Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial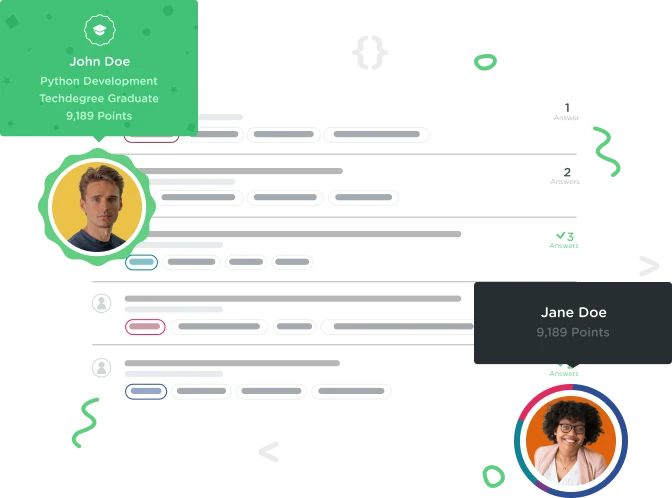

Shawn Black
2,164 PointsI'm pretty sure I know how to traverse the node, but I don't recall how to add a class
I'm assuming the CSS has a class of "highlight" available but using the previousLi.className = "h1" doesn't result in a change to the <li> text. I can successfully use previousLi.style.backgroundColor = 'yellow', but that's not what the directions state.
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let li = event.target.parentNode;
let previousLi = li.previousElementSibling;
if (previousLi){
previousLi.className = "highlight";
// previousLi.style.backgroundColor = 'yellow';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
3 Answers

KRIS NIKOLAISEN
54,970 PointsSometimes it helps to move the code to a workspace so you can at least use the console. There you could add log statements to see:
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let li = event.target.parentNode;
console.log(li)
let previousLi = li.previousElementSibling;
console.log(previousLi)
if (previousLi){
previousLi.className = "highlight";
// previousLi.style.backgroundColor = 'yellow';
}
}
});
In the above li
references the list item. previousLi
references the previous list item relative to li
. However the instructions are to add a class of highlight to a <p> element that's an immediate previous sibling of the button being clicked. If your code is correct the paragraph text to the left of the button will highlight when the button is clicked (in the challenge preview).
Since e.target refers to the button, the immediate previous sibling is simply e.target.previousElementSibling.
Your syntax for setting the class will work.
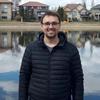
Dmitry Polyakov
4,989 PointsYou don't even need to grab on the li element here. You click on a button and add a class of highlight to its previuosElementSibling, which is a paragraph - <p></p>

Shawn Black
2,164 PointsLike I just told Kris, so much of the lesson in the "JavaScript and the DOM" had us traversing to the parent element. It just didn't register that this question was working within the li element. I honestly thought I needed to highlight the <p> of the previous <li>. Thanks for your help.
Shawn Black
2,164 PointsShawn Black
2,164 PointsSo much of the lesson in the "JavaScript and the DOM" had us traversing to the parent element. It just didn't register that this question was working within the li element. I honestly thought I needed to highlight the <p> of the previous <li>. Thanks for your help.