Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial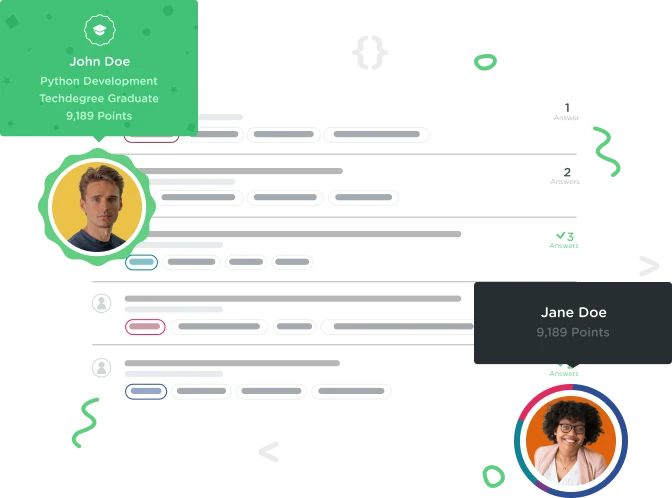

Rangarajan R
930 PointsIn the normalizeDiscountCode verify that only letters or the $ character are used. Am not able to get it right.
I have cross checked the code in Intellij.. It shows no errors. But here, it gives me an error. WHy?
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private int i = 0;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
for (i=0; i<discountCode.length(); i++){
char ch = discountCode.charAt(i);
if (! (Character.isLetter(ch)) || ch == '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
5 Answers

andren
28,558 PointsYour solution will throw an exception if the character is not a letter or it is the $ symbol. The problem is that the $ symbol is in fact a valid character so it should not throw an exception.
You can fix this issue by extending the scope of your !
operator like this:
if (!(Character.isLetter(ch) || ch == '$')){ // The ! now covers both conditions and the || operator
throw new IllegalArgumentException("Invalid discount code");
}
With that code if the character is a letter or the $ symbol the if statement will not run since the true that is produced will be turned into false by !
. And if the character is neither then the false that is returned will be converted to true and the if statement will run.
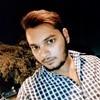
Bhawani Shankar
954 Pointspublic class Order { private String itemName; private int priceInCents; private String discountCode;
public Order(String itemName, int priceInCents) { this.itemName = itemName; this.priceInCents = priceInCents; }
public String getItemName() { return itemName; }
public int getPriceInCents() { return priceInCents; }
public String getDiscountCode() { return discountCode; }
public void applyDiscountCode(String discountCode) { this.discountCode = normalizeDiscountCode(discountCode); } private String normalizeDiscountCode(String discountCode){ if(!discountCode.matches"(A-Za-z$)+"); throw new IllegalArgumentException("Invalid discountCode"); return discountCode.toUpperCase(); } }

Rangarajan R
930 PointsThank you!!!
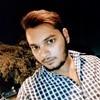
Bhawani Shankar
954 Points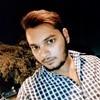
Bhawani Shankar
954 PointsPlease visit this link ThankYou
Bhawani Shankar
954 PointsBhawani Shankar
954 Pointsnot working
andren
28,558 Pointsandren
28,558 PointsBhawani Shankar: My solution was specifically tailored around the code Rangarajan R had already written, if the code you have written around the if statement differs then the conditions in my post might not work. Can you post all of the code you have written so far? That will give me a better idea of how to fix it in your case.