Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial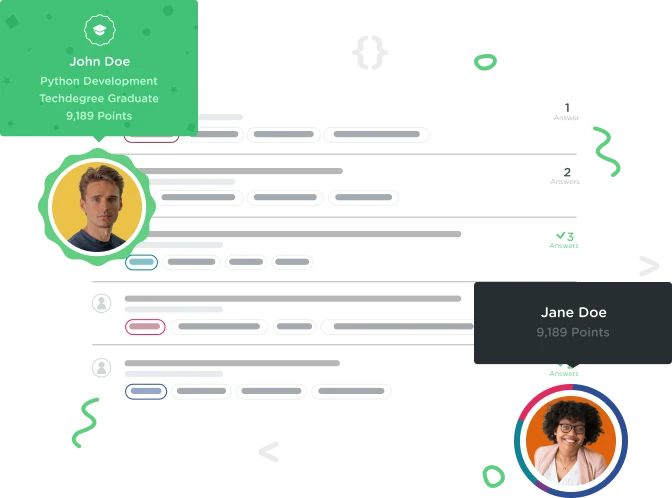

Abson Chifodya
11,817 PointsInside the major() setter method, set the student's major to a backing property "major". If the student's level is Junio
Inside the major() setter method, set the student's major to a backing property "major". If the student's level is Junior or Senior, the value of the backing property should be equal to the parameter passed to the setter method. If the student is only a Freshman or Sophomore, set the "major" backing property equal to 'None'
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
get major () {
return this._major;
}
set major(major){
this._major = major;
}
set none(none){
this._none = none;
}
}
var student = new Student(3.9, 60);
12 Answers
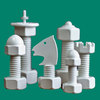
Steven Parker
231,269 PointsFor this challenge, you only need to create the setter method "major", you won't need to create a getter or any other setters.
Then, inside the setter, you'll need some conditional logic to store the correct value based on student's credits (or level).
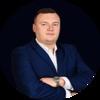
Patryk Holody
3,776 Pointsclass Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major) {
if ( this.level === 'Senior' || this.level === 'Junior') {
this._major = major;
return this._major;
} else {
this._major = 'none';
return this._major;
}
}
}
var student = new Student(3.9, 60);
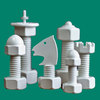
Steven Parker
231,269 Points Posting solutions without explanation is strongly discouraged by Treehouse and possibly subject to redaction by staff and moderators!
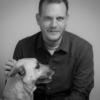
Tyler Dix
14,230 PointsThank you. I personally don't understand any of this, nor am I compelled to dig deeper because (more importantly), I don't understand *WHY I'm learning this. This isn't tied to any real-world examples, and I'm unclear how this in any way fits in to Front-End Web Development. I'm hoping that I'll look back at this comment and chuckle, but for now I'm completely lost.
A better explanation of the WHY question, more consistent quizes, more thorough explanations, and clear pre-reqs (why are we using back-ticks all of the sudden, along with a dollar sign? What did I miss here?).
I'm almost done with this track, and just need to slog through a few more videos to complete this course. In the meantime, thanks for just giving me the answer, because I have no understanding as to why I need to know this at this point.
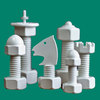
Steven Parker
231,269 PointsI don't see the "back-ticks...along with a dollar sign" that you mentioned here in these examples, but it sounds like you're talking about template literals and interpolation. There's a workshop specifically for that here called Introducing Template Literals and an associated Practice Template Literals to go with it.
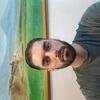
Vahe Gharagiozyan
10,088 PointsThanks Patryk

Jaroslav Bílek
9,415 PointsHorrible instructions, horrible task.
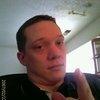
Timothy Sawyer
31,052 PointsThis worked for me:
set major(major) {
this._major = major
if (this.level !== 'Senior' && this.level !== 'Junior') {
this._major = 'None'
}
}
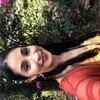
Jelena Feliciano
Full Stack JavaScript Techdegree Student 12,729 Pointsthis makes more sense

Godwin Onuegbu
16,460 PointsAlternatively you can keep the major method code shorter by using the credit property attribute, since both senior and junior have over 60 credits.
set major(major) { if ( this.credits > 60) { this._major = major; return this._major; } else { this._major = 'none'; return this._major; } }

Autour Love jr
10,230 PointsIm not saying this is stupid, but i do find it rather annoying that these quizzes keep including things we havent went over in the course. I know a lot of times in coding youre going to have to find the answer but man teach it to us first. At no point in the video did she use !==

L Haney
Front End Web Development Techdegree Student 10,263 PointsThis helped me: https://www.hongkiat.com/blog/getters-setters-javascript/
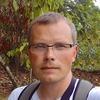
Ivan Kazakov
Courses Plus Student 43,317 Pointsunlike getters
, setters
shouldn't return
anything by the way.
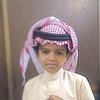
Ayman Omer
9,472 Points class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major) {
if ( this.level === 'Senior' || this.level === 'Junior') {
this._major = major;
return this._major;
} else {
this._major = 'none';
return this._major;
}
}
}
var student = new Student(3.9, 60);

Amir Khalil
4,565 PointsI'm wondering why this isn't working
set major(major){
this._major = 'None';
if (this.level !== 'Freshman') {
this._major = major;
}
}
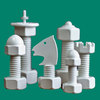
Steven Parker
231,269 PointsThink about what this does for a Sophomore, and compare that to the instructions.
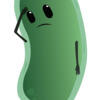
Garrett Ebersole
7,036 Pointsnot a big fan of some of these javascript lessons. i feel like some of the information is never covered in the videos

Vojciech Sobolevski
9,348 PointsI would highly encourage doing courses as part of the "track" rather than doing courses separately. Very often you need to know HTML and CSS to write decent JavaScript. If you do it as a part of a track, very often you will be taught things in other courses that are part of the same track but are not part of the JavaScript course.

kirkls
7,955 PointsI agree 100%, Vojciech. I saw the 'back ticks' in the code and knew immediately that was a template literal. Guil uses them throughout his JavaScript courses in the Web Dev track.
At the same time, students who just want to learn OOJS should be given a hint about something that is introduced in the individual course that hasn't yet been covered.
J llama
12,631 PointsJ llama
12,631 Pointsyoure sick steven. Keep it up
J llama
12,631 PointsJ llama
12,631 Pointswow steven thanks for the great help man you are just fantastic aren't you !?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsAww, shucks.
But clearly not everyone likes my hints. Someone gave me a 
Brandon Olson
Full Stack JavaScript Techdegree Student 5,445 PointsBrandon Olson
Full Stack JavaScript Techdegree Student 5,445 PointsWhats the logic behind turning credits into levels?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsI think the assumption is the credit/level system is an existing policy of the institution that would use this program. The program just implements what they already do.