Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial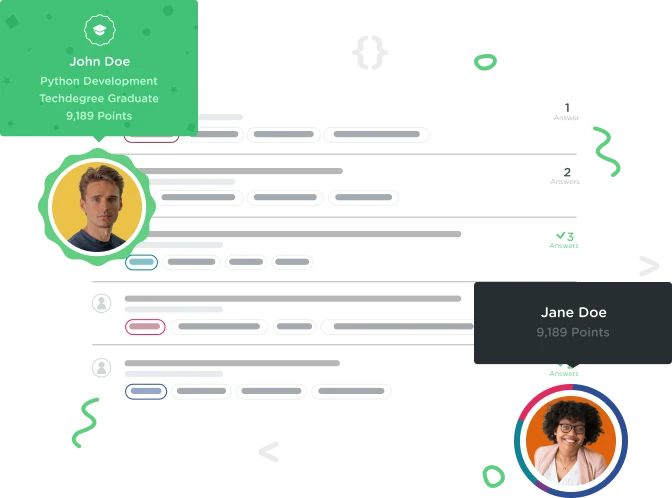

N Elliott
2,628 PointsI'm stuck on this last question. help
Not sure what I'm doing wrong
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum(String topic) {
this.forum = forum(topic);
}
public String getTopic() {
return topic;
}
//Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
// TODO: add getters for firstName and lastName
public String getFirstName() {
return this.firstName;
}
public String getLastName() {
return this.lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public ForumPost(User author, String title, String description) {
this.author = author;
this.title = title;
this.description = description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
// Uncomment this when prompted
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User("John","Smith");
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost("John Smith", "A title", "A description");
forum.addPost("this is a test post");
//
}
}
4 Answers

andren
28,558 PointsThere are a number of issues with your code:
In the
Forum
class constructor you have this linethis.forum = forum(topic);
I'm not quite sure what the logic behind it is but it is incorrect. The constructor is meant to set the field variable called topic (this.topic
) equal to the parameter called topic (topic
) this is done simply like thisthis.topic = topic
. You got the constructor correct for theForumPost
class so I'm not sure why you did it differently for this constructor.The task does not want you to supply the name of the
User
you create. It wants you to get the first and last name through arguments passed to the program. In Java you access program arguments though an array calledargs
. Soargs[0]
would be used to fetch the first argument andargs[1]
would be used to fetch the second one. The task wants these arguments to be used to supply the first and last name to theUser
object you create.The
ForumPost
class requires aUser
object as it first argument, but you pass it a string (John Smith
). You are meant to pass theUser
you created above and stored in the author variable instead.You make a similar mistake when it comes to calling the
addPost
method, it takes aForumPost
object not a string. Again you are meant to pass the object you created in the above line of code.
If you fix those mistakes your code should pass.

N Elliott
2,628 PointsThanks! It worked. However, I don't think Craig Dennnis covered arrays in detail in this class. The only thing he covered was .toarray This was confusing.

N Elliott
2,628 Pointswait, now it doesn't work.

andren
28,558 PointsCan you post the code? If you followed all of my instructions to the letter then the code should work, as I basically listed out all of the things I changed in your code in order to get it to pass the challenge.
If you want to look at the solution in order to compare it to your code I have posted it here. It only includes the solution code for the Main.java file. But your code in the other files were already correct with the exception of the Forum constructor which I already addressed in my first post.

N Elliott
2,628 PointsIt works as you described. Thank you Andren.