Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial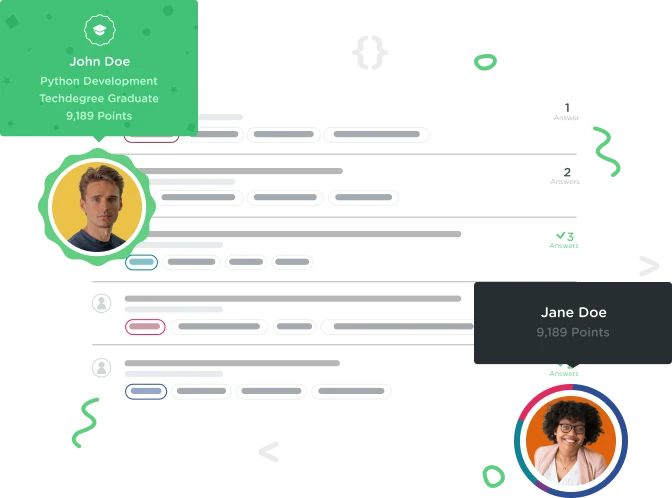

Anthony Reymond
329 PointsI'm stuck in task 2 in swift basics "operators"
task 2
I just don't know what to do, the question isn't clear?
result == someOperation result =< anotherOperation isPerfectMultiple == someOperation
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = 200 % 5
// Task 2 - Enter your code below
result == someOperation
result =< anotherOperation
isPerfectMultiple == someOperation
1 Answer

Addison Francisco
9,561 PointsHi, Anthony. Let's see if we can clarify the questions asked for this challenge.
Step 1: Using the remainder operator, compute the remainder given the value and a divisor. Assign this value to a constant named result.
So, as you have learned in the Arithmetic Operators video, we can use a modulo operator (%
) to get the remainder of a number and divisor. For this challenge, they have asked you to get the remainder of the declared variables value
and divisor
, then assign the remainder of this operation to a constant variable named result
. I'll break this into 2 steps so it's a little more clear what is happening. First, we want to get the remainder of value
and divisor
.
// Calculate the remainder by using a modulo
value % divisor
This is the same as 200 % 5
, which returns 0
because 200 / 5
has no remainder.
Second, we want to assign the remainder to a constant variable named result
. We can do this by modifying the above...
// Calculate the remainder by using a modulo AND assign the remainder of value and divisor to a constant var named result
let result = value % divisor
Now we have a variable named result
that holds the remaining value of value % divisor
. We can now address Step 2.
Step 2: When value obtained using a remainder operator is 0, this means that the value is a perfect multiple of the divisor. Compare the value of
result
to0
using the equality operator and assign the resulting value to a constant namedisPerfectMultiple
.
First, as they have asked, let's compare result
to 0
using the equality operator.
// Is result the same value as 0? We can find out by using the equality operator
result == 0
result
's value is 0. So, comparing (==
) result
against 0
will return true
.
Second, we want to assign the returned value of this comparison to a constant variable named 'isPerfectMatch'. Here's how...
let isPerfectMultiple = result == 0
What this is doing is first comparing result
with 0
, which equals true
because result
's value is also 0
. Then it is taking that true
value and assigning it to isPerfectMultiple
. So, now we have a var named isPerfectMultiple
that equals true
, just as they asked.
Our code should now look like this...
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = value % divisor
// Task 2 - Enter your code below
let isPerfectMultiple = result == 0
Hope this was helpful for you, Anthony. Keep coding!
clarkbridgewater
5,519 Pointsclarkbridgewater
5,519 PointsHey there so the question I believe you are stuck on is:
Step 2: When value obtained using a remainder operator is 0, this means that the value is a perfect multiple of the divisor. Compare the value of result to 0 using the equality operator and assign the resulting value to a constant named isPerfectMultiple.
So in confusing questions like this I like to break it down in pieces.
a. Constant: isPerfectMultiple.
let isPerfectMultiple
and the value of RESULT is 0
result == 0.
let isPerfectMultiple = result == 0
Hope this has helped