Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial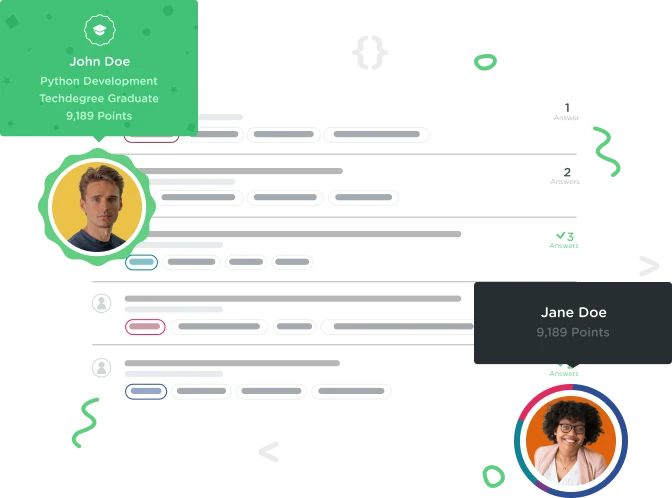
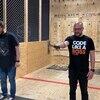
Shawn Currey
15,742 PointsInvoking functions with and without parenthesis
Finishing the last hour of the front-end development track and I feel I'm missing something.
Can someone better explain invoking functions with and without parenthesis? I want to say I understand it, and have been digging on StackOverflow for explanations (the best one for me so far https://stackoverflow.com/a/30751972/12956277) but I feel like I still don't quite get it in my head the way I feel I ought to and it seems oddly crucial in situations like when to invoke callbacks as parameters for something like an event's sake.
My question is more so, is there grand reasoning as to WHEN you should choose one over the other, especially in situations like callbacks where the call stack is semi-based on this I assume? For instance, if I use a function without parenthesis in a parameter, that parameter won't be put in UNTIL that function returns something? Otherwise, I guess the parameter never gets filled? And is this the only time it would be practical to invoke a function without parenthesis is "because" of waiting for Javascript to run synchronously through all of the steps?
Part of me feels like maybe I'm overthinking it so I wanted to ask the community. Thanks!
3 Answers
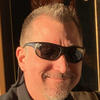
Peter Vann
36,427 PointsHi Shawn!
The difference is timing.
myFunction() immediately executes.
addEventlistener('click', myFunction) /*** Won't execute until the element is clicked ***/
This explains it better than I could:
here's an example that might help you see what's happening behind the scenes:
const square = function(num) {
return num * num; // returns the squared number
}
const quad = function(func, num) { // func is function being passed (not called) as a parameter
return func(num) * func(num); // here is where func is called and executed
}
alert( quad(square, 3) ); // square is the argument (a potentially executable function) that becomes the func parameter
Therefore square is passed into quad but won't execute until quad is called.
BTW, in this case, the alert will display 81.
I hope that helps.
Stay safe and happy coding!
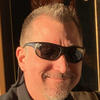
Peter Vann
36,427 PointsHi Shawn!
This takes the discussion to a whole (n)other level:
https://www.w3schools.com/js/js_function_closures.asp
(Read especially the full closure example near the bottom.)
This is interesting, too:
More info:
https://www.w3schools.com/js/js_function_definition.asp
I hope that helps.
Stay safe and happy coding!
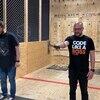
Shawn Currey
15,742 PointsWell dang Peter that more than helped! Thank you so much!
That's a lot of information. I'll be going thru this a few times I'm sure, thanks again!