Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial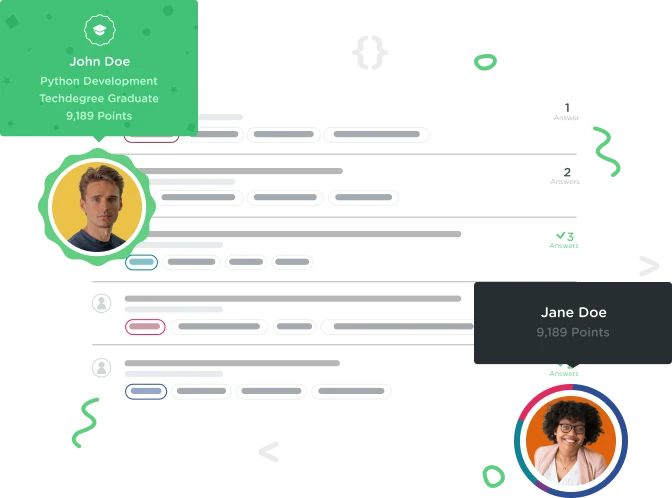
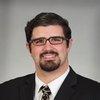
Aaron Goodman
3,953 PointsI'm unsure of how to add to the course list. mVideos is private to Course, and in Course.java, there are only getters.
Unless I change mVideos to protected, I don't know how I'm going to change it from another class. But that is outside the scope of the instructions.
Please help!!! -Aaron
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Course mCourse;
mCourse = course;
Video newVideo = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
mVideos.add(1,newVideo);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
return null;
}
}
4 Answers

Gavin Ralston
28,770 PointsFrom Course.java
public List<Video> getVideos() {
return mVideos;
}
While mVideos is private, Course.getVideos() returns a reference to the list, so you can modify that just fine by chaining method calls.
course.getVideos().doSomethingToTheList();
Hope that helps!
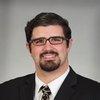
Aaron Goodman
3,953 PointsYou guys are amazing. Thank you so much. I'm almost done with stage 3 now. The treehouse community is awesome.
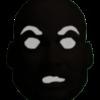
Geovanie Alvarez
21,500 Pointspublic void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video video = new Video("The Beginning Bits"); // <------ create the video
// TODO(2): Add the newly created video to the course videos as the second video.
course.getVideos().add(1, video); // <------ add the video
}
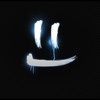
Grigorij Schleifer
10,365 PointsHi Aaron,
let me comment the challenge. Try to solve it by your own and if you are stuck read the comments:
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.TreeMap;
public class QuickFix {
public void addForgottenVideo(Course course) {
Video vid = new Video("The Beginning Bits");
// creating a new instance of the Video class
// thet takes a String parameter with the title of the Video
course.getVideos().add(1, vid);
// getVideos() returns a List with Videos
// so we can add our newly created Video "vid" on the second place in the List
// into our Course named "course"
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> map = videosByTitle(course);
// creating a Map and using the videoByTitle method to generate a Map
for (Video vid: course.getVideos()) {
// „for every video in all videos from the course“
if (vid.getTitle().equals(oldTitle)) {
// vid.getTitle() gets you the title from every video
// equals(oldTitle) and compares it with the old title
// so if the condition is true (vid.getTitle() gives you a title that is equal to oldTitle
vid.setTitle(newTitle);
// set a new title
// baaaaaaaaam !!!
}
}
}
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> byTitle = new TreeMap<String, Video>();
// create am Map and declare it as TreeMap
// that will give you a natural ordered collection of Titles and Videos
// Title as key and the Video as value
for(Video video: course.getVideos()) {
// you need a for loop that goes over every video inside the course
// to do that you will need to use the getVideos() method
// that gives you a List of videos from the Course "course"
// you can read the line as „ for every video in all videos from the course“
byTitle.put(video.getTitle(), video);
// here comes our new created Map
// we can add the title and the video using the "put"
// "put" method is included into the Map interface to add keys and values
// to get the title we use getTitle() method from our Video class
}
return byTitle;
}
}
Let me know if something is not clear...
Grigorij
Shadd Anderson
Treehouse Project ReviewerShadd Anderson
Treehouse Project ReviewerHa.... I just made mVideos public. Guess I should have probably done it this way though. :S