum;
}
If randNum == min, it generates a different number, thus excluding min, yet still producing a random number.
I hope that helps.
Stay safe and happy coding!
Unsubscribed User
Full Stack JavaScript Techdegree Student 1,198 PointsUnsubscribed User
Full Stack JavaScript Techdegree Student 1,198 Pointsgreat, thanks!