Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial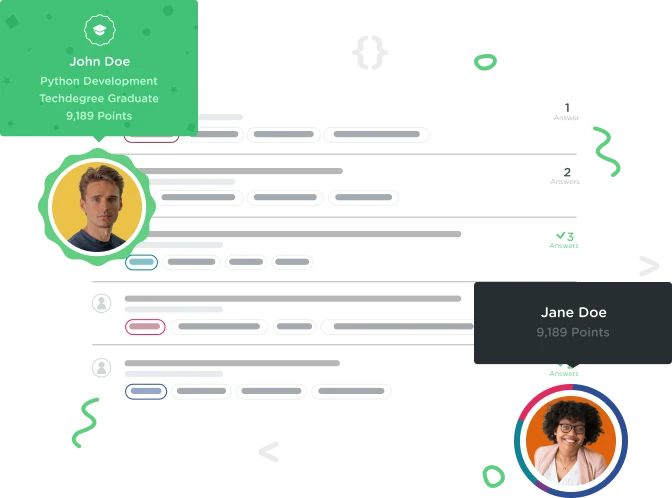

meitav asulin
1,123 Pointsinitializers
HI I've been stuck on it for a while: I can't understand what is override init and method, what is super init and what the connection between them can you give me explain about that subject?
1 Answer
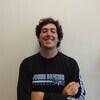
Charles Kenney
15,604 PointsHey meitav,
I'll try to break this down in order for you.
Methods are functions associated with a particular type. They handle the verbs of the objects they are defined in. For instance, a player object may have a move method, which provides the necessary functionality for the player to move. Don't over think it; it's a very simple concept. You can read more about methods here.
As we know, the init method is a special method that is called when an object is instantiated. We can use this to set up our object (like giving properties initial values or passing parameters). Here is an example
class Counter {
// init method
init(_ startingValue: Int) {
print("new instance created!")
self.count = startingValue
}
var count: Int
// starts at 0
// method that increases count by 1
func increment() {
self.count += 1
}
// method that decreases count by 1
func decrement() {
self.count -= 1
}
}
If we were to subclass this counter, we would inherit all of its methods and stored property. But what if we want to change how the methods and properties work? We would do this using the override keyword.
To override properties/methods of the parent class in a subclass declaration, prefix the properties/methods with override. For instance if I wanted to change the functionality of the increment method to make it decrement I would do the following,
class SillyCounter: Counter {
override func increment() {
self.count -= 1
}
}
Now if I were to make an instance of silly counter and call the increment method, it would decrement, unlike the parent class.
But what if we wanted to extend functionality without completely overriding a parent method/property. We could do this with the super method. The super keyword represents the parent class. So when you see super.foo, you know it is referring to the parent class's implementation of the foo property/method.
In this case, we will extend the functionality of our initializer by making it print "Hello, World".
class AdvancedCounter: Counter {
override init(_ startingValue: Int) {
// call the parents init method
// pass parameters down to the init
super.init(startingValue)
// extend the functionality
print("Hello, World!")
}
}
Basically, I defined a new init method that took the same parameters as the parent method. Then, I called the parents init method with the startingValue parameter supplied by the current init method. From there, the parent init method is able to initialize the object just as it would in the parent class. Finally, I was able to extend the functionality of the init method by adding more code after the super.init method.
This can be a rather confusing concept when first introduced to the object oriented paradigm. I encourage you to consult the docs when you are confused. They've helped me a lot.
Here is the inheritance page of the docs.
Hope this helps
-Charles