Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial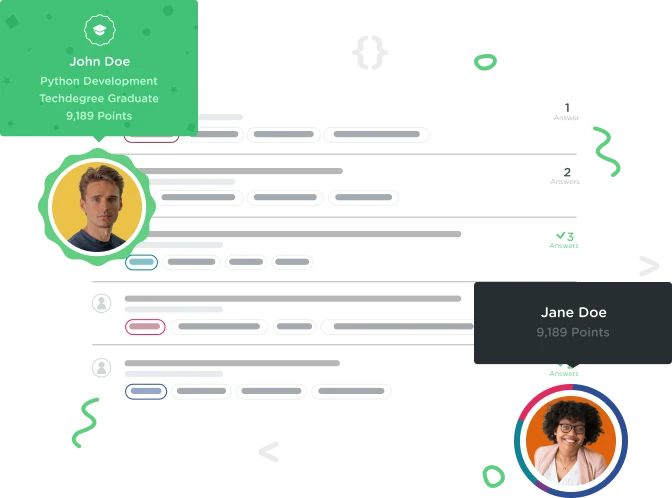
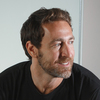
Daniel Kokin
1,496 PointsInitializing a variable to equal another variable
While experimenting with variables, I declared "subTotal" and initialed it to equal "total" but I'm get error: initializer element is not a compile-time constant. Is this a limitation of obj-c?
10 Answers
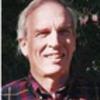
jcorum
71,830 PointsAh, ha! If you take a close look, you will see that in my code the two lines that are giving you a problem are inside the main method. In your code they are not. So you didn't just copy my code, because your's is quite different.
Delete everything, and I mean everything, and then paste this in:
#import <Foundation/Foundation.h>
NSString *employeeMessage;
void printTicket(){
employeeMessage = @"printed message";
NSLog(@"%@", employeeMessage);
}
int main(int argc, const char * argv[]) {
//Is the customer under 13 OR over 65 and over? If so, ageDiscount
//Is the movie a matinee?
//Is the customer an employee?
bool ageDiscount;
bool isEmployee = TRUE;
bool isMatinee = false;
//Full price tickets are $10
//Non employee with age discount AND matinee: $6.50
//Non employee without age discount OR matinee: $8.00
//Employee attending non-matinee: $4.50
//Employee attending matinee: $0.0 (FREE)
float regularPrice = 10.00;
float ageOrMatineePrice = 8.50;
float ageAndMatineePrice = 6.60;
float employeeRegPrice = 4.50;
float employeeMatineePrice = 0.0;
int customerAge;
float customerPrice;
int youthAge = 13;
int seniorAge = 65;
float subTotal = 0.0;
float total = subTotal;
float taxRate = .05;
NSArray *agesArray = @[@5,@5,@14,@42,@77];
ageDiscount = ((customerAge < 13) || (customerAge >=65)) ? YES : NO;
//if ((customerAge < 13) || (customerAge >=65)) {
// ageDiscount = YES;
//}
if (ageDiscount && isMatinee && !isEmployee) {
customerPrice = ageAndMatineePrice;
} else if ((ageDiscount || isMatinee) && !isEmployee) {
customerPrice = ageOrMatineePrice;
} else if (isEmployee && !isMatinee) {
customerPrice = employeeRegPrice;
employeeMessage = @"Thanks! Enjoy the movie.";
NSLog(@"%@", employeeMessage);
} else if (isEmployee && isMatinee) {
customerPrice = employeeMatineePrice;
employeeMessage = @"Thanks! Enjoy the FREE movie.";
NSLog(@"%@", employeeMessage);
} else {
customerPrice = regularPrice;
}
printTicket();
int mathTotal;
bool isComplete;
for(int i = 5; i <= 25; i++){
mathTotal++;
}
isComplete = TRUE;
NSLog(@"%i", mathTotal);
return 0;
}
Now all those declarations are inside the main method and everyone's happy. Why the difference. Here's an explanation from StackOverflow (http://stackoverflow.com/questions/6143107/compiler-error-initializer-element-is-not-a-compile-time-constant), and he says it much better than I could:
When you define a variable outside the scope of a function, that variable's value is actually written into your executable file. This means you can only use a constant value. Since you don't know everything about the runtime environment at compile time (which classes are available, what is their structure, etc.), you cannot create objective c objects until runtime, with the exception of constant strings, which are given a specific structure and guaranteed to stay that way.
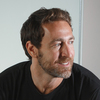
Daniel Kokin
1,496 PointsYou're right. I've been declaring my variables, bools, and array above the main function, which made them global. Everything was working until I tried initializing new variables equal other variables. I guess it also applies to arrays. Wow, that was a lesson in scope, huh?
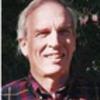
jcorum
71,830 PointsNeed to see your code. This works fine, so you must be doing something else.
float total = 10; //declare and initialize total
float subTotal = total; //declare subTotal and initialize it to total
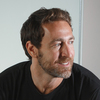
Daniel Kokin
1,496 Pointsfloat subTotal = 0.0;
float total = subTotal;
strange, right?
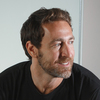
Daniel Kokin
1,496 PointsHmm, why doesn't my code get formatted like your code, too?
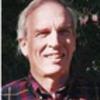
jcorum
71,830 PointsIf you mean "why doesn't it show up here with a black background, etc." it may be because you are not asking the server to treat it like code. To display code as code you enter a blank line and then a new line with 3 accents grave (the symbol under the tilde) and the word java. Then you paste in your code, starting on the next line. On the line after your code you do 3 more accents grave and another blank line.
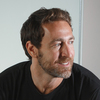
Daniel Kokin
1,496 PointsSomething is strange. I'm getting the same error when I try to declare a new array.
NSArray *agesArray = @[@5,@5,@14,@42,@77];
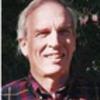
jcorum
71,830 PointsYour line of code works fine in my Xcode.
NSArray *agesArray = @[@5,@5,@14,@42,@77];
P.S., in my last comment I said java. I meant c (after the ``` you put the kind of code).

Reed Carson
8,306 Pointscheck to make sure you havent missed a semicolon anywhere, forgotten a bracket or something like that
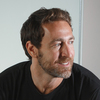
Daniel Kokin
1,496 Pointsfloat test = 0;
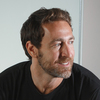
Daniel Kokin
1,496 PointsThank you!
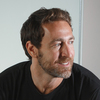
Daniel Kokin
1,496 Points#import <Foundation/Foundation.h>
NSString *employeeMessage;
//AND &&
//OR ||
//NOT !
//Is the customer under 13 OR over 65 and over? If so, ageDiscount
//Is the movie a matinee?
//Is the customer an employee?
bool ageDiscount;
bool isEmployee = TRUE;
bool isMatinee = false;
//Full price tickets are $10
//Non employee with age discount AND matinee: $6.50
//Non employee without age discount OR matinee: $8.00
//Employee attending non-matinee: $4.50
//Employee attending matinee: $0.0 (FREE)
float regularPrice = 10.00;
float ageOrMatineePrice = 8.50;
float ageAndMatineePrice = 6.60;
float employeeRegPrice = 4.50;
float employeeMatineePrice = 0.0;
int customerAge;
float customerPrice;
int youthAge = 13;
int seniorAge = 65;
float subTotal = 0.0;
float total = subTotal;
float taxRate = .05;
NSArray *agesArray = @[@5,@5,@14,@42,@77];
I'm getting the error on "total = subTotal" and "NSArray *agesArray"
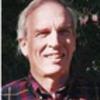
jcorum
71,830 PointsThis is really strange. I copy your code and paste it into Xcode and it's fine (although there are quite a few warning icons ("unused"), which are expected. Let's try this. Start a new project. Erase everything that Xcode puts in. Copy all the code below. Paste it in. See if you get any errors.
#import <Foundation/Foundation.h>
int main(int argc, const char * argv[]) {
@autoreleasepool {
// insert code here...
NSLog(@"Hello, World!");
}
bool ageDiscount;
bool isEmployee = TRUE;
bool isMatinee = false;
//Full price tickets are $10
//Non employee with age discount AND matinee: $6.50
//Non employee without age discount OR matinee: $8.00
//Employee attending non-matinee: $4.50
//Employee attending matinee: $0.0 (FREE)
float regularPrice = 10.00;
float ageOrMatineePrice = 8.50;
float ageAndMatineePrice = 6.60;
float employeeRegPrice = 4.50;
float employeeMatineePrice = 0.0;
int customerAge;
float customerPrice;
int youthAge = 13;
int seniorAge = 65;
float subTotal = 0.0;
float total = subTotal;
float taxRate = .05;
NSArray *agesArray = @[@5,@5,@14,@42,@77];
}
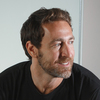
Daniel Kokin
1,496 PointsI tried copying it and it's giving me the same error! This is all the code:
#import <Foundation/Foundation.h>
//Is the customer under 13 OR over 65 and over? If so, ageDiscount
//Is the movie a matinee?
//Is the customer an employee?
bool ageDiscount;
bool isEmployee = TRUE;
bool isMatinee = false;
//Full price tickets are $10
//Non employee with age discount AND matinee: $6.50
//Non employee without age discount OR matinee: $8.00
//Employee attending non-matinee: $4.50
//Employee attending matinee: $0.0 (FREE)
NSString *employeeMessage;
float regularPrice = 10.00;
float ageOrMatineePrice = 8.50;
float ageAndMatineePrice = 6.60;
float employeeRegPrice = 4.50;
float employeeMatineePrice = 0.0;
int customerAge;
float customerPrice;
int youthAge = 13;
int seniorAge = 65;
float subTotal = 0.0;
float total = subTotal;
float taxRate = .05;
NSArray *agesArray = @[@5,@5,@14,@42,@77];
void printTicket(){
employeeMessage = @"printed message";
NSLog(@"%@", employeeMessage);
}
int main(int argc, const char * argv[]) {
ageDiscount = ((customerAge < 13) || (customerAge >=65)) ? YES : NO;
//if ((customerAge < 13) || (customerAge >=65)) {
// ageDiscount = YES;
//}
if (ageDiscount && isMatinee && !isEmployee) {
customerPrice = ageAndMatineePrice;
} else if ((ageDiscount || isMatinee) && !isEmployee) {
customerPrice = ageOrMatineePrice;
} else if (isEmployee && !isMatinee) {
customerPrice = employeeRegPrice;
employeeMessage = @"Thanks! Enjoy the movie.";
NSLog(@"%@", employeeMessage);
} else if (isEmployee && isMatinee) {
customerPrice = employeeMatineePrice;
employeeMessage = @"Thanks! Enjoy the FREE movie.";
NSLog(@"%@", employeeMessage);
} else {
customerPrice = regularPrice;
}
printTicket();
int mathTotal;
bool isComplete;
for(int i = 5; i <= 25; i++){
mathTotal++;
}
isComplete = TRUE;
NSLog(@"%i", mathTotal);
return 0;
}
Daniel Kokin
1,496 PointsDaniel Kokin
1,496 PointsThat's where I found the same answer! Thank you, jcorum. Good times.