Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial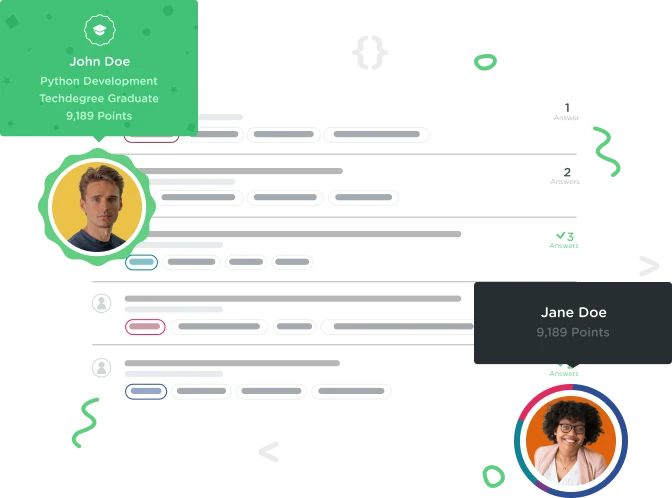

Akshay Chaudhary
Courses Plus Student 2,428 PointsIn the example given in the tutorial, is "task" a constant or a variable?
In the following code:
var todo: [String] = ["Finish Collections Course", "Buy groceries", "Respond to emails", "Order books online"]
for task in todo {
print(task)
}
Pasan explained the use of "task". But he said that it's a constant. If "task" is a constant, then how is it's value changing everytime the loop is running. Shouldn't it just print only the first item from array, and then returned an error?
4 Answers
Hannah Gaskins
14,572 Points"task" there in the for loop is a constant that represents the current value of the iterator. This constant only exists inside the body of the loop. And because it the value of "task" is automatically set at the beginning of each iteration of the loop it is a constant and not a variable. My understanding is that it is constant for each iteration over the range of the loop. Here is a link on the Apple Dev Guide that explains For-In Loops a bit more in depth:
Apple Developer Guide on Control Flow
Hope this helps a bit!
Cheers :)
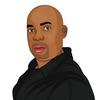
Quinton Gordon
10,985 PointsI think a super simple way to conceptualize it is to just think of that constant being destroyed and recreated for each item.

Martin Granger
14,521 PointsHi Akshay,
Each time this loop runs, it grabs the array's value for the iteration/index and sets that value to new constant called "task". So since "todo" has 4 values, this loop will be run 4 times. Essentially, every value inside of todo is set to its own separate constant. This loop is written in a shorthand fashion to remove unnecessary lines of code.
Take a look at this rewritten one:
for i in 0...todo.count - 1 {
let task = todo[i]
//This time the constant "i" represents the index value for todo. In this case, it'll be numbers, 0,1,2,3
print(task)
/*
This print statement will print the string value of the todo array at the given index
If you only wanted to print the first value, then you would do something like
print(todo[0])
*/
}
I really hope this helps. Let me know if it doesn't. Also, try to play around with this code snippet in a Playground. That has been a huge help for me when I get stuck.

Akshay Chaudhary
Courses Plus Student 2,428 PointsThanks Martin. You provided a very good explanation there.
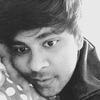
SivaKumar Kataru
2,386 PointsAs far as My understanding............
// Creating an array
let someIntegers: [UInt] = [1,2,3,4,5,6,9,7]
for task in someIntegers {
print(task)
}
/*
-------------------------------------------------------------------------------------
** Internally the For in Loop syntax will look like this, remember this is sort of hidden
from user perspective: **
-------------------------------------------------------------------------------------
for i in 0 ..< someIntegers.count {
// REMEMBER,Every Time The for in loop is done printing each statement,
// it will create new constant named (task) everytime
// and removes the old constant.
let task = someIntegers[i]
print(task)
}
// So swift simply generate a new syntax with exisiting syntax below:
for task in someIntegers {
print(task)
}
*/