Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial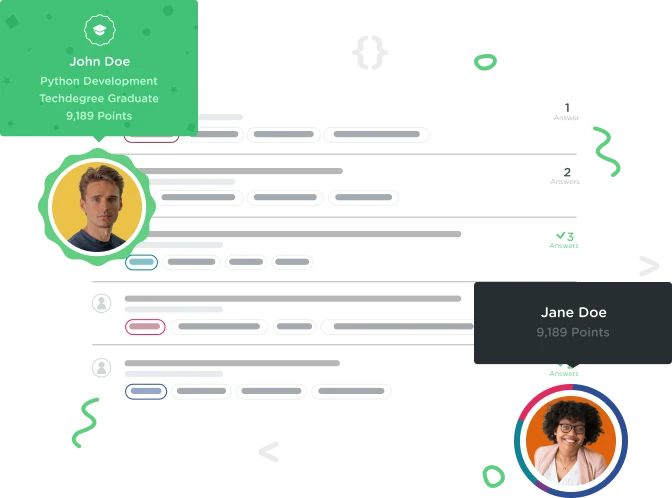

wngcarpenter
2,987 Pointsindex.html doesn't run the code.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Practice JavaScript Math</title> <link href="css/styles.css" rel="stylesheet"> </head> <body> <script src="js/math.js"></script> </body> </html>
This code above does not run this. // 1. Attach this file -- math.js -- to the index.html file using a <script> tag
// 2. Add an alert to announce the program with a message like "Let's do some math!"
alert("Let's do some math!"); // 3. Create a variable and use the prompt() method to collect a number from a visitor
var aNumb = prompt("Give me a number."); // 4. Convert that value from a string to a floating point number
var theNumb = parseInt(aNumb); // 5. Repeat steps 3 and 4 to create a second variable and collect a second number
var secNumb = prompt("Give me a second number."); var secondNumb = parseInt(secNumb); // 6. Create a new variable -- message -- which you'll use to build // a complete message to print to the document // Start by creating a string that includes <h1> tags as well // and the two input numbers. The string should look something like this: // "<h1>Math with the numbers 3 and 4</h1>" where the two numbers are // the values input from the user. Use string concatenation to create this // and make sure you actually perform the math on the values by // using the + symbol to add their values together var message = "<h1>Math with the numbers " + theNumb " and " + secondNumb + "</h1>";
// 7. Add another string to the message variable. // The string should look something like this after concatenation: // "3 + 4 = 7"
// 8. Add a linebreak tag -- <br> -- to the message variable
// 9. Continue to add to the message variable to include strings // demonstrating multiplication, division and subtraction // For example: // "3 * 4 = 12" // "3 / 4 = 0.75" // "3 - 4 = -1"
// 10. Use the document.write() method to print the message variable // to the web page. Open the finished.png file in this workspace // to see what the completed output should look like document.write(message);
5 Answers
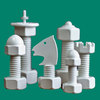
Steven Parker
231,275 PointsIt looks like you have a typo.
On line 24 of math.js it looks like there's a missing concatenation operator ("+
") between theNumb and the literal string following it.
Corrected version:
var message = "<h1>Math with the numbers " + theNumb + " and " + secondNumb + "</h1>";
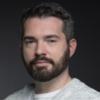
Cory Harkins
16,500 PointsMy only thoughts on this are to restart your workspace, I plugged your code in, and it's working fine for me.
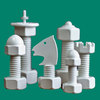
Steven Parker
231,275 PointsCheck the code file name and location.
The HTML is expecting to load the code from a file named math.js and from subfolder named js. Check the name of your code file, and that it is in that subfolder.
To enable a more complete and accurate analysis, make a snapshot of your workspace and post the link to it here.
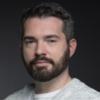
Cory Harkins
16,500 PointsI copied and pasted, and loaded, worked fine... I wonder if maybe he/she didn't save the HTML file? :-/

wngcarpenter
2,987 PointsGotta be something else. It doesn't pull up my alert message when I open the HTML file.
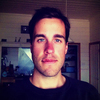
Sam Donald
36,305 PointsAnother way of doing things
This is not an answer for your work. I just thought I'd share another way of implementing the programme.