Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial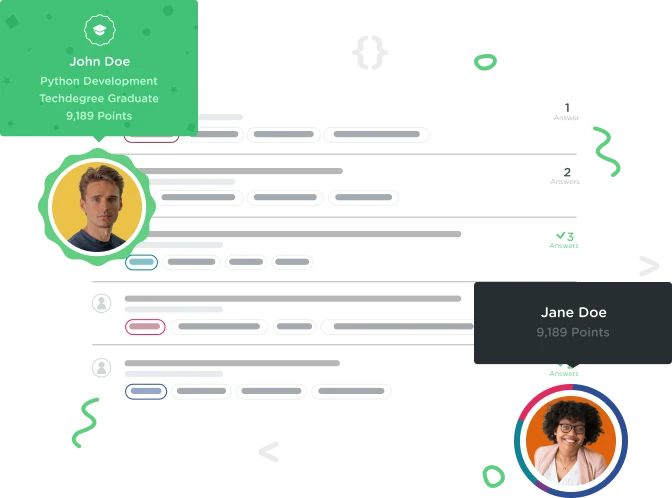

Addison Francisco
9,561 PointsImage literal name doesn't display next to image literal
I've noticed that the name of the image literal is not present after selecting the image. This is inconsistent with Pasan's code but still works fine. It's the same with color literals, as well. Was this a change between Xcode 8 and 9? I'm currently using Xcode 9.

Jason Gallant
8,437 PointsI am having the same issue. When I autocomplete .returnTrip: return ReturnImage, the "ReturnImage" image is replaced with a very small version of the image, without any code after it, which looks very different than the code above. I've seen this before in the vending machine app as I recall. Is this expected behavior, or do I have a setting I need to change? I can't find anything online, nor in the documentation about this particular strangeness. I am on Xcode 9.2 in Swift 4.1
thanks,
Jason.

Alex Busu
Courses Plus Student 11,819 PointsWhat's even more annoying is when I declare the enum cases because the name is one capitalized letter different between the case and the image file it's constantly trying to add the image file to the enum which is soo annoying.

Amer Khalid
11,533 PointsSame here, went through all setting in xcode, cannot get it to show.

Josh Gonet
5,602 PointsNot sure if it's due to Swift 5 being the latest version that works with this, but the below worked for me -
extension Story {
var artwork: UIImage {
switch self {
case .returnTrip:
return UIImage(imageLiteralResourceName: "ReturnTrip")
case .touchDown:
return UIImage(imageLiteralResourceName: "TouchDown")
case .homeward:
return UIImage(imageLiteralResourceName: "Homeward")
case .rover:
return UIImage(imageLiteralResourceName: "Rover")
case .cave:
return UIImage(imageLiteralResourceName: "Cave")
case .crate:
return UIImage(imageLiteralResourceName: "Crate")
case .monster:
return UIImage(imageLiteralResourceName: "Monster")
case .droid:
return UIImage(imageLiteralResourceName: "Droid")
case .home:
return UIImage(imageLiteralResourceName: "Home")
}
}
}
The imageLiteralResourceName keyword was needed each time.
4 Answers
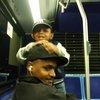
Xavier D
Courses Plus Student 5,840 PointsAs an alternative to the options already provided here, you can just add a single line comment at the end of each case to describe the name of each image literal, since Xcode appears to no longer provide the names of image literals...
extension Story
{
var artwork: UIImage
{
switch self
{
case .returnTrip: return #imageLiteral(resourceName: "ReturnTrip") // returns an image literal named ReturnTrip
case .touchDown: return #imageLiteral(resourceName: "TouchDown") // returns an image literal named TouchDown
case .homeward: return #imageLiteral(resourceName: "Homeward") // "" "" "" named Homeward
case .rover: return #imageLiteral(resourceName: "Rover") // "" "" "" named Rover
case .cave: return #imageLiteral(resourceName: "Cave") // "" "" "" "" Cave
case .crate: return #imageLiteral(resourceName: "Crate") // "" "" "" "" Crate
case .monster: return #imageLiteral(resourceName: "Monster") // "" "" "" "" Monster
case .droid: return #imageLiteral(resourceName: "Droid") // "" "" "" "" Droid
case .home: return #imageLiteral(resourceName: "Home") // "" "" "" "" named Home
}
}
}
FYI, if you cut and paste a line of code from Xcode into your post like how I did above, you'll notice that the image literal is substituted out and replaced with:
#imageLiteral(resourceName: "ReturnTrip")
And, if your tried to enter the substitution text into Xcode, it will be replaced by the corresponding image literal without its name, thus I felt it's best to use a single-line comment if you need to describe the name of the image literal for that case.
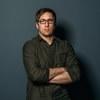
Jesse Gay
Full Stack JavaScript Techdegree Student 14,045 PointsIt seems autocomplete for image literals was removed from Xcode in Xcode 10 and Swift 4.2. https://stackoverflow.com/questions/51397347/xcode-10-image-literals-no-longer-available
Even when trying to type in #imageLiteral(resourceName: "ReturnTrip") I'm getting some strange autocomplete behavior in which as soon as I start typing re (for resource...), it deletes #imageLiteral(r (i.e. what's left is esourceName: "ReturnTrip")) There's some other strange autocomplete behavior as well. I'm on Version 10.1 (10B61)
If you comment out your code, you can type it in properly (without Autocomplete messing with it), then uncomment when you're done.

Justin Comstock
122 PointsSame problem here.

Lisa Vega
5,736 PointsI was bothered by this so I rewrote this section of code as follows: enum Story: String { case ReturnTrip case TouchDown case Homeward case Rover case Cave case Crate case Monster case Droid case Home }
extension Story { var artwork: UIImage { guard let artwork = UIImage(named: self.rawValue) else { fatalError() } return artwork } }
Thomas Swatland
13,530 PointsThomas Swatland
13,530 PointsSame here, probably part of the Swift 4 update.